C++ Struct Default Values Initialization
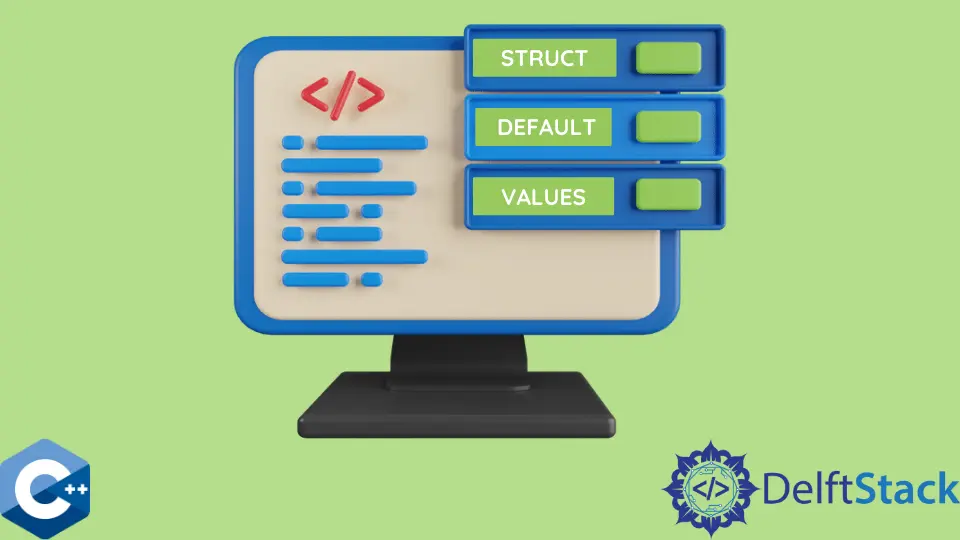
This article will cover how to initialize default values in a struct
in C++.
Initialize Default Values in a struct
in C++
Mainly, there are two methods to initialize the default values; the first is using the constructor and the second without using the constructor. The latest and most updated method is without using the constructor, and in this article, we will also focus on this method.
But, firstly, it’s important to understand the concept of the struct
.
The struct
is a data type that can store related data in a single variable. struct
is most commonly used to implement an abstract data type and can be either rigid or flexible.
A struct
is not an object, so it does not have memory management associated with it and cannot be created as an instance of a class. It also cannot inherit from another struct
or class.
A struct
is often used to store simple types, such as integers or floating point numbers, but they can also contain more complex types such as arrays, strings, and even other structs.
Let’s discuss the steps to initialize the default values in a struct in C++.
Steps to Initialize Default Values in a struct
in C++
The steps to initialize default values in a struct
in C++ using the Brace-or-Equal-Initializers are as follows:
-
Start with a code block and type the keyword
struct
and a name for your data type. -
Add brackets after the name of your data type and add an opening curly brace (
{
). -
Inside the curly braces, add a comma-separated list of variables you want to initialize with default values.
-
Add an equal sign (
=
), followed by the value you want to initialize each variable. -
Add a closing curly brace (
}
) -
At the end of your code block, add one more comma (
,
) followed by a semicolon (;
).
Let’s have an example.
#include <iostream>
using namespace std;
struct hello {
bool x = true;
bool y = true;
bool z = false;
bool a = false;
bool b = true;
bool c = false;
} demo;
int main() {
cout << demo.x << demo.y << demo.z << demo.a << demo.b << demo.c << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook