How to Throw Exception in C++
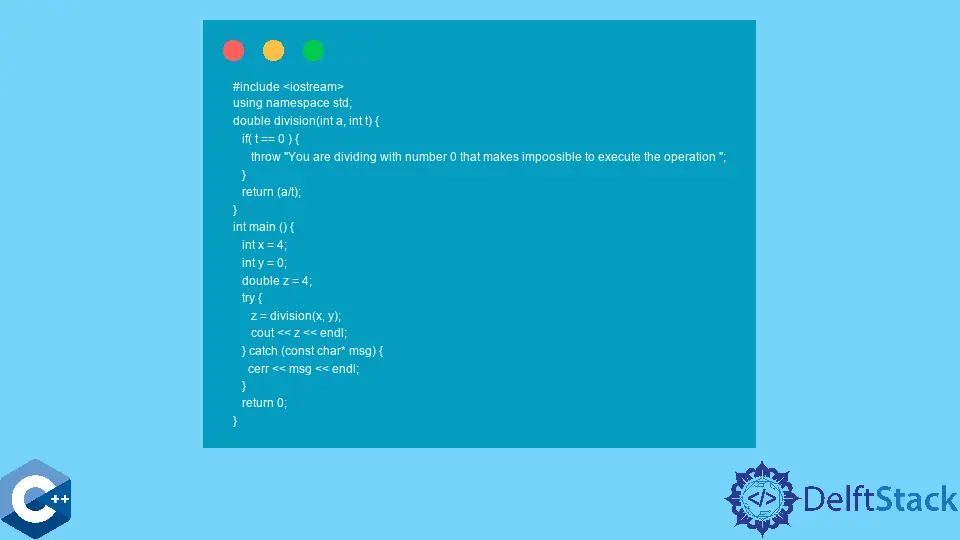
C++ throw
exception is a powerful feature of C++ that can be used to handle errors and unexpected events. It is mainly used to terminate the program’s execution or transfer control to a different part of the program.
Throw Exception in C++
A C++ exception is generated by an exceptional situation, such as an invalid argument, division by zero, etc. The compiler can create an exception for every error it detects at compile time.
However, programmers can also make exceptions by using the keyword throw
in their code.
Apart from the throw
, we also have a catch
and try
keyword.
Catch
− A code catches an exception using an exception handler at the point in the code where the problem has to be addressed. Thecatch
keyword indicates that an exception has been caught.try
− Atry
block indicates a section of code for which certain exceptions will be triggered. One or morecatch
blocks come after it.
A method captures an exception by combining the try
and catch
keywords, assuming a block would raise an exception. A try/catch
block encapsulates code that might throw an error.
Purpose of Using Exceptions in C++
Exceptions are a way of handling errors in C++. They are used to make the code more efficient, maintainable, and robust.
Exceptions allow programmers to handle errors more systematically than simply returning error codes or status codes. The exceptions mechanism is designed to be flexible and can be used for handling any error that might occur during the execution of a program.
Some common uses for exceptions include:
- Detecting invalid input parameters
- Detecting invalid data structures
- Handling I/O errors
- Handling memory allocation failures and so on
Use C++ throw
Exception
This section provides a guide on using C++ throw
exceptions.
- The first step to using C++
throw
exception is to create a class. - The second step is to create a constructor for the class. This will be used to initialize the variables in the class, and it will also be used when creating objects from this class.
- Create an object of this class by calling the constructor and then calling a method. If there is an error in the code, this method will throw an exception and print out some information.
Let’s discuss an example by using the steps mentioned above.
#include <iostream>
using namespace std;
double division(int a, int t) {
if (t == 0) {
throw "You are dividing with number 0 that makes impoosible to execute the operation ";
}
return (a / t);
}
int main() {
int x = 4;
int y = 0;
double z = 4;
try {
z = division(x, y);
cout << z << endl;
} catch (const char* msg) {
cerr << msg << endl;
}
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook