How to Deallocate a std::vector Object in C++
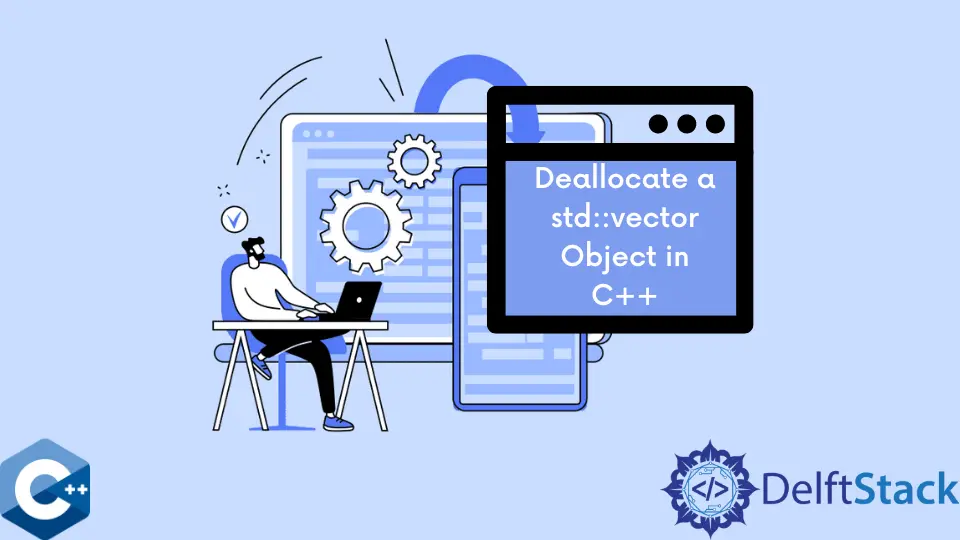
This article will explain how we can deallocate the std::vector
objects in C++/C. First, we will see the self-coded ways of deallocation, and after that, we will examine how the std::vector
objects are dynamically deallocated in C++.
Deallocate Objects in C++
When a class object in C++ is created, the special member function known as constructor
is automatically called, which initializes the object with some default values. Similarly, a special member function destructor
is automatically called when the created object goes out of scope.
This destructor
function releases all the memory occupied by the created object. This process of releasing the memory occupied by the object is known as deallocation.
the std::vector
Object
In C++, std
is a namespace, and the ::
operator is a scope operator. This scope operator tells the compiler which class/namespace to look for the identifier/class.
If the expression is like std::cout
, it means to look for the cout
identifier in the std
namespace. Similarly, std::vector
means to look for a vector class in the std
namespace.
For deallocation, first, we need to create an object of the vector
class. For that, we can use the below code with GCC compiler C++11:
#include <iostream>
#include <vector>
int main() {
// Create a vector containing integers
std::vector<int> v = {7, 5, 16, 8};
}
The std
namespace contains multiple C++ libraries in the above code. We first explicitly added the vector
library and used it to initialize the vector with the std::vector<int>
type.
Self-Coded Ways of Vectors Deallocation in C++
In the below code, we explicitly wrote the commands to delete the vector. This only applies when you use the new
keyword to create the vectors.
The new
operator signals an inquiry for memory allocation on the Free Store. A new
operator will allocate the memory if there is enough space, initialize it, and then provide the location of the newly created and initialized memory to the pointer variable.
In the code below, we first initialized the vector pointer, then checked whether it was NULL
or not. After doing our required computation, we ensured through the assert
statement that the vector pointer is not empty.
Then we removed the vector and its occupied space using the delete
statement.
#include <cassert>
#include <iostream>
#include <vector>
using namespace std;
int main() {
std::vector<int>* vec = new std::vector<int>;
\\*** assert(vec != NULL);
delete vec;
return 0;
}
Using the code below, we made the allocated memory free by vec
by assigning an empty vector after doing the necessary computations. The command std::vector<int>()
creates an empty vector with zero bytes.
The last statement checks the occupied memory by vec
in bytes.
std::vector<int> vec;
\\***
\\*** vec = std::vector<int>();
std::cout << sizeof(decltype(vec.back())) * vec.capacity() << std::endl;
Dynamic Ways of Vectors Deallocation in C++
In C++, when you define a std::vector
object inside a function (i.e., inside {....}
), the vector class constructor is automatically called, which initializes the vector object. When the function execution ends (i.e., it reaches the closing bracket }
), the destructor is automatically called.
For example,
void Foo() {
std::vector<int> v;
...
}
C++ guarantees that the destructor of v
will be called when the method executes. The destructor of std::vector
will ensure any memory it allocated is freed.