How to Write to File in C++
-
Use
fstream
and<<
Operator to Write to File -
Use
fstream
andwrite
Function to Write to File -
Use the
fwrite
Function to Write to File
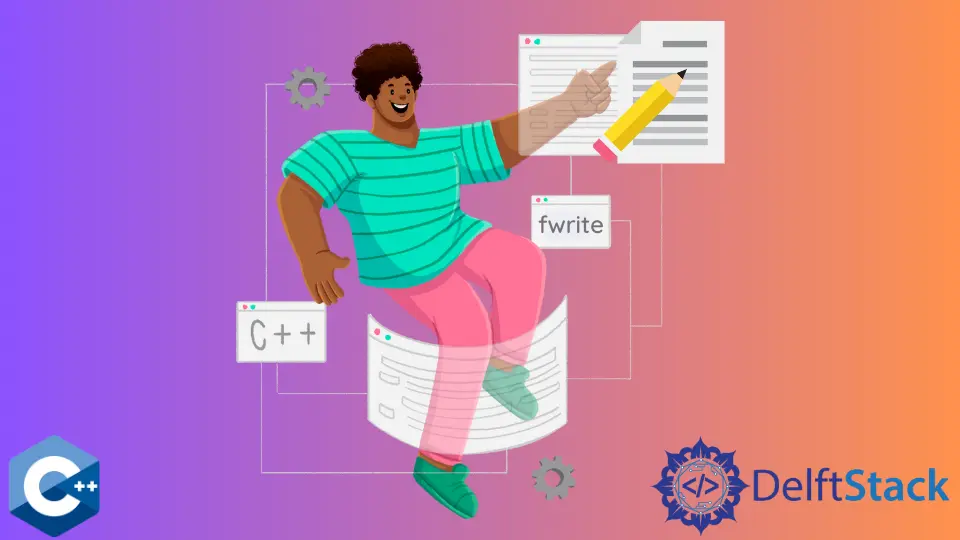
This article will explain several methods of how to write to a file in C++.
Use fstream
and <<
Operator to Write to File
File I/O
in C++ is handled using the fstream
class, which provides multiple built-in methods for stream manipulation and positioning. Once fstream
object is declared, we can call the open
function, passing the name of the file and the mode in which to open the file as arguments.
When writing to a file, we specify std::ios_base::out
mode, which corresponds to open for writing state. Next, we check if the file has been successfully associated with the stream object, that is, the call to the is_open
method in the if
condition. At this point, the file is ready to take input using the <<
operator and process the output as needed.
Notice that, else
block can be utilized for error logging purposes, or we can implement error handling routines for better fault-tolerant program execution.
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string filename("tmp.txt");
fstream file_out;
file_out.open(filename, std::ios_base::out);
if (!file_out.is_open()) {
cout << "failed to open " << filename << '\n';
} else {
file_out << "Some random text to write." << endl;
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Use fstream
and write
Function to Write to File
Another method to write to file is the built-in write
function that can be called from the fstream
object. The write
function takes a character string pointer and the size of the data stored at that address. As a result, given data is inserted into the file associated with the calling stream object.
In the following code sample, we demonstrate the minimal error reporting by printing the status of the open
function call, but in real-world applications, it would require more robust exception handling, which is documented in the manual page.
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename2("tmp2.txt");
fstream outfile;
outfile.open(filename2, std::ios_base::out);
if (!outfile.is_open()) {
cout << "failed to open " << filename2 << '\n';
} else {
outfile.write(text.data(), text.size());
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Use the fwrite
Function to Write to File
Alternatively, we can use fwrite
function from the C-style file I/O
to write to the file. fwrite
obtains the data from the void
pointer and the programmer is responsible for passing the number of items stored at that address as well as the size of each item. The function needs the file stream to be of type FILE*
and that can be obtained by calling the fopen
function.
Notice that fwrite
returns the number of items written to a file when the operation is successful.
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename3("tmp3.txt");
FILE *o_file = fopen(filename3.c_str(), "w+");
if (o_file) {
fwrite(text.c_str(), 1, text.size(), o_file);
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
Output:
Done Writing!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook