How to Find Element Index in Vector in C++
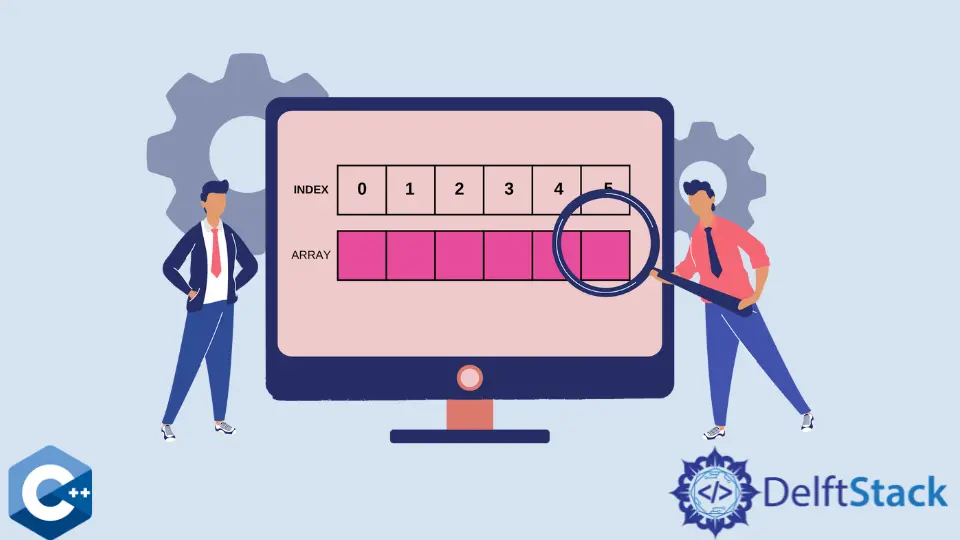
In the world of C++, vectors are a fundamental part of the Standard Template Library (STL). They provide a dynamic array that can resize itself automatically when elements are added or removed. One common task that programmers often face is finding the index of a specific element within a vector.
This article demonstrates how to find element index in vector in C++. Whether you’re a beginner or an experienced developer, understanding how to efficiently retrieve an index can save you time and enhance your coding skills. We’ll explore various methods to accomplish this task, complete with code examples and explanations, ensuring you have a comprehensive understanding of the topic.
Using std::find
One of the simplest ways to find an element’s index in a vector is by using the std::find
algorithm from the <algorithm>
header. This method is straightforward and leverages the power of STL algorithms to locate an element.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
int element = 30;
auto it = std::find(vec.begin(), vec.end(), element);
if (it != vec.end()) {
int index = std::distance(vec.begin(), it);
std::cout << "Element " << element << " found at index: " << index << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
}
Output:
Element 30 found at index: 2
This method begins by including the necessary headers, <iostream>
, <vector>
, and <algorithm>
. We then define a vector containing integers and specify the element we want to find. The std::find
function searches the vector for the specified element. If found, we calculate the index using std::distance
, which provides the distance between the beginning of the vector and the iterator returned by std::find
. This is a clean and efficient way to locate an element’s index.
Using a Loop
Another method to find the index of an element in a vector is by manually iterating through the vector using a loop. This approach gives you more control and can be easier to understand for those new to C++.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
int element = 40;
int index = -1;
for (size_t i = 0; i < vec.size(); ++i) {
if (vec[i] == element) {
index = i;
break;
}
}
if (index != -1) {
std::cout << "Element " << element << " found at index: " << index << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
}
Output:
Element 40 found at index: 3
In this example, we define a vector and the element we wish to find, initializing an index variable to -1 to indicate that the element has not been found. The loop iterates over each element in the vector, checking if it matches the target element. If a match is found, we store the index and exit the loop. After the loop, we check if the index was updated, allowing us to print the result accordingly. This method is straightforward and effective, especially for those who prefer traditional control structures.
Using std::find_if with a Lambda Function
For a more advanced approach, we can utilize std::find_if
in combination with a lambda function. This method is particularly useful when searching for elements based on specific conditions rather than direct value comparison.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
int element = 50;
auto it = std::find_if(vec.begin(), vec.end(), [element](int value) {
return value == element;
});
if (it != vec.end()) {
int index = std::distance(vec.begin(), it);
std::cout << "Element " << element << " found at index: " << index << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
}
Output:
Element 50 found at index: 4
In this code snippet, we again use the std::find_if
function, but this time, we pass a lambda function that checks if each vector element matches the target element. This method is powerful because it allows you to define more complex conditions for finding elements. The process of calculating the index remains the same, utilizing std::distance
to find the position of the iterator. This approach is especially useful when dealing with custom objects or more complicated conditions.
Conclusion
Finding the index of an element in a vector in C++ can be achieved through various methods, each with its own advantages. Whether you choose to use std::find
, a simple loop, or std::find_if
with a lambda function, understanding these techniques will enhance your programming toolkit. As you practice these methods, you’ll become more comfortable with C++ STL and improve your overall coding efficiency. Keep experimenting with different approaches to find what works best for your specific needs.
FAQ
- What is a vector in C++?
A vector is a dynamic array provided by the Standard Template Library (STL) in C++, which can resize itself automatically when elements are added or removed.
-
How do I check if an element exists in a vector?
You can usestd::find
to check for the existence of an element in a vector. If the result is not equal to the end iterator, the element exists. -
Can I find the index of a custom object in a vector?
Yes, you can find the index of a custom object by usingstd::find_if
with a lambda function that defines the condition for equality. -
What happens if the element is not found?
If the element is not found, the iterator returned bystd::find
orstd::find_if
will be equal to the end iterator, which you can check to handle the absence of the element. -
Is using a loop to find an index efficient?
Using a loop is generally less efficient than STL algorithms for large vectors, but it can be easier to understand and implement for simple cases.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook