How to Generate Fibonacci Numbers in C++
- Understanding the Fibonacci Sequence
- Method 1: Iterative Approach
- Method 2: Recursive Approach
- Method 3: Dynamic Programming
- Conclusion
- FAQ
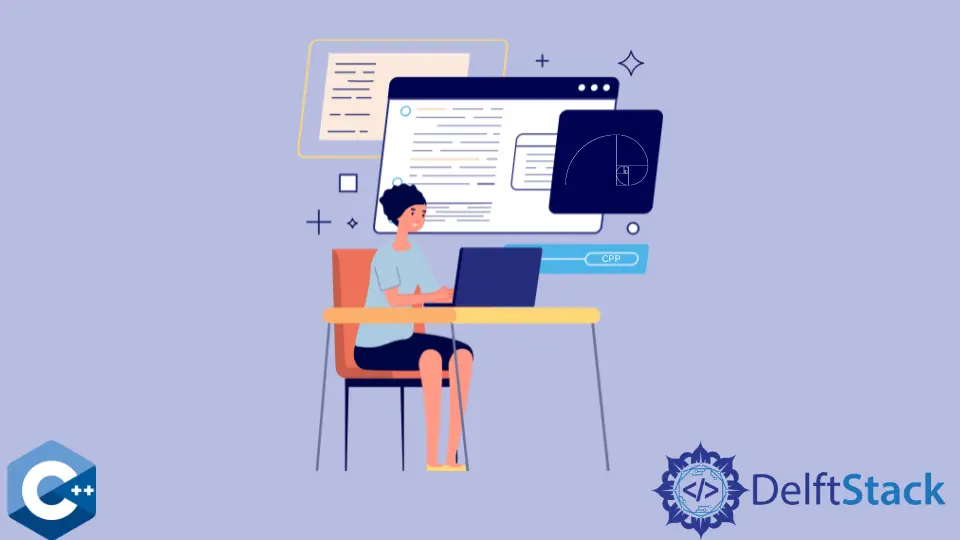
Generating Fibonacci numbers is a fascinating topic that blends mathematics with programming. Whether you’re a beginner learning the ropes or a seasoned developer looking to brush up on your skills, understanding how to generate Fibonacci numbers in C++ is a valuable exercise. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, typically starting with 0 and 1.
In this article, we’ll explore multiple methods to generate Fibonacci numbers in C++, complete with code examples and explanations. By the end, you’ll have a solid grasp of how to implement this classic algorithm in your own projects.
Understanding the Fibonacci Sequence
Before diving into the code, let’s recap what the Fibonacci sequence is. The sequence starts with 0 and 1, and each subsequent number is the sum of the two preceding numbers. The sequence looks like this:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, …
This pattern continues infinitely, and it has applications in various fields, including computer science, mathematics, and even nature. Now, let’s explore different methods to generate these numbers in C++.
Method 1: Iterative Approach
The iterative approach is one of the most straightforward ways to generate Fibonacci numbers. It uses a loop to calculate each number in the sequence up to a specified limit.
#include <iostream>
using namespace std;
void generateFibonacci(int n) {
int a = 0, b = 1, c;
if (n >= 1) cout << a << " ";
if (n >= 2) cout << b << " ";
for (int i = 2; i < n; i++) {
c = a + b;
cout << c << " ";
a = b;
b = c;
}
}
int main() {
int n = 10;
generateFibonacci(n);
return 0;
}
Output:
0 1 1 2 3 5 8 13 21 34
This code defines a function generateFibonacci
that takes an integer n
as input, representing the number of Fibonacci numbers to generate. The function initializes the first two Fibonacci numbers, 0 and 1, and prints them if n
is greater than or equal to their respective indices. Then, it enters a loop that iterates from 2 to n
, calculating each Fibonacci number by summing the two previous numbers. The values of a
and b
are updated in each iteration, ensuring that the next Fibonacci number is calculated correctly.
Method 2: Recursive Approach
The recursive method for generating Fibonacci numbers is elegant but less efficient due to its exponential time complexity. It involves calling the same function multiple times, which can lead to a large number of calculations.
#include <iostream>
using namespace std;
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
int main() {
int n = 10;
for (int i = 0; i < n; i++) {
cout << fibonacci(i) << " ";
}
return 0;
}
Output:
0 1 1 2 3 5 8 13 21 34
In this code, the fibonacci
function checks if n
is 0 or 1, returning n
directly. If n
is greater than 1, it recursively calls itself twice to compute the two preceding Fibonacci numbers. The main
function iterates through the first n
Fibonacci numbers, calling the fibonacci
function for each index and printing the results. While this method is straightforward, its inefficiency becomes apparent as n
increases, making it suitable only for small values.
Method 3: Dynamic Programming
To improve the efficiency of the recursive approach, we can use dynamic programming. This method stores previously calculated Fibonacci numbers, significantly reducing the number of function calls.
#include <iostream>
#include <vector>
using namespace std;
void generateFibonacci(int n) {
vector<int> fib(n);
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i < n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
for (int i = 0; i < n; i++) {
cout << fib[i] << " ";
}
}
int main() {
int n = 10;
generateFibonacci(n);
return 0;
}
Output:
0 1 1 2 3 5 8 13 21 34
In this implementation, we use a vector to store Fibonacci numbers. The first two numbers are initialized, and then a loop calculates the rest by adding the two previous numbers. Finally, we print all the Fibonacci numbers stored in the vector. This method is efficient because it only requires linear time, making it suitable for larger values of n
.
Conclusion
Generating Fibonacci numbers in C++ can be accomplished through various methods, each with its own strengths and weaknesses. The iterative approach is simple and efficient for most cases. The recursive method is elegant but can be impractical for larger values due to its exponential time complexity. Lastly, the dynamic programming approach strikes a balance between clarity and efficiency, making it a great choice for larger inputs. By mastering these techniques, you can enhance your programming skills and apply them in various computational problems.
FAQ
-
What is the Fibonacci sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, starting with 0 and 1. -
Why is the recursive approach inefficient for generating Fibonacci numbers?
The recursive approach recalculates Fibonacci numbers multiple times, leading to exponential time complexity, which becomes impractical for larger inputs. -
How does dynamic programming improve the Fibonacci generation process?
Dynamic programming stores previously calculated Fibonacci numbers, reducing redundant calculations and improving efficiency to linear time complexity. -
Can I generate Fibonacci numbers in other programming languages?
Yes, Fibonacci numbers can be generated in virtually any programming language, including Python, Java, and JavaScript, using similar algorithms. -
What are some real-world applications of Fibonacci numbers?
Fibonacci numbers appear in various fields, including computer algorithms, financial modeling, and even in nature, such as in the arrangement of leaves and flowers.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook