C++ Cube Root
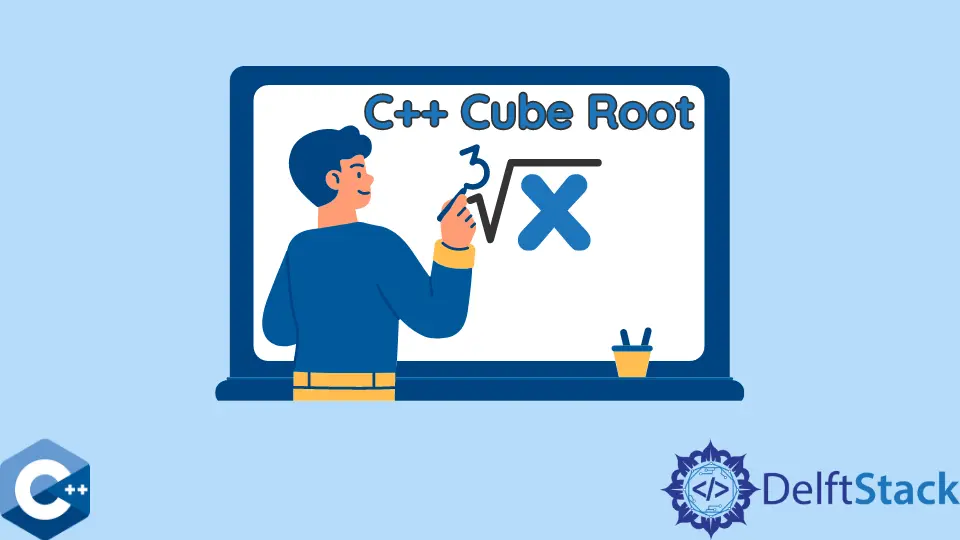
This tutorial will discuss computing the cube root of a number using the pow()
or cbrt()
function of the cmath
library in C++.
Cube Root Using the cbrt()
Function in C++
We can find the cube root of a number using the cbrt()
function of the cmath
library of C++. The basic syntax of the function is given below.
output = cbrt(input);
The input of the cbrt()
function should be several data type int
, float
, double
, or long
. The input should not be a string or character value.
The cbrt()
function returns the cube root of the input number in the double
data type, but we can also store the result in other data types like float
or long
.
For example, let’s take the cube root of a number using the cbrt()
function. See the code below.
#include <cmath>
#include <iostream>
int main() {
double number = 3;
double cubeRoot = std::cbrt(number);
std::cout << cubeRoot;
return 0;
}
Output:
1.44225
We have included two libraries, iostream
and cmath
, in the above code. The cout
function in the above code belongs to the iostream
library, which displays the output.
The cbrt()
function belongs to the cmath
library and is used to take the cube root of the given number. We have used the double
data type to store the given number and the output of the cbrt
function.
The cbrt()
function returns the result in double
data type but we can also use the cbrtf()
and cbrtl()
function to take the cube root of a number. The cbrtf()
function returns the result in floating point data type and the cbrtl()
function returns the data type in long
data type.
The difference between these functions is the size of the output result. For example, the size of a float
variable is 4 bytes, and the size of a double
and long
variable is 8 bytes.
So, a long
or double
variable will take more space in the memory than the float
variable, but it can be used to take the cube root of very long numbers so that the result will be accurate.
Cube Root Using the pow()
Function in C++
We can find the cube root of a number using the pow()
function of the cmath
library of C++. The pow()
function takes the power of a given number.
We know that the cube root can be expressed as an exponent with the value 1/3
, and to take the cube root of a number, we have to pass the 1/3
exponent as the power inside the pow()
function. The basic syntax of the function is given below.
output = pow(input, power);
The input number and the power of the pow()
function should be several data types float
, double
, or long
. The input and the power should not be a string or character value.
The pow()
function returns the result in the same data type as the input number and power. For example, let’s take the cube root of a number using the pow()
function.
See the code below.
#include <cmath>
#include <iostream>
int main() {
double number = 3;
double power = 1 / 3.;
double cubeRoot = std::pow(number, power);
std::cout << cubeRoot;
return 0;
}
Output:
1.44225
The result of the pow()
function is the same as compared with the cbrt()
function. The power function will not work if an input number is negative and will give unexpected results.
The pow()
function will give an error if the number and the power are zero, and it will also give an error if the result is too large or too small.