How to Create Vector of Pointers in C++
-
Use
[]
Notation to Create Vector of Pointers in C++ -
Use the
new
Operator to Create Vector of Pointers on Dynamic Memory in C++ -
Use the
std::vector
Container to Create Vector of Pointers in C++
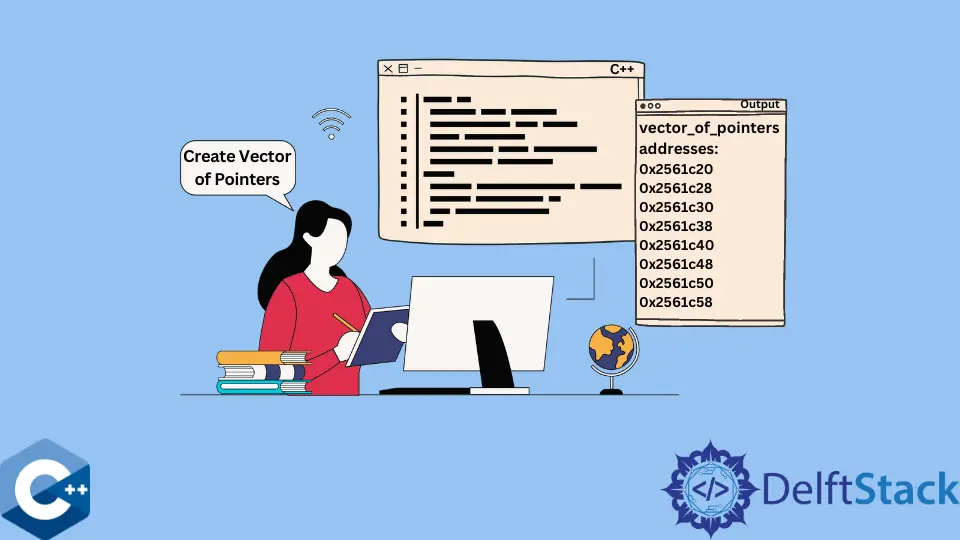
This article will explain several methods of how to create a vector of pointers in C++.
Use []
Notation to Create Vector of Pointers in C++
Since the pointer types can be modified easily, we will use int *
in the following examples to declare a vector of pointers. Alternatively, we can use the void *
pointer if you require a generic address type to store opaque data structures or, on the contrary, use a pointer to a custom defined class.
This solution uses a C-style array notation - []
that declares a fixed-length array. It is similar to the regular array declaration, but in this case, we are interested in accessing each element’s addresses. We are using the &
(address of) operator to access pointers in the vector and print them out to the console. Note that these memory addresses are located on the stack memory.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
constexpr int WIDTH = 8;
int main() {
int *pointers_vec[WIDTH];
cout << "pointers_vec addresses:" << endl;
for (auto &i : pointers_vec) {
cout << &i << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
pointers_vec addresses:
0x7ffecd7a00d0
0x7ffecd7a00d8
0x7ffecd7a00e0
0x7ffecd7a00e8
0x7ffecd7a00f0
0x7ffecd7a00f8
0x7ffecd7a0100
0x7ffecd7a0108
Use the new
Operator to Create Vector of Pointers on Dynamic Memory in C++
On the other hand, we can utilize the new
operator to create a vector of pointers dynamically allocated on the heap.
This solution requires the programmer to free up the memory before the program exits; otherwise, the code will suffer from the memory leak, which can be a massive problem in long-running applications and resource-constrained hardware environments. Note that we use the delete []
notation to clean up each location in a dynamically allocated vector.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
constexpr int WIDTH = 8;
int main() {
int *vector_of_pointers = new int[WIDTH];
cout << "vector_of_pointers addresses:" << endl;
for (int i = 0; i < WIDTH; ++i) {
cout << &vector_of_pointers[i] << endl;
}
cout << endl;
delete[] vector_of_pointers;
return EXIT_SUCCESS;
}
Output:
vector_of_pointers addresses:
0x2561c20
0x2561c28
0x2561c30
0x2561c38
0x2561c40
0x2561c48
0x2561c50
0x2561c58
Use the std::vector
Container to Create Vector of Pointers in C++
std::vector
offers rich functionality to allocate a vector of pointers and manipulate the vector with multiple built-in functions. This method provides a more flexible interface for new element creation during the run-time. Notice that we initialized the vector elements with the nullptr
value, as shown in the following example.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
constexpr int WIDTH = 8;
int main() {
vector<int *> vector_p(WIDTH, nullptr);
cout << "vector_p addresses:" << endl;
for (int i = 0; i < WIDTH; ++i) {
cout << &vector_p[i] << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
vector_p addresses:
0x1255c20
0x1255c28
0x1255c30
0x1255c38
0x1255c40
0x1255c48
0x1255c50
0x1255c58
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook