How to Clear the Console in C++
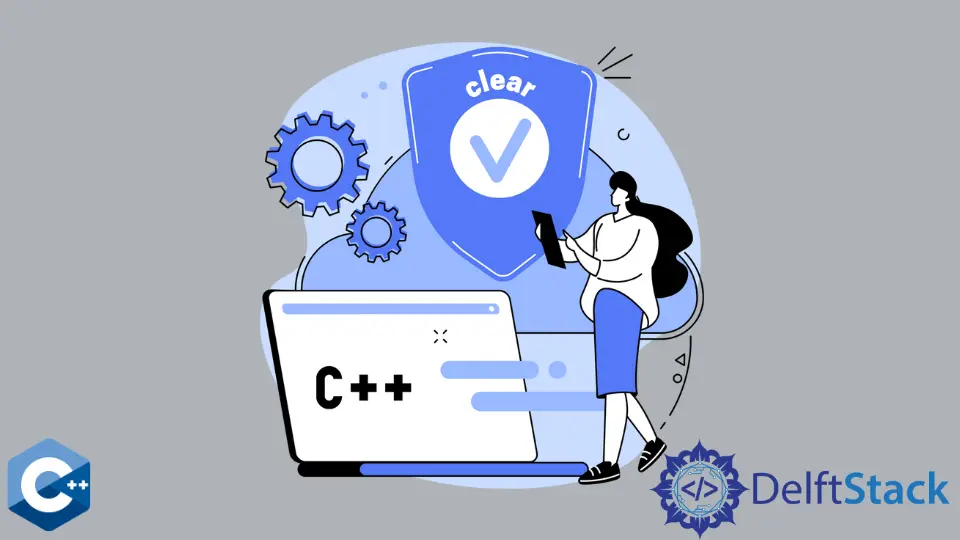
This article will explain several methods of how to clear console in C++.
Use ANSI Escape Codes to Clear Console
There are no built-in C++ language features to manipulate the console and clear the output text. However, ANSI escape codes can be a relatively portable way to achieve this goal. Escape codes are byte sequences starting with an ASCII Escape character and bracket character followed by parameters. These characters can be inserted into the output string, and the console interprets them as commands rather than text to display.
ANSI codes include multiple console output sequences with features like moving the cursor up/down, erasing in line, scrolling, and several other options. The following code example uses the Erase in Display
sequence that clears the entire screen and does not delete the scrollback buffer. Notice that we’ve constructed a separate function named clear
to make code more flexible and readable.
#include <iostream>
using std::cout;
using std::endl;
void Clear() { cout << "\x1B[2J\x1B[H"; }
int main() {
cout << "Some console filling text ..." << endl;
cout << "Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
<< endl;
Clear();
return EXIT_SUCCESS;
}
Alternatively, we can insert the same escape sequence with a slight modification (substitute 2 with 3) to clear the whole console screen and delete the scrollback buffer, as shown in the next code sample. Some useful ANSI control sequences are described in the following table. You could also refer to this Wikipedia page.
Code | Name | Effect |
---|---|---|
CSI n A |
Cursor Up | Move the terminal cursor up by n cells. The default value of cells is 1. If the cursor is already at the edge, the sequence command has no effect. |
CSI n B |
Cursor Down | Move the terminal cursor down by n cells. The default value of cells is 1. If the cursor is already at the edge, this sequence command has no effect. |
CSI n J |
Erase in Display | Clear part of the terminal window. If n is 0 or not specified, the command clears from the current position of the cursor to the end of the window. If n is 1, the command clears from the cursor position to the beginning of the window. The command clears the whole screen if the n is 2. If n is 3, the command clears the entire window and deletes lines in the scroll-back buffer. |
CSI n K |
Erase in Line | Erase the part of the line. If n is 0 or not specified, the command clears from the cursor to the end of the line. If n is 1, the command clears from the cursor to the beginning of the line. If n is 2, the entire line is cleared. |
#include <iostream>
using std::cout;
using std::endl;
void ClearScrollback() { cout << "\x1B[3J\x1B[H"; }
int main() {
cout << "Some console filling text ..." << endl;
cout << "Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
"Another filler string for the stdout\n"
<< endl;
ClearScrollback();
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook