How to Round Floating-Point Number to 2 Decimals in C++
-
Use the
printf
Function Format Specifiers to Round Floating-Point Number to 2 Decimals in C++ -
Use
fprintf
Function Format Specifiers to Round Floating-Point Number to 2 Decimals in C++ -
Use
std::setprecision
andstd::fixed
to Round Floating-Point Number to 2 Decimals in C++
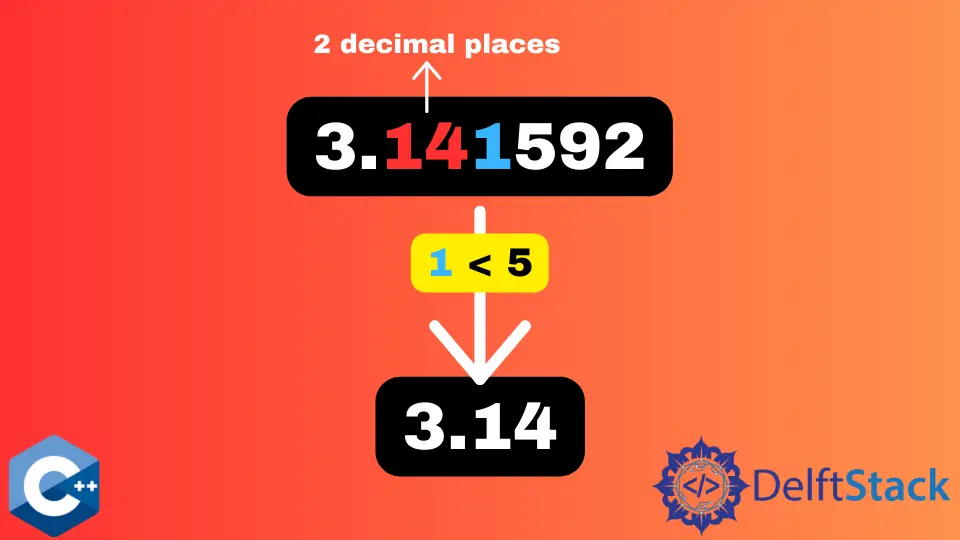
This article will explain several methods of how to round floating-point numbers to 2 decimals in C++.
Use the printf
Function Format Specifiers to Round Floating-Point Number to 2 Decimals in C++
Floating-point numbers have special binary representation, which entails that real numbers can’t be represented exactly by the machines. Thus, it’s common to resort to rounding functions when operating on the floating-point numbers and doing calculations on them. In this case, though, we only need to output a certain portion of the numbers’ fractional part.
The first solution is to utilize the printf
function to output formatted text to the stdout
stream. Note that the regular format specifier for the float
numbers - %f
should be modified to %.2f
. The latter notation ensures that only 2 decimals are printed from the number, and at the same time, it does the rounding according to the common mathematical rules. Mind that, a similar notation can be used with format specifiers of other data types.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
printf("%.2f; ", item);
}
return EXIT_SUCCESS;
}
Output:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
Use fprintf
Function Format Specifiers to Round Floating-Point Number to 2 Decimals in C++
fprintf
is another function that can be used to do the output formatting like the printf
call and additionally, it can write to any FILE*
stream object that can be passed as the first argument. In the following example, we demonstrate printing to the stderr
stream, an unbuffered version of stdout
for error reporting and logging.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
fprintf(stderr, "%.2f; ", item);
}
return EXIT_SUCCESS;
}
Output:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
Use std::setprecision
and std::fixed
to Round Floating-Point Number to 2 Decimals in C++
Alternatively, one can utilize the std::setprecision
function from the I/O manipulators’ library in conjunction with std::fixed
. The latter is used to modify the default formatting for floating-point input/output operations. If we use it together with std::setprecision
, the result is fixed precision of the real numbers, and the precision can be specified with the integer argument passed to the std::setprecision
itself.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
cout << setprecision(2) << fixed << item << "; ";
}
return EXIT_SUCCESS;
}
Output:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook