Difference Between Structure and Class in C++
-
the
struct
vs.class
Keywords in C++ -
Access Modifiers in
class
vs.struct
in C++ -
Memory Allocation in
class
vs.struct
in C++ -
Null Values in
class
vs.struct
in C++ -
Value Type vs. Reference Type in
class
vs.struct
in C++ -
Security in
class
vs.struct
in C++
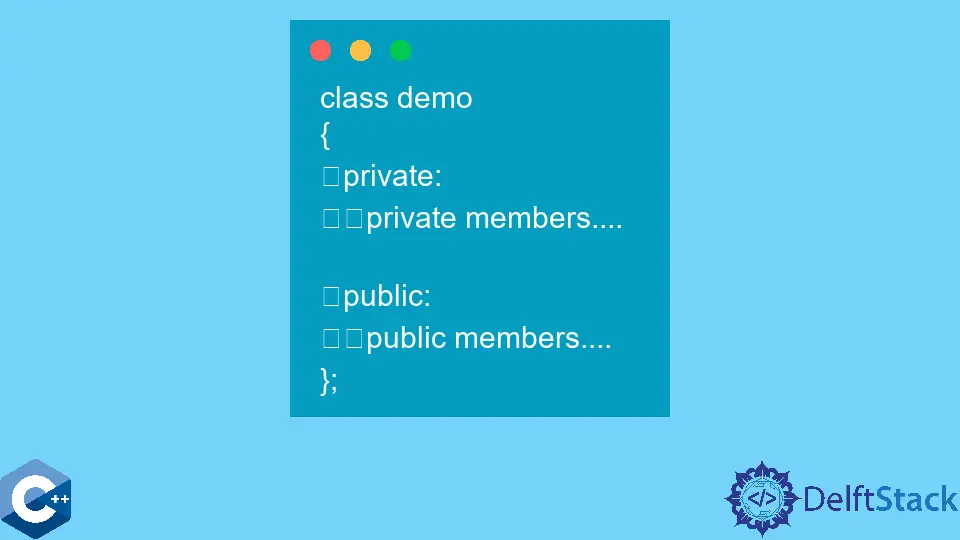
This article explains the difference between a structure and a class in C++. This article is written concerning the latest version of C++; there were many more restrictions and differences between a struct
and a class
in the old versions.
In most cases, the structure
is quite similar to a class
, but few differences. Let’s look at them one by one.
the struct
vs. class
Keywords in C++
A structure is declared using the struct
keyword, whereas the class
keyword is used to declare a class.
The syntax for a class:
class demo {
private:
private
members...
.
public : public members....
};
The syntax for a structure:
struct demo {
members1;
members2;
...
};
Access Modifiers in class
vs. struct
in C++
The class
and struct
in C++ have three access specifiers public
, private
, and protected
, but in C++, members of a structure are public
by default, and members of a class are private
by default.
Example code using class
:
#include <bits/stdc++.h>
using namespace std;
class demo {
int temp;
};
int main() {
demo d;
d.temp = 20;
}
Output: We get a compile error because the temp
is private.
[Error] int demo::temp is private
[Error] within this context
Example code using struct
:
#include <bits/stdc++.h>
using namespace std;
struct demo {
int temp;
};
int main() {
demo d;
d.temp = 20;
cout << d.temp;
}
Output:
20
Memory Allocation in class
vs. struct
in C++
Whenever a structure is created and implemented, memory is allocated in the stack
area, but memory is allocated in the heap
area when a class is created and implemented.
Null Values in class
vs. struct
in C++
Members of structure cannot be null
values, whereas class members can have null
values.
Value Type vs. Reference Type in class
vs. struct
in C++
In C++, Structures are a value type, and Classes are a reference type. Value types store their content on a stack, and they directly hold the value where they are declared, so whenever a struct variable is created, the variable directly contains the data of the struct
.
But in the case of a class of reference type, whenever an object is created, it holds the reference
to it instead of the data.
Security in class
vs. struct
in C++
Security is a major issue concern with structures because we cannot hide the end-users implementation details, but design details can easily be hidden in class implementation.
Let’s summarize the differences in a tabular form.
Structure | Class |
---|---|
Use the struct keyword to define a structure. |
Use the class keyword to define a class. |
Less secure. | More secure as implementations can be hidden from the end-user. |
Memory is allocated in the stack area. |
Memory is allocated in the heap area. |
Members cannot have null values. |
Members can have null values. |
Access modifier is public by default. |
Access modifier is private by default. |
Structure is a value type . |
Class is a reference type . |