exit(1) in C++
-
Use the
exit
Function to Terminate C++ Program With Status Code -
Use
exit(1)
to Terminate C++ Program With Failure Status Code
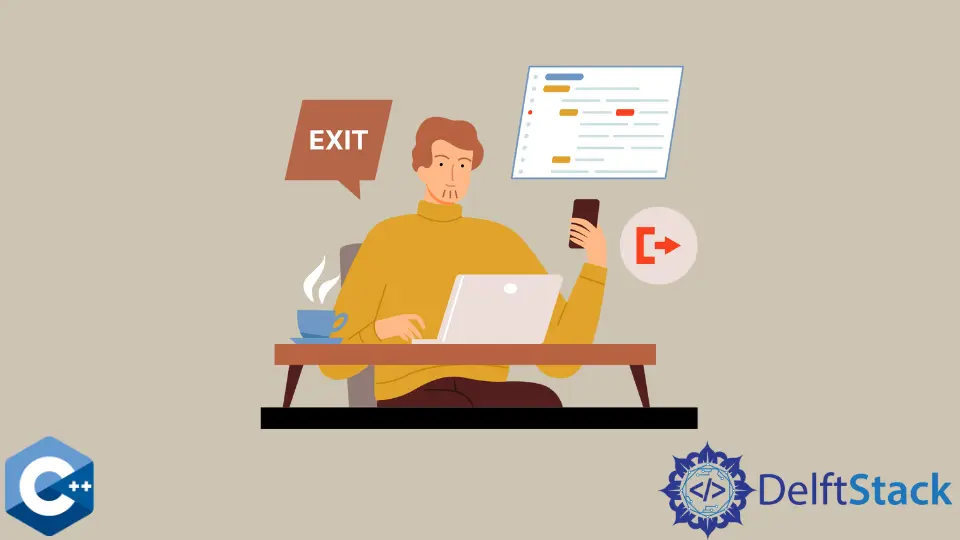
This article will explain how to use the exit()
function in C++.
Use the exit
Function to Terminate C++ Program With Status Code
The running program is generally called a process in an operating system, and it has its life cycle, which is represented as different states. There are long-running programs like web servers, but even those need to be terminated in some circumstances or by delivering the user’s signal. Process termination can be invoked with the exit()
function, which is part of the C standard and included in C++. It takes one integer argument that specifies the program termination status that can be read from the parent process if the child is waited upon.
The conventional value of the successful return status code is 0
, and anything other than zero is considered an error code, which may correspond to the predefined scenario. Note that there are two macro expressions called EXIT_SUCCESS
and EXIT_FAILURE
to denote the corresponding exit codes passed to the exit
call. Generally, the program has multiple file streams and temporary files open, which get closed or removed automatically when the exit
is invoked.
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
int main() {
printf("Executing the program...\n");
sleep(3);
exit(0);
// exit(EXIT_SUCCESS);
}
Output:
Executing the program...
Use exit(1)
to Terminate C++ Program With Failure Status Code
exit
function with the argument value of 1
is used to denote the termination with failure. Some functions that return the value of a successful call status code could be combined with the conditional statement that verifies the return value and exits the program if the error occurred. Note that exit(1)
is the same as calling the exit(EXIT_FAILURE)
.
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
int main() {
if (getchar() == EOF) {
perror("getline");
exit(EXIT_FAILURE);
}
cout << "Executing the program..." << endl;
sleep(3);
exit(1);
// exit(EXIT_SUCCESS);
}
Output:
Executing the program...
Another useful feature of the exit()
function is to execute specially registered functions before the program is finally terminated. These functions can be defined as regular functions, and to be called upon the termination, they need to be registered with the atexit
function. atexit
is part of the standard library and takes a function pointer as the only argument. Notice that multiple functions can be registered using the multiple atexit
calls, and each of them is invoked in reverse order once the exit
executes.
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
static void at_exit_func() { cout << "at_exit_func called" << endl; }
static void at_exit_func2() { cout << "at_exit_func called" << endl; }
int main() {
if (atexit(at_exit_func) != 0) {
perror("atexit");
exit(EXIT_FAILURE);
}
if (atexit(at_exit_func2) != 0) {
perror("atexit");
exit(EXIT_FAILURE);
}
cout << "Executing the program..." << endl;
sleep(3);
exit(EXIT_SUCCESS);
}
Output:
Executing the program...
at_exit_func2 called
at_exit_func called
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook