Void Pointer in C++
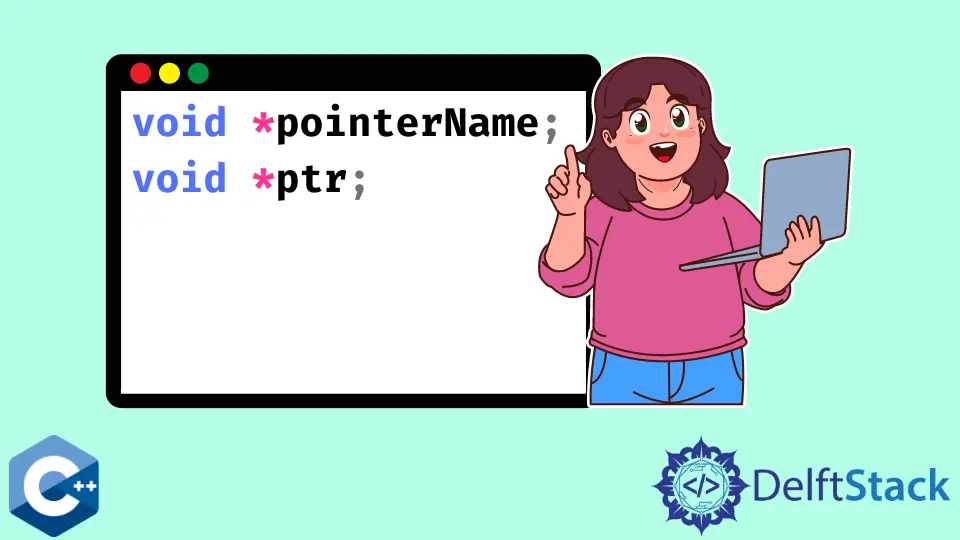
This article will discuss the uses of void*
pointers in C++. An example code is also provided to demonstrate its implementation.
Implement the void*
Pointer in C++
We have studied that the pointer should be of the same data type to which it is pointing, as specified in the pointer declaration. If we declare a float pointer, it cannot point to any other data type such as int, long, or string; it can only point to a float type variable.
C/C++ has developed a solution to overcome this problem - the void*
pointer. A void*
pointer is a general-purpose pointer that can point to any data type without any static
pointer definition.
You can assign the address of any data type to a void*
pointer. Similarly, the void*
pointer can be assigned to any data type of pointers without explicitly typecasting them.
Syntax:
void *pointerName;
void *ptr;
There are two main disadvantages of using void*
pointers in C++:
- Due to the concrete size, performing pointer arithmetic is not possible with the
void*
pointer in C++. - You cannot dereference a
void*
pointer.
Size of the void*
Pointer in C++
The size of the void*
pointer is similar to the size of the pointer of the character type in C++. The pointer stores the memory address that points to some data in the program.
This pointer in C++ depends on your computer platform. On a 32-bit platform, you need 4 bytes or 32 bits to store the memory address data.
The sizeof()
function can identify the size of the pointer.
Syntax:
void *ptr = NULL; // void pointer
sizeof(ptr);
Example code:
#include
#include
using namespace std;
int main() {
void *ptr = NULL; // void pointer
int *p = NULL; // integer pointer
char *cp = NULL; // character pointer
float *fp = NULL; // float pointer
// size of pointers
cout
Reason to Use void*
Pointers in C++ Programs
We use the void*
pointer in C++ to overcome the issues of assigning separate values to different data types in a program. The void*
pointer in C++ can point to any data type and typecast that data type without explicitly typecasting.
It does not have any return value. A void*
pointer can point to an int, float, or char and typecasts to that specific data type.
It helps developers in writing code and reducing the complications of a program.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn