How to Convert Int to Char Array in C++
-
Use
std::sprintf
Function to Convertint
to Char Array in C++ -
Combine
to_string()
andc_str()
Methods to Convertint
to Char Array in C++ -
Use
std::stringstream
Class Methods to Convertint
to Char Array in C++ -
Use
std::to_chars
Function to Convertint
to Char Array in C++
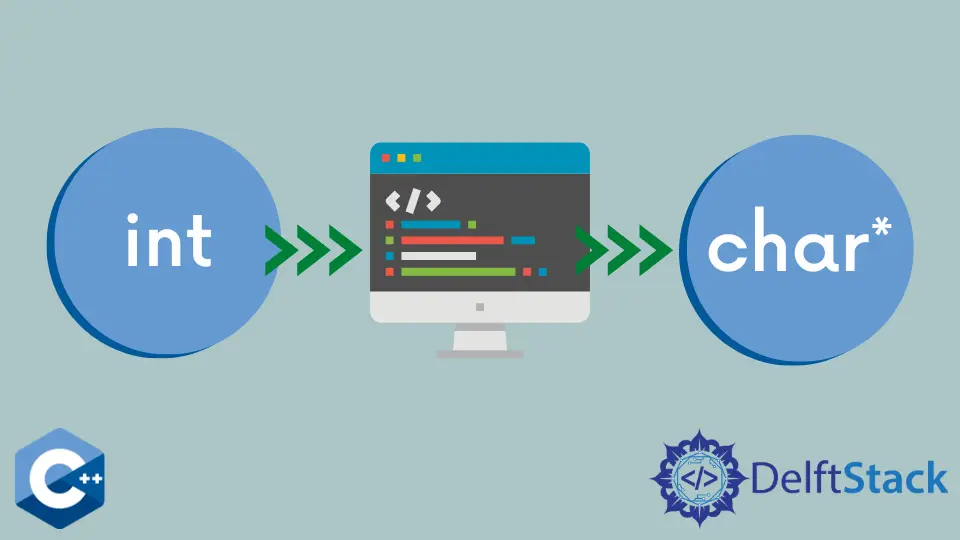
This article will explain how to convert int
to a char array (char*
) using different methods.
In the following examples, we assume to store the output of conversion in-memory buffer, and for verification purposes, we’re going to output result with std::printf
.
Use std::sprintf
Function to Convert int
to Char Array in C++
First, we need to allocate space to store a single int
variable that we’re going to convert in a char
buffer. Note that the following example is defining the maximum length MAX_DIGITS
for integer data. To calculate the char buffer length, we add sizeof(char)
because the sprintf
function writes char string terminating \0
byte automatically at the destination. Thus we should mind allocating enough space for this buffer.
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::sprintf(num_char, "%d", number);
std::printf("num_char: %s \n", num_char);
return 0;
}
Output:
num_char: 1234
Note that calling sprintf
with overlapping source and destination buffers (e.g. sprintf(buf, "%s some text to add", buf)
) is not recommended as it has undefined behavior and will produce incorrect results depending on the compiler.
Combine to_string()
and c_str()
Methods to Convert int
to Char Array in C++
This version utilizes std::string
class methods to do the conversion, making it a far safer version than dealing with sprintf
as shown in the previous example.
#include <iostream>
int main() {
int number = 1234;
std::string tmp = std::to_string(number);
char const *num_char = tmp.c_str();
printf("num_char: %s \n", num_char);
return 0;
}
Use std::stringstream
Class Methods to Convert int
to Char Array in C++
This method is implemented using[the std::stringstream
class. Namely, we create a temporary string-based stream where store int
data, then return string object by the str
method and finally call c_str
to do the conversion.
#include <iostream>
#include <sstream>
int main() {
int number = 1234;
std::stringstream tmp;
tmp << number;
char const *num_char = tmp.str().c_str();
printf("num_char: %s \n", num_char);
;
return 0;
}
Use std::to_chars
Function to Convert int
to Char Array in C++
This version is a pure C++ style function added in C++17 and defined in header <charconv>
. On the plus side, this method offers operations on ranges, which might be the most flexible solution in specific scenarios.
#include <charconv>
#include <iostream>
#define MAX_DIGITS 10
int main() {
int number = 1234;
char num_char[MAX_DIGITS + sizeof(char)];
std::to_chars(num_char, num_char + MAX_DIGITS, number);
std::printf("num_char: %s \n", num_char);
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Integer
- Use of 128-Bit Integer in C++
- Big Integer in C++
- How to Specify 64-Bit Integer in C++
- Comparison Between Signed and Unsigned Integer Expressions in C++
- INT_MAX and INT_MIN Macro Expressions in C++
- How to Check if a Number Is Prime in C++