Default Constructor and Default Keyword in C++
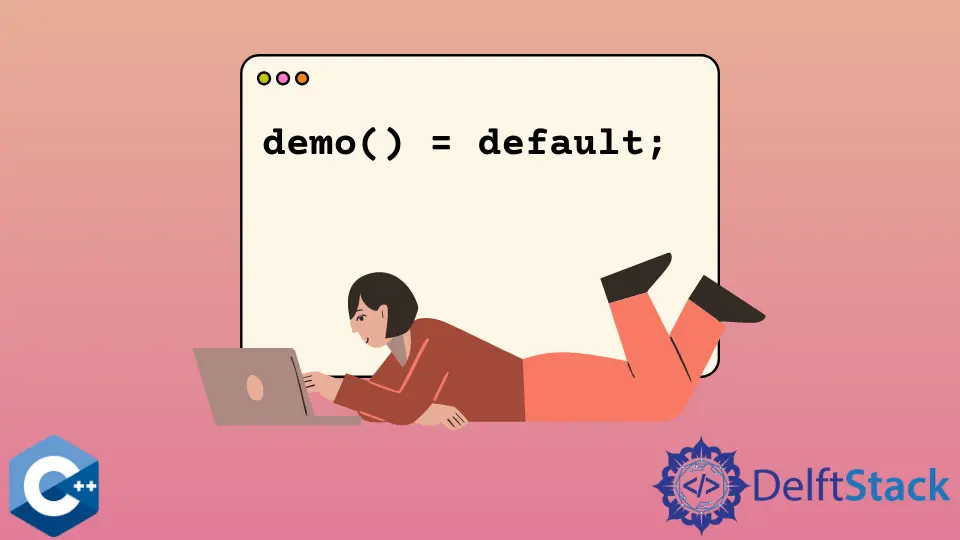
This article talks about default constructors in C++ and the newly introduced keyword default
.
First, let us learn about the default constructor in C++. The default constructor is a special kind of constructor that has no arguments and is used to set default values to the data members of a class.
Example:
class demo {
int age;
int id;
public:
demo() {
age = 10;
id = 1;
}
};
Default
Keyword and Defaulted Function in C++
C++ 11 version introduced a new form of function declaration way to explicitly declare the default functions with the help of the =default
specifier. We can explicitly declare a defaulted function by appending this at the end of the function declaration.
Making a function explicitly default has advantages as it forces the compiler to generate the default implementation. And in terms of efficiency, they are better than the manually implemented functions.
For example, when creating a parameterized constructor in a class
, the compiler doesn’t create or add a default constructor in the program. We can use the =default
to create a default constructor in those cases.
Example code:
#include <bits/stdc++.h>
using namespace std;
class demo {
public:
int x;
demo(int temp) // parameterized constructor
{
x = temp;
}
demo() = default;
};
int main() {
demo d; // uses default constructor
demo d2(200); // uses parameterized constructor
}
We can see that after the parameterized constructor, we have used the =default
specifier to force the compiler to create a default version of the constructor. And we can observe that when using =default
, we don’t have to specify the body of our constructor.
Another reason for using =default
than using the {}
(empty body) of function is that it enhances the readability of the code.
When dealing with the =default
specifier, one rule is that the function should have no default argument. Only special member functions such as default constructor, copy constructor, or destructor can be defaulted.
Example code:
#include <bits/stdc++.h>
using namespace std;
class demo {
public:
int add() = default;
demo(float, float) = default;
demo(int = 0) = default;
};
int main() {
demo d2(200, 300); // uses parameterized constructor
}
Output:
[Error] 'int demo::add()' cannot be defaulted
[Error] 'demo::demo(float, float)' cannot be defaulted
[Error] 'demo::demo(int)' cannot be defaulted
The reason above doesn’t work because the first function and the second function add()
and demo(float,float)
is not a special member function, and the third function demo(int = 0)
has some default argument. So these functions can’t be defaulted using =default
; hence we get the compile error.