How to Decompile a DLL Into C++ Source Code
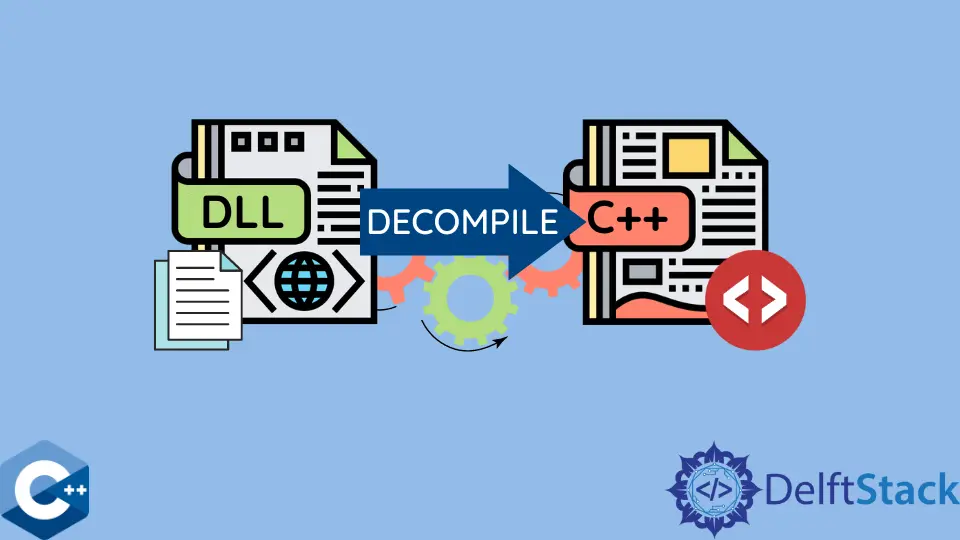
This article discusses the tools we can use to decompile a DLL
into C++ source code.
Brief Introduction of Decompiler
The decompiler is a type of reverse engineering tool. It is an essential tool for programmers because it lets them see how the program functions, which can help resolve software bugs or other problems.
A decompiler will work by taking the compiled code and analyzing it to extract information about the original program’s logic, data structures, and machine language instructions.
This information is then converted into a high-level programming language so the programmer can see how the original code was written. This process is called reverse engineering.
The advantage of decompiling is that we can do it on executable files, which are not human-readable. In addition, it means we can recover source code for programs after compilation.
The disadvantage is that the decompiled code may not compile due to compiler optimizations or machine language incompatibilities, and it may produce errors or warnings when compiled.
Tools to Decompile a DLL
A DLL
is a file containing executable instructions in a format specific to the operating system. Although there are many different formats for DLLs
, they all have one thing in common - they are machine code files.
The only way to decompile a DLL
into C++ source code is by using an assembler program. Assemblers are programs that translate machine code into assembly language, which is much easier for humans to read and understand.
Assembly language is not easy to write, but we can do it with the help of an assembler program. Let’s have the most straightforward example code to decompile a DLL
.
Example Code:
#include "stdafx.h"
#include "windows.h"
char *x = "hello";
int APIENTRY WinMain(DEMO demo, DEMO dPrevEMO, int nCmdShow) {
x = (char *)0x50000;
return 0;
}
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook