Struct Constructors in C++
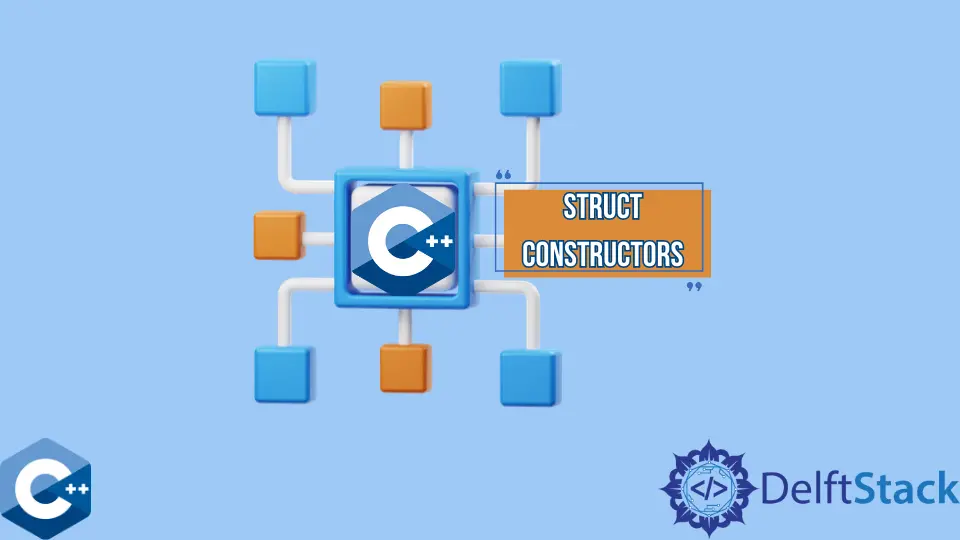
This small tutorial will discuss the use of struct
and adding constructors to it using C++.
Introduction to struct
in C++
struct
stands for Structure and is the user-defined data type that combines some primitive type variables. These variables are mixed to form a new unit.
It has a user-defined name as well. The syntax to declare a struct
is as follows:
struct structName {
data - type var_1;
data - type var_2;
........date - type var_N
};
The above syntax suggests that the struct
has a user-defined name and some set of variables. These variables can be of some primitive datatypes and also some other user-defined class
or struct
variables.
A struct
can have all or any data members, member functions, constructors, destructors, operators, events, and any nested datatypes. After the struct
is declared, its object can be made with or without the new
keyword like this:
structName objectName = new structName();
OR structName objectName;
The difference is that if we initialize the object with new
, then the default constructor is called, and all the data members are assigned some values, and if we don’t use new
, then the data members remain unassigned.
struct
Constructors in C++
Constructors are the member functions called implicitly when the object is created using the new
keyword. These member functions are used to initialize the value to data members of the struct
.
Moreover, we can have a default and a parameterized constructor in a struct
. The syntax of declaring constructor is as follows:
struct structName {
datatype var1;
structName() { var1 = [some - value]; }
};
The above pseudocode shows the syntax for declaring a default constructor. The actual code is demonstrated in the following example:
struct Square {
float sides;
Square() { sides = 0; }
Square(int s) { sides = s; }
};
In the code above, we have declared two constructors, one is the default, and the other is parameterized. To create the object of struct
:
int main() {
Square s1 = new Square(); // default constructor called
Square s2 = new Square(5); // parameterized constructor called
Square s3; // No constructor called
}