Static Function in C++
- What is a Static Function?
- How to Define and Use Static Member Functions
- Benefits of Using Static Member Functions
- Common Use Cases for Static Member Functions
- Conclusion
- FAQ
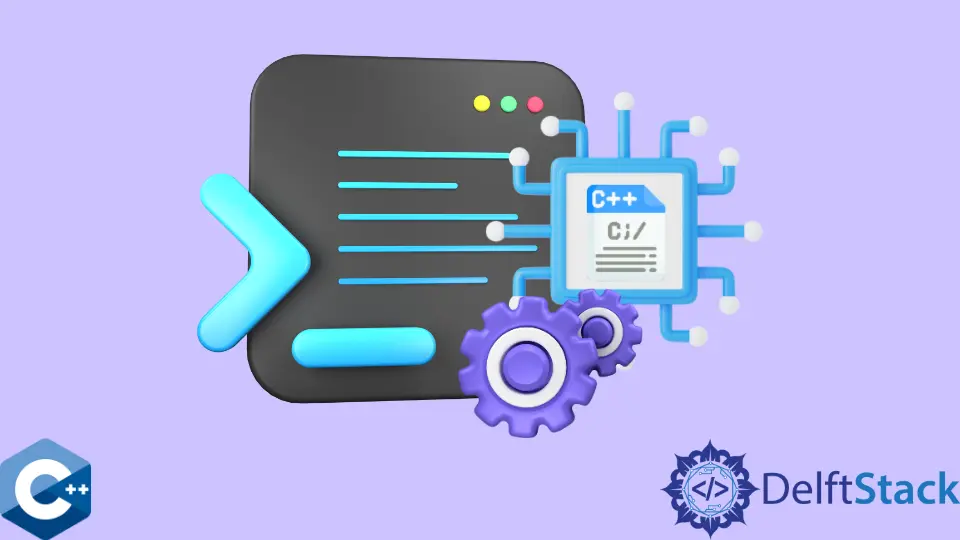
In the world of C++, understanding static member functions is essential for effective programming. Static member functions are unique because they belong to the class itself rather than any particular object. This means you can call these functions without creating an instance of the class. This feature is particularly useful for utility functions that don’t require access to instance-specific data.
In this article, we will explore how to utilize static member functions in C++, providing clear examples and explanations. Whether you’re a beginner or looking to refine your skills, this guide aims to enhance your knowledge of static functions in C++.
What is a Static Function?
Static functions in C++ are defined using the static
keyword. When a member function is declared static, it can be called on the class itself, not on instances of the class. This feature allows you to perform operations that are not dependent on object-specific data. For example, static functions can be used to maintain a count of instances created or to provide utility functions that assist in class-related tasks.
Here’s a simple example to illustrate the concept of static functions:
#include <iostream>
using namespace std;
class Example {
public:
static int count;
Example() {
count++;
}
static void displayCount() {
cout << "Count of instances: " << count << endl;
}
};
int Example::count = 0;
int main() {
Example obj1;
Example obj2;
Example::displayCount();
return 0;
}
Output:
Count of instances: 2
In this code, we have a class Example
that contains a static member count
, which keeps track of how many instances of the class have been created. The static member function displayCount
can be called without needing an object, showcasing its utility in providing class-level information.
How to Define and Use Static Member Functions
Defining static member functions in C++ is straightforward. You simply declare the function in the class with the static
keyword and define it outside the class. The key point to remember is that static member functions can only access static data members or other static member functions. They cannot access non-static members directly since they do not operate on a specific instance of the class.
Here’s an example that demonstrates this concept:
#include <iostream>
using namespace std;
class Math {
public:
static int add(int a, int b) {
return a + b;
}
static int multiply(int a, int b) {
return a * b;
}
};
int main() {
int sum = Math::add(5, 10);
int product = Math::multiply(5, 10);
cout << "Sum: " << sum << endl;
cout << "Product: " << product << endl;
return 0;
}
Output:
Sum: 15
Product: 50
In this example, the Math
class contains two static functions: add
and multiply
. These functions can be called directly using the class name, demonstrating how static functions can serve as utility functions without the need for an object. This makes your code cleaner and more efficient, especially when the functions do not rely on instance data.
Benefits of Using Static Member Functions
Using static member functions in C++ offers several advantages. Firstly, they promote better organization of code by grouping related functions within a class, even if those functions do not operate on instance data. This helps in maintaining a clean and logical structure in your codebase.
Secondly, static member functions can be called without creating an instance of the class, which can lead to performance improvements in certain scenarios. For example, if you have utility functions that are frequently called, using static functions can save memory and processing time.
Moreover, static functions can be particularly useful in scenarios where you need to maintain global state or configuration settings across different instances of a class. For instance, you can use static member variables to store configuration settings that should be shared among all instances, while static functions can provide methods to modify or retrieve those settings.
Here’s a practical example demonstrating the benefits of static functions:
#include <iostream>
#include <string>
using namespace std;
class Logger {
public:
static void log(const string& message) {
cout << "Log: " << message << endl;
}
};
int main() {
Logger::log("Application started");
Logger::log("Error occurred");
Logger::log("Application ended");
return 0;
}
Output:
Log: Application started
Log: Error occurred
Log: Application ended
In this example, the Logger
class provides a static log
function that can be called directly to log messages. This approach centralizes logging functionality, making it easier to manage and maintain.
Common Use Cases for Static Member Functions
Static member functions are commonly used in various scenarios in C++. One popular use case is for factory methods. These methods can create instances of a class without requiring an existing object. This is particularly useful in design patterns like Singleton or Factory patterns, where you may want to control the instantiation process.
Another common application is in mathematical or utility classes, where functions perform operations that do not depend on object state. For example, a class that provides mathematical constants or calculations can benefit from static methods.
Here’s an example of a factory method using static functions:
#include <iostream>
#include <memory>
using namespace std;
class Product {
public:
static unique_ptr<Product> create() {
return make_unique<Product>();
}
void display() {
cout << "Product created!" << endl;
}
};
int main() {
auto product = Product::create();
product->display();
return 0;
}
Output:
Product created!
In this case, the Product
class has a static create
method that returns a unique pointer to a new instance of Product
. This encapsulates the instantiation logic and allows for more controlled object creation.
Conclusion
Static member functions in C++ are powerful tools that enhance the functionality and organization of your code. By allowing you to define functions that can be called without an instance of the class, they provide flexibility and efficiency. Whether you’re implementing utility functions, factory methods, or managing global state, understanding how to effectively use static functions can significantly improve your programming skills. As you continue to explore C++, keep static functions in mind as a valuable addition to your toolkit.
FAQ
-
What is a static member function in C++?
A static member function is a function that belongs to the class itself rather than any object instance. It can be called using the class name. -
Can static member functions access non-static members?
No, static member functions cannot access non-static members directly since they do not operate on a specific instance of the class. -
What are some common use cases for static member functions?
Common use cases include utility functions, factory methods, and managing global state or configuration settings. -
How do you declare a static member function?
You declare a static member function by using thestatic
keyword before the function’s return type in the class definition. -
Can static member functions be overloaded?
Yes, static member functions can be overloaded just like regular member functions, as long as they have different parameter lists.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook