Garbage Collection in C++
- Garbage Collection as a Memory Management Technique
- Manual Memory Management in C++
- Issues with Manual Memory Management in C++
- Algorithms for Garbage Collection
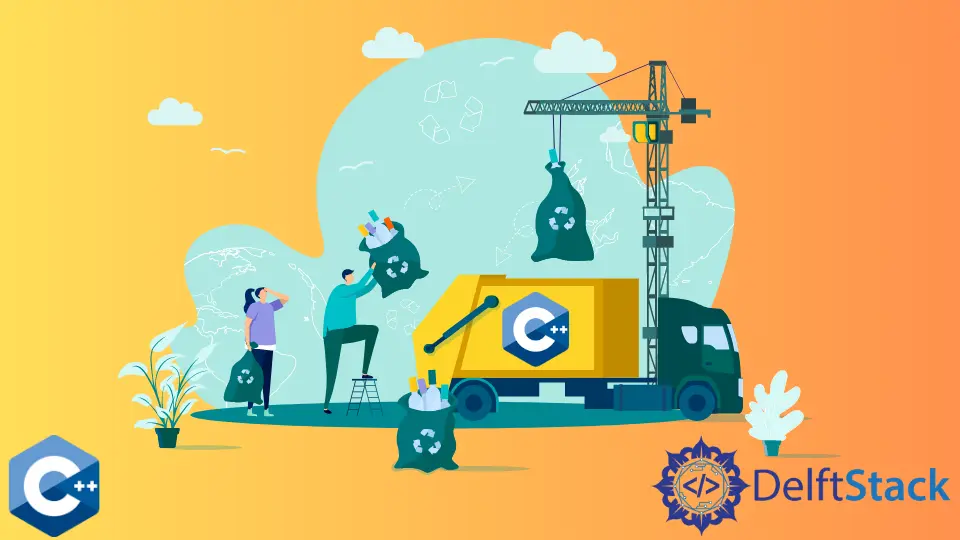
In this article, we will learn about garbage collection in C++.
Garbage Collection as a Memory Management Technique
Garbage collection is one of the memory management techniques used in programming languages. It is an automatic memory management technique added as a feature for many programming languages.
The garbage collector collects or reclaims the memory allocated to variables or objects but is no longer in use by the program; this is also termed garbage.
One thing to note is that garbage collector only manages memory o resources like files. UI windows and destructors are not handled by it.
Languages like Java, C#, and most scripting languages have garbage collection as part of the language for better efficiency.
But in languages like C++, we have manual memory management, which means the programmer has to manually do it using commands like new,
delete,
or some algorithm.
Manual Memory Management in C++
Dynamic memory allocation is a type of memory allocated during the run-time in the heap
area. Once we stop using that memory, it must be released; else, it might lead to a memory leak.
Memory allocation and deallocation in C++ are manually done using commands like new
or delete.
The new
keyword allocates the memory dynamically from the heap.
Once usage is done, we use the delete
keyword to deallocate and clear the memory. Internally, it calls the destructor
to destroy the memory.
Hence, new
should always be followed by the delete
command to avoid memory leaks.
Example code:
#include <bits/stdc++.h>
using namespace std;
int main() {
int *arr = new int[5]; // 5 blocks of memory allocated
if (!arr)
cout << "Memory allocation failed\n";
else {
for (int i = 0; i < 5; i++) arr[i] = i + 1;
cout << "Value stored in memory blocks ";
for (int i = 0; i < 5; i++) cout << arr[i] << " ";
}
delete[] arr; // deallocates the block of memory
}
Output:
Value stored in memory blocks 1 2 3 4 5
Issues with Manual Memory Management in C++
One major issue is that we might forget to use delete,
leading to a memory leak. The second major problem is when dealing with extensive programs where a lot of dynamic memory allocation occurs, we might accidentally deallocate the memory already in use.
Algorithms for Garbage Collection
We can use different garbage collection algorithms like mark and sweep,
copying,
and reference counting
so that a program, especially for languages like C++, can simultaneously have garbage collection and manual memory management.
A reference count is associated with each dynamic memory allocation in the reference counting
algorithm. The reference count increases when a new reference is created and is decremented when a reference is deleted.
When this count reaches 0, it signifies that memory is no longer in use, and we can release it.