How to Handle Arguments Using getopt in C++
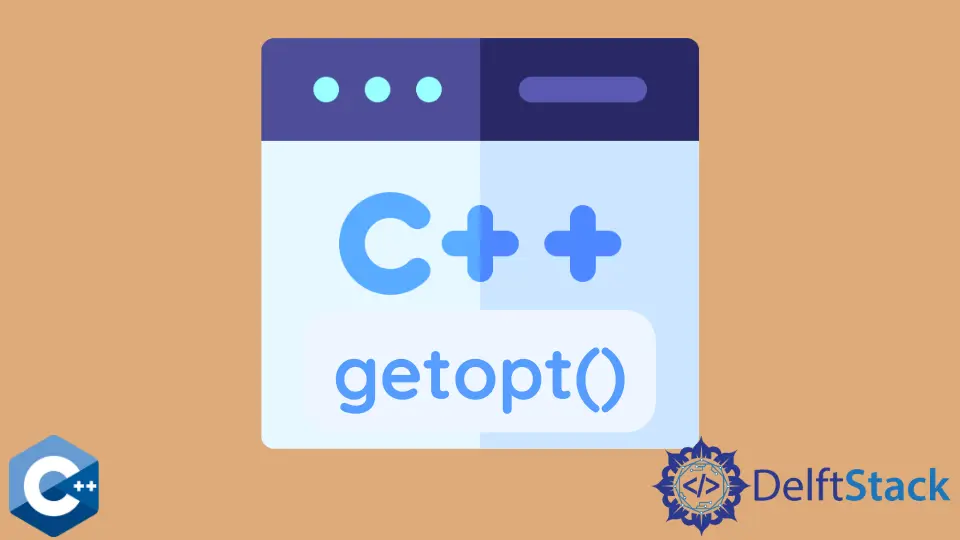
This article demonstrates how to use the getopt()
function to deal with arguments passed in the command line to the code. The tutorial also explains how the code can be manipulated to perform certain actions with specific input.
Handle Arguments Using the getopt()
Function in C++
Suppose we have the code shown below.
// File titled arg_test.cpp
#include <stdio.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
int opt;
while ((opt = getopt(argc, argv, ":f:asd")) != -1) {
if (opt == 'a' || opt == 's' || opt == 'd') {
printf("Option Selected is: %c\n", opt);
} else if (opt == 'f') {
printf("Filename entered is: %s\n", optarg);
} else if (opt == ':') {
printf("Option requires a value \n");
} else if (opt == '?') {
printf("Unknown option: %c\n", optopt);
}
}
// optind is for the extra arguments that are not parsed by the program
for (; optind < argc; optind++) {
printf("Extra arguments, not parsed: %s\n", argv[optind]);
}
return 0;
}
The syntax of the getopt()
function is as follows:
getopt(int argc, char *const argv[], const char *optstring)
The argc
is an integer, and argv
is a character array. The optstring
is a list of characters, each representing an option that is a single character long.
The function returns -1
when it is done processing everything in the input.
The getopt()
function returns ?
when it encounters something it doesn’t recognize. Furthermore, this unrecognized option is stored in the external variable optopt
.
By default, if the option requires a value, for example, in our case, the option f
requires an input value, so getopt()
will return ?
. However, by placing the colon (:
) as the first character of optstring
, the function returns :
instead of ?
.
In our case, entering an input of a
, s
, or d
should return the option itself, using f
should return the filename provided as an argument, and everything else should not be considered a valid option.
To run the above code, you can make use of a similar command in your terminal:
gcc arg_test.cpp && ./a.out
Of course, just entering this will not give you any useful output. We will enter an example use case and a sample output and explain how each command is processed.
If we enter the following command as an example:
gcc arg_test.cpp && ./a.out -f filename.txt -i -y -a -d testingstring not_an_option
We’ll get the following as the output:
Filename entered is: filename.txt
Unknown option: i
Unknown option: y
Option Selected is: a
Option Selected is: d
Extra arguments, not parsed: testingstring
Extra arguments, not parsed: not_an_option
The options a
and d
were returned as expected. The filename given after the f
flag is also reported, and the options i
and y
went into the ?
category and were recognized as unknown commands.
Here, we can see that in addition to the above-described scenarios, the last loop we added after the getopt()
function processes the remaining arguments that were not processed by the function (testingstring
and not_an_option
, in our case).
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn