How to Understand the Extern Keyword in C++
-
the
extern
Keyword in C++ - Syntax of External Variable and External Function in C++
-
Advantages of Using the
extern
Keyword in C++
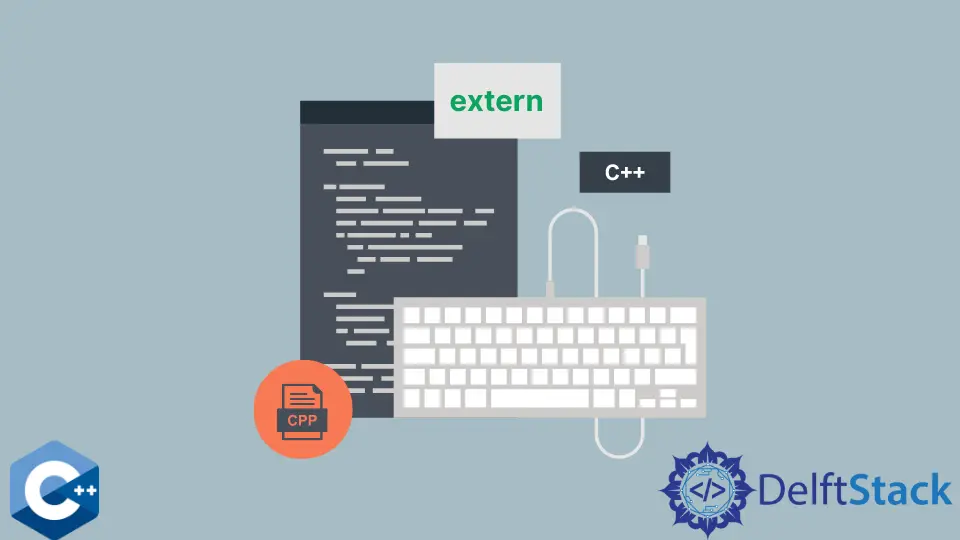
This article will introduce the extern
keyword in C++, the syntax of external variables and functions, and the uses of using this keyword.
the extern
Keyword in C++
The keyword extern
denotes external or global variables and external functions. This keyword tells the compiler that the variable is global in many source files.
The extern
keyword is very useful when combining (linking) multiple source files as a single program.
External variables are declared outside the main function right after the header files. The scope of the external variables is global, and its lifetime is equivalent to the static variables (i.e., the lifetime is equal to the lifetime of the program).
Syntax of External Variable and External Function in C++
The external variables and external functions can be declared using the following syntax:
Syntax of External Variable:
extern datatype variable_name;
Example : extern int a = 40;
Syntax of External Function:
extern datatype function_name();
Example : extern int add();
Example:
Suppose you have two source files: first.cpp
and second.cpp
with the following codes:
"first.cpp" Int a = 40;
Int b = 50;
Void add();
Int main() {
add();
return 0;
}
"Second.cpp" extern int a;
extern int b;
Void add() { a + b; }
In the example above, variables a
and b
are defined in first.cpp
. Then to utilize both of these variables in second.cpp
, both must be declared.
Advantages of Using the extern
Keyword in C++
The following are the advantages of using the extern keyword in a C++ program:
- The
extern
keyword tells the compiler about the existence of the external variables and their potential use in another translation unit or source file. - It increases the visibility of variables and functions.
- This keyword makes it easy to understand errors such as duplicate symbol errors.
- We use an
extern
keyword for the modern linker to increase readability. - It makes the code maintenance.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn