The std::hash Template Class in C++
-
Use
std::hash
to Generate Hash forstd::string
Objects -
Use
std::hash
to Generate Hash forstd::bitset
Objects -
Use
std::hash
to Generate Hash forstd::vector<bool>
Objects
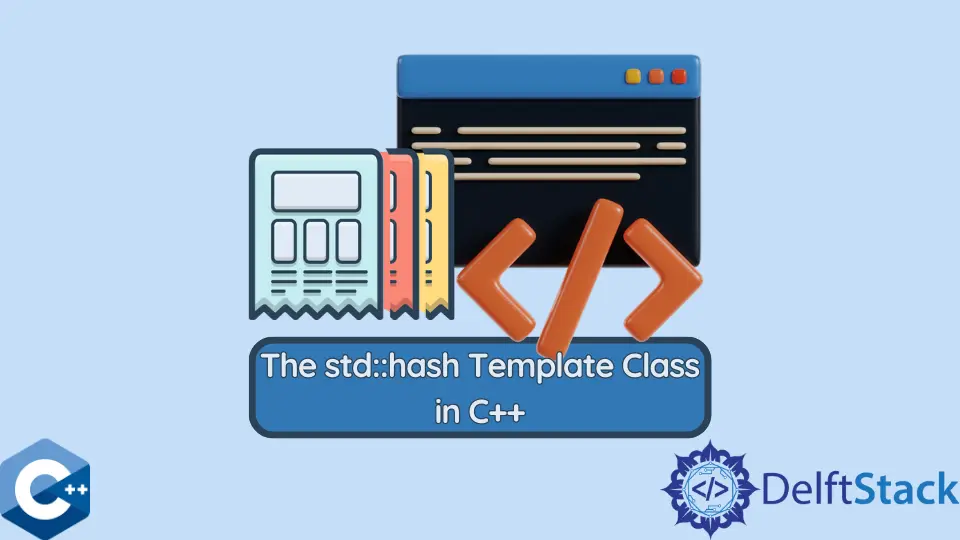
This article will introduce the std::hash
template class from STL in C++.
Use std::hash
to Generate Hash for std::string
Objects
The std::hash
template class is provided under the STL <functional>
header. It creates a hash function object. std::hash
satisfies the requirements of the DefaultConstructible
type, and it only requires to have template argument supplied.
Multiple default specializations of this template class are provided in C++ standard library, and the full list can be seen here. Once the hash function object is created with the given template argument, it can be utilized to generate specific hash values using operator()
that accepts a single argument and returns the size_t
value.
In the next example, we use string
specialization and generate some hash values for arbitrary strings.
#include <functional>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
using std::string;
int main() {
string str1("arbitrary string");
std::vector<string> vec2 = {"true", "false", "false",
"true", "false", "true"};
std::hash<string> str_hash;
cout << "hash(str1) - " << str_hash(str1) << endl;
cout << "hash(vec2 elements) - ";
for (const auto &item : vec2) {
cout << str_hash(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
hash(str1) - 3484993726433737991
hash(vec2 elements) - 1325321672193711394, 3658472883277130625, 3658472883277130625, 1325321672193711394, 3658472883277130625, 1325321672193711394,
Use std::hash
to Generate Hash for std::bitset
Objects
Another specialization of std::hash
provided in the STL is for std::bitset
arguments. Remember that std::bitset
is the class that represents a fixed number of bits as a sequence, and it provides multiple member functions for easy bit manipulation.
Generally, the hash functions used by std::hash
specializations are implementation-dependent, and one should not use these objects as a universal solution to hashing problem. Also, these hash functions are only required to produce the same output for the same input within a single execution of the program.
#include <bitset>
#include <functional>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
using std::string;
int main() {
std::bitset<8> b1("00111001");
std::hash<std::bitset<8>> bitset_hash;
cout << "hash(bitset<8>) - " << bitset_hash(b1) << endl;
return EXIT_SUCCESS;
}
Output:
hash(bitset<8>) - 6623666755989985924
Use std::hash
to Generate Hash for std::vector<bool>
Objects
We can also use std::hash
specialization for a vector
of boolean values, as shown in the following code snippet. Note that std::hash
can also be specialized for user-defined classes, and some additional specializations are available through Boost library (details of which are listed here.
#include <functional>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
using std::string;
int main() {
std::vector<bool> vec1 = {true, false, false, true, false, true};
std::hash<std::vector<bool> > vec_str_hash;
cout << "hash(vector<bool>) - " << vec_str_hash(vec1) << endl;
return EXIT_SUCCESS;
}
Output:
hash(vector<bool>) - 12868445110721718657
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook