Warning: Cast From Pointer to Integer of Different Size in C++
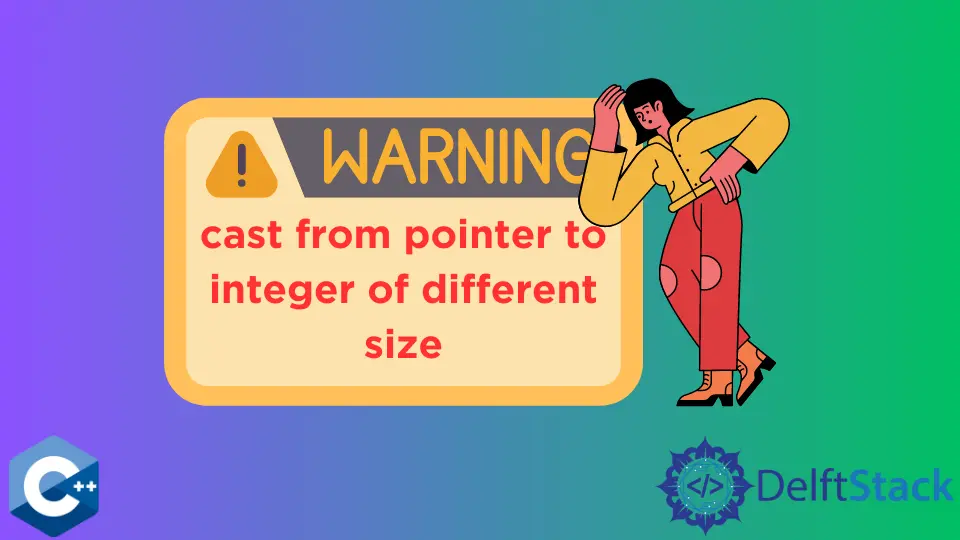
This quick article is about the solution to the warning of cast from pointer to integer of different size
in C++. The warning is often displayed as an error on different compilers.
Warning: Cast From Pointer to Integer of Different Size in C++
The compiler issues warnings and errors to inform the programmer that something is wrong with the code. The errors forbid the code from executing, whereas warnings allow the execution of the code, but sometimes this execution can cause some memory handling issues.
Therefore, cleaning your code from all the warnings and errors is advised before executing it.
The warning of cast from pointer to integer of different size
occurs when you try to save the value of an integer to a pointer variable, as demonstrated in the code below.
int var = 897;
int* ptr;
ptr = var; // this conversion is wrong
The above code generates a warning or an error, depending on the compiler type.
Output:
This type of conversion is wrong for some reasons:
-
ptr
is a pointer-type variable used to save the addresses. This means it can point to some memory location, and that memory location can have some value saved.Therefore, it cannot save
int
-type data in itself. We can save the address ofvar
in this pointer variable, not the value. -
Secondly, the size of both the variables is different, so there is a chance of data loss in case of this conversion.
We can do the following to solve this error.
int main() {
int var = 897;
int* ptr;
ptr = &var;
cout << "ptr contains: " << *ptr << endl;
cout << "var contains: " << var << endl;
}
This will give the following output.