Function Overloading in C++
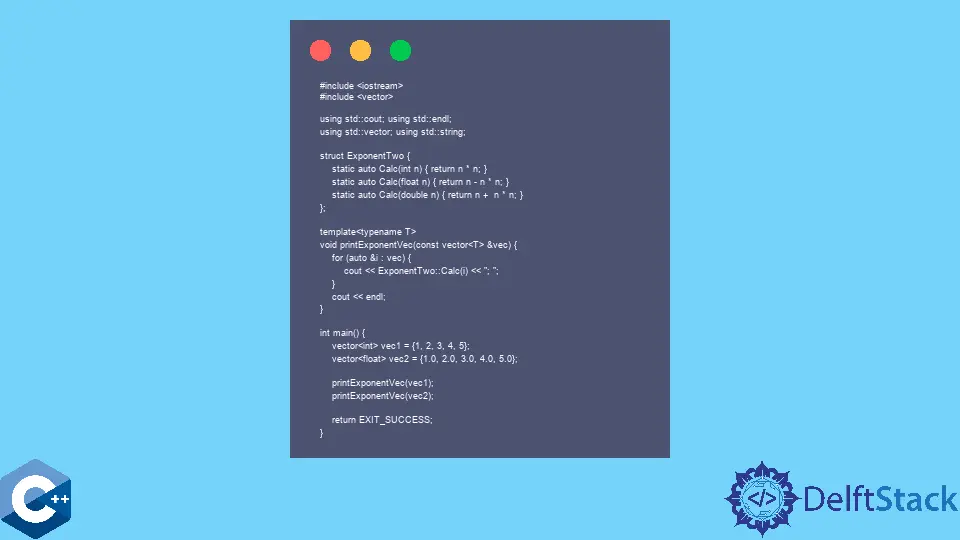
This article will demonstrate multiple methods of using function overloading in C++.
Define Overloaded Functions in C++
Function overloading is known as a part of ad hoc polymorphism that provides the functions that can be applied to objects of different types. Namely, these functions are defined with the same name in the given scope, and they should differ in the number or types of arguments. Note that overloaded functions are just a set of different functions which are resolved at compile time. The latter feature differentiates them from run-time polymorphism, which is used for virtual functions. The compiler observes the type of the arguments and chooses which function to call.
Overloaded functions must differ in parameter type or a number of parameters. Otherwise, the compiler error will occur. Even when the return type is different among overloaded functions or aliased types are used as arguments, the compiler fails with an error. In the following example, we implemented a Math
structure that has three static
functions for number summation. Each of the functions takes different types of argument pairs and returns the corresponding type. As a result, the Math::sum
can be invoked with vector elements of a different type. Note that, struct
keyword defines a class in C++ and its members are public by default.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct Math {
static auto sum(int x, int y) { return x + y; }
static auto sum(float x, float y) { return x + y; }
static auto sum(double x, double y) { return x + y; }
};
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
for (const auto &item : vec1) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
for (const auto &item : vec2) {
cout << Math::sum(item, item * 2) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
3; 6; 9; 12; 15;
3; 6; 9; 12; 15;
Use Overloaded Functions with Templates in C++
Templates are a form of generic programming implemented in the C++ language. It has similar features to function overloading, as both are inferred at compile time. Notice that the following code snippet has the printExponentVec
function that outputs the elements of the generic vector. Thus it is utilized to conduct the same operation for each type, but when we want to implement different function bodies for different types, function overloading should be used.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct ExponentTwo {
static auto Calc(int n) { return n * n; }
static auto Calc(float n) { return n - n * n; }
static auto Calc(double n) { return n + n * n; }
};
template <typename T>
void printExponentVec(const vector<T> &vec) {
for (auto &i : vec) {
cout << ExponentTwo::Calc(i) << "; ";
}
cout << endl;
}
int main() {
vector<int> vec1 = {1, 2, 3, 4, 5};
vector<float> vec2 = {1.0, 2.0, 3.0, 4.0, 5.0};
printExponentVec(vec1);
printExponentVec(vec2);
return EXIT_SUCCESS;
}
Output:
1; 4; 9; 16; 25;
0; -2; -6; -12; -20;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook