Difference Between Struct and Typedef Struct in C++
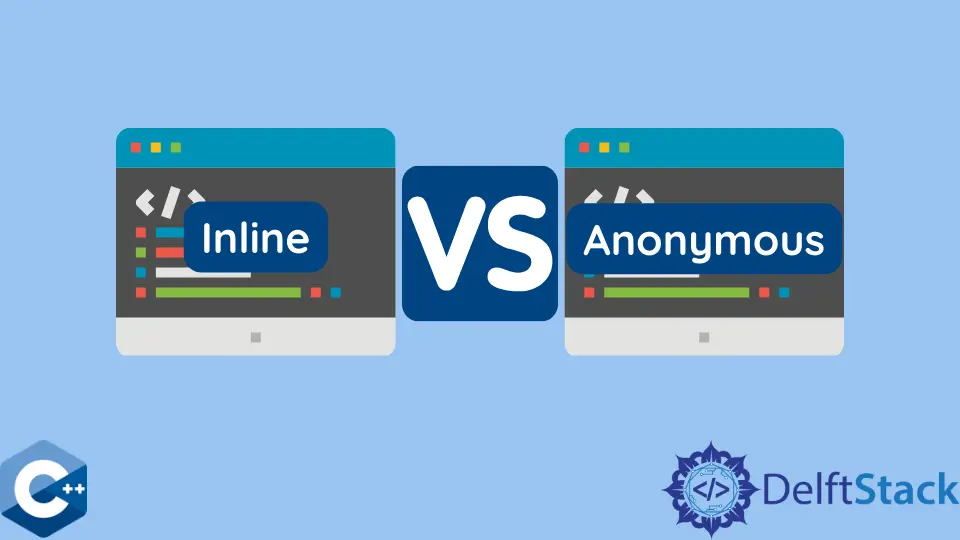
This small article will discuss the keyword typedef
in C++. We will also discuss the difference between a simple struct and a typedef
struct in C++.
the typedef
Keyword in C/C++
The typedef
is a keyword in C/C++ used for giving new names or aliases to data types. These data types can be primitive (e.g., int, float, etc.) or non-primitive or user-defined defined data types (e.g., a class
, a struct
, or a union
).
The typedef
keyword is used mainly with user-defined data types that are somewhat complicated to use in the program or have names poorly related to the program context.
The syntax for declaring typedef
for the primitive int data type is as follows:
typedef int negative_int;
Now, whenever we must declare an int variable in the program, we can do that with the name negative_int
like this:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to the typedef tutorial" << endl;
typedef int negative_int; // typedef keyword use
negative_int num = -7;
cout << "The number is: " << num << endl;
return 0;
}
The output of this code will be:
Welcome to the typedef tutorial
The number is: -7
Difference Between struct
and typedef struct
in C++
There is no distinction between struct
and typedef struct
in C++ since as long as another declaration with the same name does not obscure the name, all structs
, unions
, enums
, and class
declarations behave as these are implicitly typedef
‘ed.
The C language used typedef struct
because, in C, whenever you need to create an object of struct
, it is compulsory to use the struct
keyword every time. Therefore, typedef
is helpful where you can use struct
only by its name.