How to Concatenate Two Strings in C++
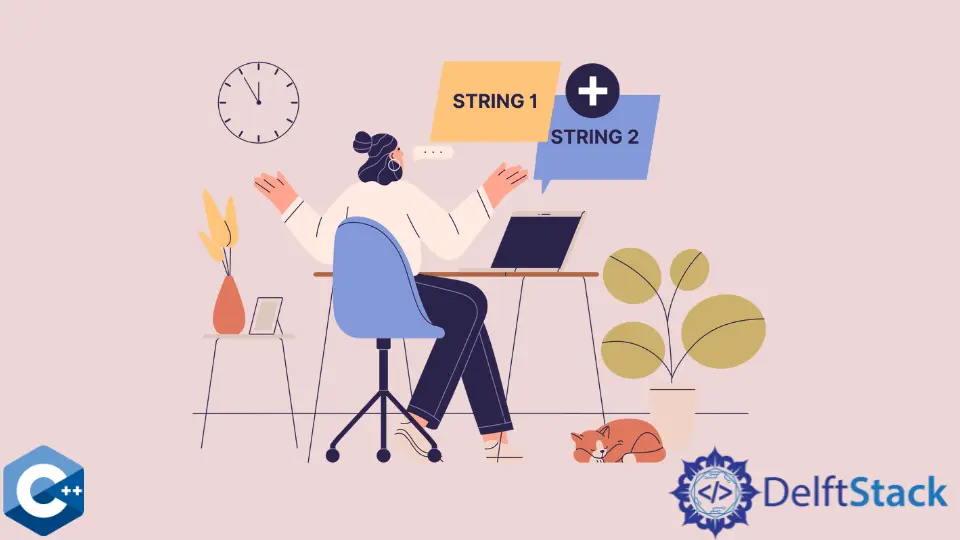
Concatenating strings is a fundamental task in programming, and C++ offers several ways to achieve this. Whether you’re working on a simple project or a complex application, knowing how to combine strings efficiently is essential.
In this article, we will explore different methods to concatenate two strings in C++. We’ll cover the use of the +
operator, the append()
method, and the sprintf()
function. Each method has its own advantages, so understanding them will help you choose the right one for your needs. Let’s dive into the world of string manipulation in C++!
Using the +
Operator
One of the simplest and most intuitive ways to concatenate two strings in C++ is by using the +
operator. This method is straightforward and allows you to combine strings seamlessly. Here’s how you can do it:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2;
std::cout << result << std::endl;
return 0;
}
Output:
Hello, World!
In this example, we first include the necessary headers and define the main
function. We create two string variables, str1
and str2
, and then concatenate them using the +
operator. The result is stored in a new string variable called result
. Finally, we print the concatenated string to the console.
This method is particularly useful for quick concatenation tasks. It’s easy to read and understand, making your code more maintainable. However, if you are concatenating multiple strings in a loop or dealing with large strings, performance might become a concern. In such cases, alternative methods may be more efficient.
Alternatively, we can construct a custom function that takes two string
variables as parameters and returns the result of concatenation. Note that string
has a move constructor, so returning long strings by value is quite efficient. The concTwoStrings
function constructs a new string
object, which is assigned to the string2
variable.
#include <iostream>
#include <string>
using std::copy;
using std::cout;
using std::endl;
using std::string;
string concTwoStrings(const string& s1, const string& s2) { return s1 + s2; }
int main() {
string string1("Starting string ");
string string2 = concTwoStrings(string1, " conc two strings");
cout << "string2: " << string2 << endl;
return EXIT_SUCCESS;
}
Output:
string2: Starting string conc two strings
Using the append()
Method
std::string
type is mutable and natively supports =
and +=
operators, the latter of which directly translates into in-place string concatenation. This operator can be used to concatenate a string
type variable, a string literal, a C-style string, or a character to a string
object. The following example shows two string variables getting appended to each other and outputted to the console.
#include <iostream>
#include <string>
using std::copy;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("Starting string ");
string string2("end of the string ");
cout << "string1: " << string1 << endl;
string1 += string2;
cout << "string1: " << string1 << endl;
return EXIT_SUCCESS;
}
Output:
string1: Starting string
string1: Starting string end of the string
Using the sprintf()
Function
For those who prefer a more C-style approach, the sprintf()
function can be used to concatenate strings in C++. This method is particularly useful when you want to format strings as well. Here’s how you can do it:
#include <iostream>
#include <cstdio>
int main() {
const char* str1 = "Hello, ";
const char* str2 = "World!";
char result[50]; // Ensure this buffer is large enough for the concatenated string
sprintf(result, "%s%s", str1, str2);
std::cout << result << std::endl;
return 0;
}
Output:
Hello, World!
In this example, we use sprintf()
to format and concatenate two C-style strings (str1
and str2
). The result is stored in the result
character array. The format specifier %s
is used to indicate that we are working with strings.
While sprintf()
can be powerful, it does come with some caveats. You need to ensure that the destination buffer is large enough to hold the concatenated string to avoid buffer overflows. Additionally, this method is less type-safe compared to using C++ string objects, so it’s generally recommended to use it with caution.
Conclusion
In summary, concatenating strings in C++ can be done using various methods, each with its own advantages and use cases. The +
operator is great for straightforward concatenations, while the append()
method allows for more controlled modifications of strings. If you prefer a C-style approach, sprintf()
provides powerful formatting capabilities. By understanding these methods, you can choose the best approach for your specific needs, leading to cleaner and more efficient code.
FAQ
-
What is string concatenation in C++?
String concatenation in C++ refers to the process of joining two or more strings together to form a single string. -
Can I concatenate strings of different types in C++?
Yes, you can concatenate different types of strings, such as C-style strings and C++ string objects, but you may need to convert them to a common type first. -
Is using the
+
operator for string concatenation efficient?
The+
operator is easy to use and efficient for small concatenations, but it may not be the best choice for concatenating strings in a loop due to performance concerns. -
What is the difference between
append()
and+
operator in C++?
Theappend()
method modifies the original string, while the+
operator creates a new string without altering the original. -
When should I use
sprintf()
for string concatenation?
You should usesprintf()
when you need formatted output or when working with C-style strings, but be cautious about buffer sizes to avoid overflow.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++