Difference Between int and size_t in C++
- What is int?
- What is size_t?
- Key Differences Between int and size_t
- When to Use int and size_t
- Conclusion
- FAQ
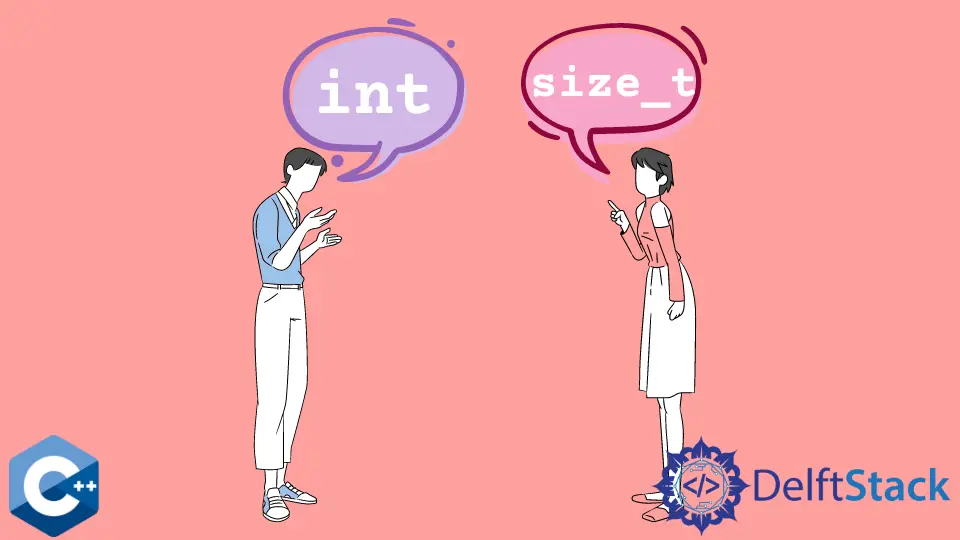
In the world of C++, understanding data types is crucial for writing efficient and error-free code. Among the various data types available, int
and size_t
often come up in discussions about memory allocation, loops, and array indexing. While they may seem interchangeable at a glance, they serve distinct purposes and have unique characteristics. In this guide, we will dive deep into the differences between int
and size_t
, exploring their definitions, use cases, and potential pitfalls. Whether you’re a beginner or an experienced programmer, grasping these differences will enhance your coding skills and improve your understanding of C++.
What is int?
The int
data type is one of the most commonly used integer types in C++. It represents a signed integer, which means it can hold both positive and negative values. The size of an int
can vary depending on the system architecture, but it typically occupies 4 bytes (32 bits) on most modern platforms. This allows it to represent a range of values from -2,147,483,648 to 2,147,483,647.
Here’s a simple example of using int
in a C++ program:
#include <iostream>
int main() {
int num = -10;
std::cout << "The value of num is: " << num << std::endl;
return 0;
}
Output:
The value of num is: -10
In this code snippet, we declare an integer variable named num
, assign it a negative value, and print it out. The use of int
is straightforward and effective for scenarios where negative numbers are required. However, it’s essential to be cautious about overflow when performing arithmetic operations, especially with large values.
What is size_t?
On the other hand, size_t
is an unsigned integer type specifically designed to represent the size of objects in memory. It is defined in the <cstddef>
header and is typically used in scenarios that involve memory allocation, array indexing, and loop counters. The key characteristic of size_t
is that it can only hold non-negative values, making it ideal for representing sizes, lengths, and counts.
Here’s an example of using size_t
in a C++ program:
#include <iostream>
#include <cstddef>
int main() {
size_t size = 10;
std::cout << "The size is: " << size << std::endl;
return 0;
}
Output:
The size is: 10
In this code, we declare a variable size
of type size_t
and assign it a positive value. The use of size_t
ensures that we won’t accidentally assign a negative value, which could lead to unexpected behavior when dealing with memory sizes or array lengths. This makes size_t
a safer choice in many programming scenarios.
Key Differences Between int and size_t
Now that we have a basic understanding of both int
and size_t
, let’s outline the key differences between them:
-
Signed vs. Unsigned: The most significant difference is that
int
is a signed type, allowing it to represent both negative and positive values, whilesize_t
is unsigned, meaning it can only represent non-negative values. -
Use Cases:
int
is generally used for arithmetic calculations and situations where negative values are possible. On the other hand,size_t
is specifically designed for representing sizes and counts, making it the preferred choice for array indices and memory allocation. -
Size: The size of
int
can vary across different platforms, but it usually occupies 4 bytes. In contrast,size_t
is defined as the maximum size that an object can have, and its size may vary depending on the architecture (32-bit or 64-bit). -
Overflow Behavior: When an
int
value exceeds its maximum limit, it wraps around to the minimum limit (overflow). In contrast, if asize_t
value exceeds its maximum limit, it can lead to undefined behavior since it cannot represent negative numbers.
When to Use int and size_t
Choosing between int
and size_t
often depends on the context of your code. If you are dealing with counts, sizes, or array indices, size_t
is the safer option. For instance, when iterating over an array, using size_t
can help avoid negative indices, which can lead to runtime errors.
Here’s an example of using size_t
in a loop:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << "Element at index " << i << " is: " << numbers[i] << std::endl;
}
return 0;
}
Output:
Element at index 0 is: 1
Element at index 1 is: 2
Element at index 2 is: 3
Element at index 3 is: 4
Element at index 4 is: 5
In this example, we use size_t
for the loop counter i
, ensuring that we safely iterate over the indices of the numbers
vector. Since numbers.size()
returns a size_t
, using the same type for i
prevents any potential issues related to type mismatch.
Conversely, when performing mathematical calculations where negative values might be involved, int
should be your go-to type. For example:
#include <iostream>
int main() {
int a = 5;
int b = -3;
int result = a + b;
std::cout << "The result is: " << result << std::endl;
return 0;
}
Output:
The result is: 2
In this case, using int
allows us to perform arithmetic operations that include both positive and negative values without any issues.
Conclusion
In summary, understanding the difference between int
and size_t
is crucial for writing robust C++ code. While int
is versatile and can represent both positive and negative integers, size_t
is specifically designed for non-negative sizes and counts, making it a safer choice in contexts like memory allocation and array indexing. By choosing the appropriate data type based on your specific needs, you can avoid common pitfalls and write more efficient code. As you continue your journey in C++, remember to consider the characteristics of different data types to enhance your programming skills.
FAQ
-
What is the main difference between int and size_t?
The main difference is thatint
is a signed type that can represent both negative and positive values, whilesize_t
is an unsigned type that can only represent non-negative values. -
When should I use size_t instead of int?
Usesize_t
when dealing with sizes, counts, or array indices, as it prevents negative values and is specifically designed for these scenarios. -
Can size_t represent negative numbers?
No,size_t
cannot represent negative numbers as it is an unsigned type. -
What happens if I assign a negative value to a size_t variable?
Assigning a negative value to asize_t
variable can lead to unexpected behavior, as it cannot hold negative values. -
Is the size of size_t the same on all platforms?
No, the size ofsize_t
can vary depending on the architecture (32-bit or 64-bit), whileint
also varies but typically occupies 4 bytes on most systems.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn