How to Use the delete Operator in C++
-
Using the
delete
Operator for Single Objects -
Using the
delete
Operator for Arrays -
Use the
delete
Operator to Free Elements in Vector - Common Mistakes with the Delete Operator
- Best Practices for Using the Delete Operator
- Conclusion
- FAQ
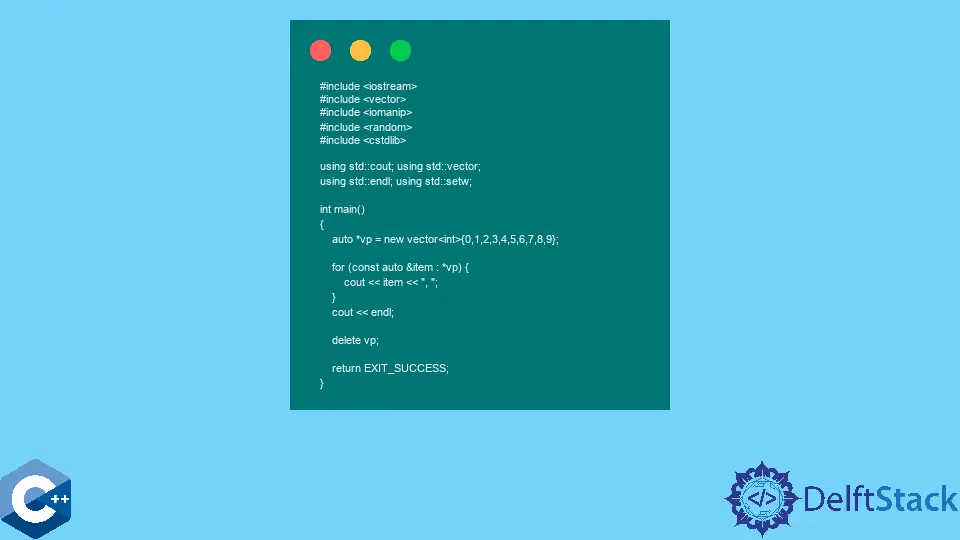
In C++ programming, memory management is a critical skill that every developer must master. One of the key components of this management is the delete operator. This operator is essential for freeing up memory that was previously allocated using the new operator. Failing to properly use delete can lead to memory leaks, which can degrade performance and lead to unpredictable behavior in your applications.
In this article, we will explore how to effectively use the delete operator in C++, providing you with practical examples and explanations to enhance your understanding. Whether you’re a beginner or looking to refine your skills, this guide will serve as a valuable resource in your C++ programming journey.
The delete operator in C++ is used to deallocate memory that was previously allocated using the new operator. When you allocate memory dynamically, it is crucial to release that memory once you’re done with it. If not, your application may consume more memory than necessary, leading to performance issues or crashes. The delete operator comes in two forms: delete
and delete[]
. The former is used for single objects, while the latter is used for arrays.
Using the delete
Operator for Single Objects
When you allocate a single object using the new operator, you should use delete to free that memory. Here’s a simple example:
#include <iostream>
class MyClass {
public:
MyClass() {
std::cout << "Constructor called!" << std::endl;
}
~MyClass() {
std::cout << "Destructor called!" << std::endl;
}
};
int main() {
MyClass* obj = new MyClass();
delete obj;
return 0;
}
Output:
Constructor called!
Destructor called!
In this example, we create a class called MyClass
. When we instantiate an object of this class using new
, the constructor is called, and we see the message “Constructor called!” in the output. Once we are done with the object, we use delete obj;
to free the memory. This action also calls the destructor, which is confirmed by the output “Destructor called!”. This demonstrates the importance of pairing new with delete to manage memory effectively.
Using the delete
Operator for Arrays
When dealing with arrays, you must use delete[] to ensure that all elements are properly deallocated. Let’s look at an example:
#include <iostream>
int main() {
int* arr = new int[5];
for (int i = 0; i < 5; ++i) {
arr[i] = i + 1;
}
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
delete[] arr;
return 0;
}
Output:
1 2 3 4 5
In this example, we allocate an array of integers using new int[5]
. After populating the array, we print its contents. Finally, we use delete[] arr;
to free the allocated memory. This is crucial because using delete instead of delete[] would lead to undefined behavior, as it would not properly call the destructors for each element in the array.
Use the delete
Operator to Free Elements in Vector
Using the delete
operator with dynamically allocated arrays is slightly different. Generally, new
and delete
operate on objects one at a time, but the arrays need multiple elements to be allocated with one call. Thus, the square bracket notation is utilized to specify the number of elements in the array, as demonstrated in the following example code.
Allocation like this returns a pointer to the first element, which can be dereferenced to access different members. Finally, when the array is not needed, it should be freed with a special delete
notation containing empty square brackets, which guarantees that every element has been deallocated.
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int SIZE = 10;
constexpr int MIN = 1;
constexpr int MAX = 1000;
void initPrintIntVector(int *arr, const int &size) {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int i = 0; i < size; ++i) {
arr[i] = distr(eng) % MAX;
cout << setw(3) << arr[i] << "; ";
}
cout << endl;
}
int main() {
int *arr1 = new int[SIZE];
initPrintIntVector(arr1, SIZE);
delete[] arr1;
return EXIT_SUCCESS;
}
Output:
311; 468; 678; 688; 468; 214; 487; 619; 464; 734;
266; 320; 571; 231; 195; 873; 645; 981; 261; 243;
Common Mistakes with the Delete Operator
When working with the delete operator, it’s easy to make mistakes that can lead to serious issues. Here are some common pitfalls to avoid:
-
Double Deletion: Attempting to delete the same pointer twice can cause your program to crash. Always set pointers to nullptr after deletion.
-
Using Delete on Non-Dynamically Allocated Memory: If you attempt to use delete on a variable that was not allocated with new, you will encounter undefined behavior.
-
Forgetting to Use Delete: Not using delete after allocating memory can lead to memory leaks. It’s essential to keep track of every allocation and ensure it has a corresponding deletion.
Here’s an example illustrating a mistake:
#include <iostream>
int main() {
int* ptr = new int(10);
delete ptr;
delete ptr; // Double deletion - undefined behavior
return 0;
}
Output:
Undefined behavior (may crash)
In this case, after deleting ptr
, trying to delete it again leads to undefined behavior. To avoid this, always set ptr
to nullptr after deletion.
Best Practices for Using the Delete Operator
To use the delete operator effectively, consider the following best practices:
-
Always Pair New with Delete: For every call to new, ensure there is a corresponding call to delete. This helps prevent memory leaks.
-
Use Smart Pointers: C++11 introduced smart pointers, such as
std::unique_ptr
andstd::shared_ptr
, which automatically manage memory. Using smart pointers can reduce the burden of manual memory management. -
Initialize Pointers: Always initialize your pointers. If a pointer is not initialized, using delete on it can lead to undefined behavior.
-
Set Pointers to Null After Deletion: After deleting a pointer, set it to nullptr to prevent accidental double deletion.
By following these practices, you can ensure that your C++ applications remain efficient and free of memory-related issues.
Conclusion
The delete operator is a fundamental aspect of memory management in C++. Understanding how to use it correctly is essential for writing robust and efficient code. By mastering the delete operator, along with its counterpart new, you’ll be well-equipped to handle dynamic memory allocation in your applications. Remember to avoid common pitfalls, adhere to best practices, and consider using smart pointers for a safer approach to memory management. With these tools in your arsenal, you can enhance the performance and reliability of your C++ programs.
FAQ
-
What is the difference between delete and delete[]?
delete is used for single objects, while delete[] is used for arrays allocated with new. -
What happens if I forget to use delete?
Failing to use delete can lead to memory leaks, causing your application to consume more memory than necessary. -
Can I use delete on a pointer that wasn’t allocated with new?
No, using delete on a pointer that wasn’t allocated with new leads to undefined behavior. -
How can I avoid memory leaks in C++?
Always pair every new with a delete, and consider using smart pointers for automatic memory management. -
Is it safe to delete a pointer multiple times?
No, deleting a pointer multiple times can cause undefined behavior. Always set the pointer to nullptr after deletion.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook