How to Generate Random Number in Range in C++
-
Use C++11
<random>
Library to Generate a Random Number in Range -
Use the
rand
Function to Generate a Random Number in Range
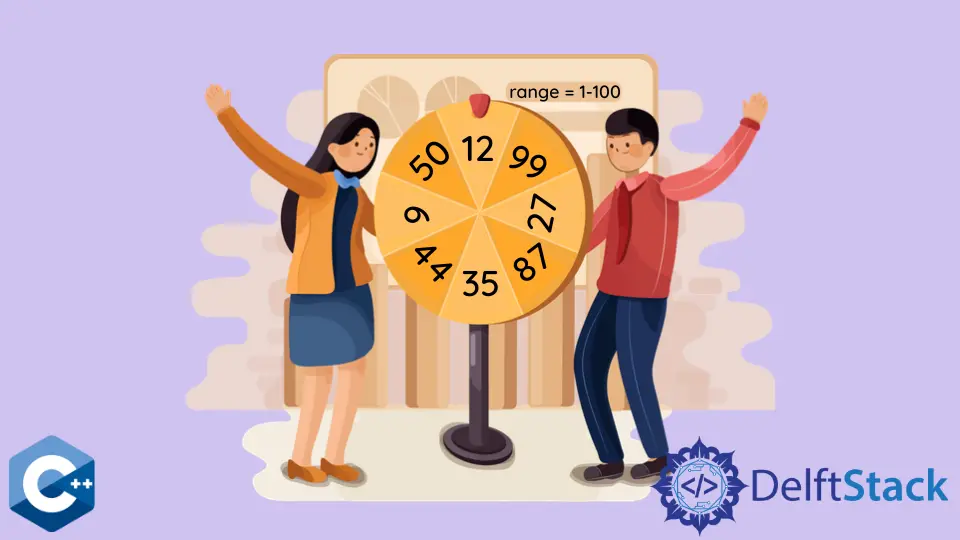
This article will introduce multiple C++ methods of how to generate random numbers in a specified number interval.
Use C++11 <random>
Library to Generate a Random Number in Range
The C++ added standard library facilities for random number generation with C++11 release under a new header <random>
. RNG workflow features provided by the <random>
header is divided into two parts: random engine and distribution. The random engine is responsible for returning unpredictable bitstream. Distribution returns random numbers (of type as specified by the user) that satisfy the specific probability distribution e.g. uniform, normal or other.
At first, the user should initialize the random engine with the seed value. It’s recommended to seed the engine with std::random_device
, the system-specific source for non-deterministic random bits. It allows the engine to generate different random bitstreams on each run. On the other hand, if the user needs to generate the same sequences across multiple program runs, the random engine should be initialized with the constant int
literal.
Next, the distribution object is initialized with arguments of min/max values for an interval, from where the random numbers are generated. In the following example, we use uniform_int_distribution
and output 10 integers to console arbitrarily.
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int n = 0; n < RAND_NUMS_TO_GENERATE; ++n) {
cout << distr(eng) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output (*random):
57; 38; 8; 69; 5; 27; 65; 65; 73; 4;
<random>
header provides multiple random engines with different algorithms and efficiency trade-offs. Hence, we can initialize the specific random engine, as shown in the next code sample:
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::random_device rd;
std::mt19937 eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int n = 0; n < RAND_NUMS_TO_GENERATE; ++n) {
cout << distr(eng) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output (*random):
59; 47; 81; 41; 28; 88; 10; 12; 86; 7;
Use the rand
Function to Generate a Random Number in Range
The rand
function is part of the C standard library and can be called from the C++ code. Although it’s not recommended to use the rand
function for high-quality random number generation, it can be utilized to fill arrays or matrices with arbitrary data for different purposes. In this example, the function generates a random integer between 0 and MAX
number interval. Note that this function should be seeded with std::srand
(preferably passing the current time with std::time(nullptr)
) to generate different values across the multiple runs, and only then we can call the rand
.
#include <ctime>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < RAND_NUMS_TO_GENERATE; i++) cout << rand() % MAX << "; ";
cout << endl;
return EXIT_SUCCESS;
}
Output (*random):
36; 91; 99; 40; 3; 60; 90; 63; 44; 22;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook