C++ std::bad_alloc Exception
- What is std::bad_alloc?
- Causes of std::bad_alloc
- Handling std::bad_alloc Exception in C++
- Best Practices for Memory Management
- Conclusion
- FAQ
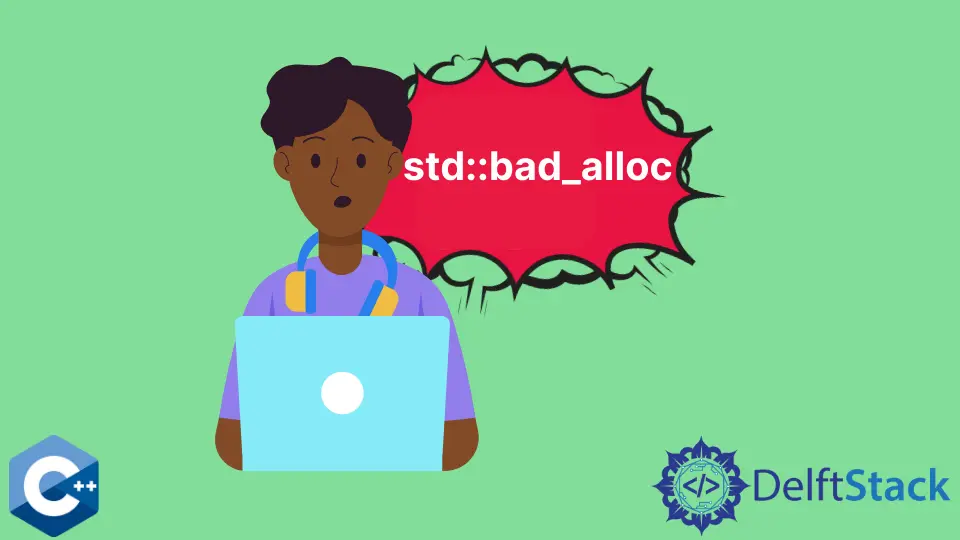
When programming in C++, developers often encounter various exceptions that can disrupt the flow of their code. One such exception is the std::bad_alloc
, which is thrown when the system fails to allocate memory dynamically. This can happen for several reasons, including insufficient memory or fragmentation. Understanding how to handle this exception is crucial for maintaining robust and efficient applications.
In this article, we will delve into the std::bad_alloc
exception, explore its causes, and discuss effective solutions to manage it. Let’s ensure your C++ applications are resilient and can handle memory allocation issues gracefully.
What is std::bad_alloc?
The std::bad_alloc
exception is part of the C++ Standard Library and is included in the <new>
header. It is derived from the std::exception
class and is specifically designed to signal memory allocation failures. When you attempt to allocate memory using operators like new
or functions like malloc
, and the allocation fails, the C++ runtime will throw a std::bad_alloc
exception. This exception allows developers to catch and handle memory allocation errors, rather than allowing the program to crash unexpectedly.
Causes of std::bad_alloc
Several factors can lead to a std::bad_alloc
exception. Here are some common causes:
-
Insufficient Memory: The most straightforward reason is that your program is trying to allocate more memory than is available on the system.
-
Memory Fragmentation: Even if there is enough total free memory, it may be fragmented into smaller blocks that cannot satisfy the allocation request.
-
Resource Limits: On some systems, there are limits imposed on the amount of memory a single process can allocate.
Understanding these causes can help you anticipate potential issues and implement strategies to mitigate them.
Handling std::bad_alloc Exception in C++
When dealing with std::bad_alloc
, it’s essential to implement proper exception handling in your code. Here’s how you can do it:
Using try-catch Blocks
A common way to handle exceptions in C++ is through the use of try
and catch
blocks. This allows you to catch the std::bad_alloc
exception and take appropriate action, such as logging the error or attempting to free up memory. Here’s an example:
#include <iostream>
#include <new>
int main() {
try {
int* arr = new int[100000000000]; // Attempting to allocate a large array
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
return 0;
}
In this code, we attempt to allocate a massive array. If the allocation fails, the std::bad_alloc
exception is caught, and an error message is printed to the standard error stream. This approach prevents the program from crashing and allows for graceful error handling.
Output:
Memory allocation failed: std::bad_alloc
Implementing exception handling using try-catch
blocks not only helps in managing memory allocation failures but also improves the overall reliability of your application. By catching exceptions, you can provide users with meaningful feedback and potentially recover from errors without terminating the program.
Best Practices for Memory Management
To minimize the chances of encountering a std::bad_alloc
exception, consider following these best practices for memory management:
1. Use Smart Pointers
Smart pointers, such as std::unique_ptr
and std::shared_ptr
, help manage memory automatically. They ensure that memory is freed when it is no longer needed, reducing the likelihood of memory leaks and fragmentation.
2. Optimize Memory Usage
Review your application’s memory usage patterns. Avoid allocating large chunks of memory at once and instead consider breaking down allocations into smaller, manageable sizes.
3. Monitor Memory Usage
Utilize profiling tools to monitor your application’s memory usage. This can help identify memory leaks or areas where memory usage can be optimized.
4. Implement Error Handling
Always implement error handling for memory allocation. Using try-catch
blocks, as shown earlier, ensures that your application can react appropriately when memory allocation fails.
By following these best practices, you can significantly reduce the risk of encountering std::bad_alloc
exceptions and improve the overall robustness of your C++ applications.
Conclusion
The std::bad_alloc
exception is a critical aspect of C++ programming that every developer should understand. By recognizing its causes and implementing effective handling strategies, you can ensure your applications remain stable even in the face of memory allocation failures. Remember to utilize best practices for memory management to minimize the chances of encountering this exception in the first place. With a solid understanding of std::bad_alloc
, you’re better equipped to build resilient and efficient C++ applications.
FAQ
-
What does std::bad_alloc indicate in C++?
std::bad_alloc indicates a failure to allocate memory dynamically in C++. -
How can I prevent std::bad_alloc exceptions?
You can prevent std::bad_alloc exceptions by using smart pointers, optimizing memory usage, and implementing error handling.
-
Where is std::bad_alloc defined?
std::bad_alloc is defined in theheader file of the C++ Standard Library. -
Can I catch std::bad_alloc exceptions?
Yes, you can catch std::bad_alloc exceptions using try-catch blocks in your C++ code. -
What should I do if I encounter a std::bad_alloc exception?
If you encounter a std::bad_alloc exception, you should log the error, free up memory if possible, and consider optimizing your memory usage patterns.