How to Get File Extension in C++
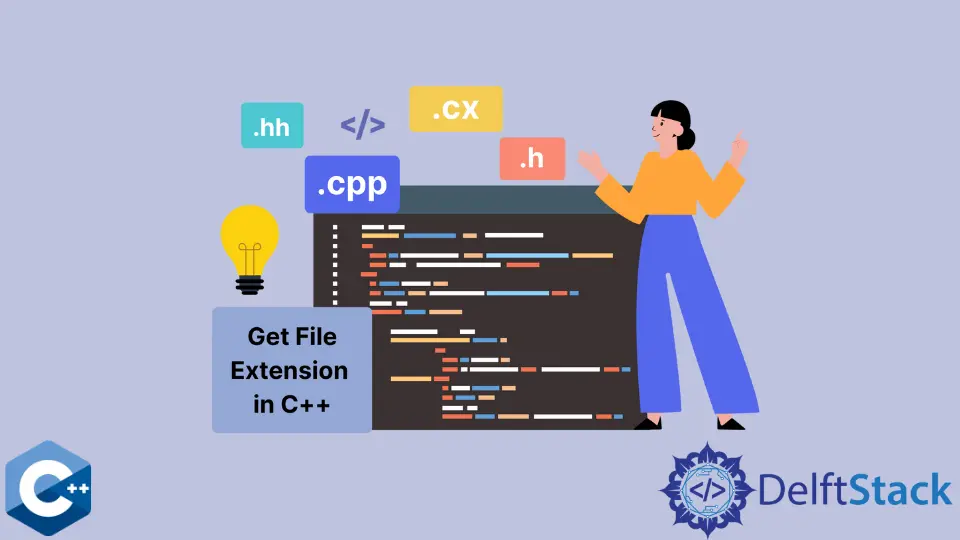
A file extension refers to the last part of a file name that contains information about the data held in the file.
In C++, we can use either .cpp
or .cxx
extensions for files containing C++ code. However, using only one extension per directory is recommended, so it’s best to pick one and stick with it throughout your project.
The next most common extension for C++ files is .h
and .hh
, which are header files that contain information about functions, classes, variables, and other features of the program that can be used by other parts of the project, such as other header files or source code files.
This article will discuss the steps to get the file extension from a string in C++. Let’s begin.
Get the File Extension From a String in C++
The following are the steps we can do to obtain the file extension using C++:
-
The first step is to include the header file that contains the function we need to use.
-
The second step is to declare a variable that will hold the file type extension we want. We can call this variable
extension
. -
The third step is to call the function and assign its return value (the extension) to our variable,
extension
. -
Finally, we can check if our string matches the desired file type by comparing it with our variable
extension
. If they match, then you have found your file type!
Let’s discuss an example to better understand the concept mentioned above.
#include <iostream>
using namespace std;
int main() {
string x = "write the name of your file here.cx";
if (x.substr(x.find_last_of("*******") + 2) == "cx") {
// Here we are finding the file
cout << "Done";
} else {
cout << "Not done";
}
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook