Time(NULL) Function in C++
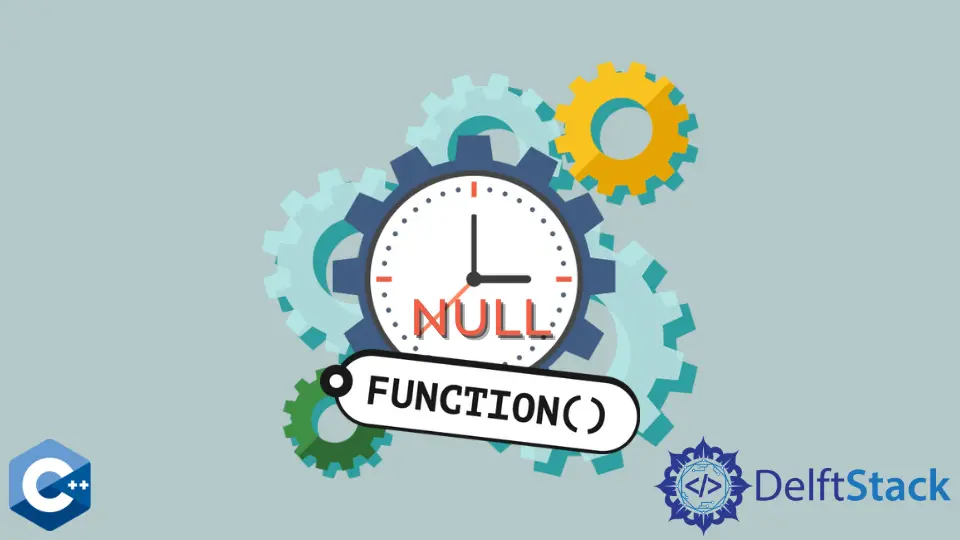
This article will discuss the time(NULL)
function in C++.
the time(NULL)
Function in C++
The time()
function with a parameter NULL, time(NULL)
, returns the current calendar time in seconds since 1 January 1970. Null is a built-in constant whose value is 0
and a pointer similar to 0
unless the CPU supports a special bit pattern for a Null pointer.
Suppose you pass a pointer to a time_t
variable; that variable will point to the current time. time_t
is a data type in the ISO C++ library defined for storing and utilizing system time values.
Such value types are returned from the standard time()
library function. It is defined in the time.h
header file and is an unsigned long integer, and its size is 8 bytes.
Example code:
#include <time.h>
#include <iostream>
using namespace std;
int main() {
time_t seconds;
seconds = time(NULL);
cout << "Time in seconds is = " << seconds << endl;
}
Output:
Time in seconds is = 1650710906
We have defined a variable seconds
of the time_t
data type and initialized it to the return value of the function time(NULL)
. This function returned time in seconds from 1 January 1970
, and we printed out the result at the end.
Now, reading time in seconds may not be conveniently understandable for humans, so there should be some mechanism to convert time in seconds into an understandable format. And thanks to the C++ libraries, we have a solution to it as follows;
#include <time.h>
#include <iostream>
using namespace std;
int main() {
time_t seconds;
seconds = time(NULL);
struct tm* local_time = localtime(&seconds);
cout << "Time in seconds " << seconds << endl;
cout << "local time " << asctime(local_time);
}
Output:
Time in seconds 1650712161
local time Sat Apr 23 16:09:21 2022
struct tm
is a built-in structure in the time.h
header file in C/C++ language, and every object contains the date and time on the machine. We can utilize these members of the tm
structure to customize our code the way we want.
struct tm {
int tm_sec; // seconds, ranges from 0 to 59
int tm_min; // minutes, ranges from 0 to 59
int tm_hour; // hours, ranges from 0 to 23
int tm_mday; // day of the month, ranges from 1 to 31
int tm_mon; // month, ranges from 0 to 11
int tm_year; // The number of years since 1900
int tm_wday; // day of the week, ranges from 0 to 6
int tm_yday; // day in the year, ranges from 0 to 365
int tm_isdst; // daylight saving time
};
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn