How to Reverse Array in C++
- Method 1: Using a Simple Loop
- Method 2: Using the Standard Library
- Method 3: Using Recursion
-
Use
rbegin
/rend
Iterators to Reverse Array in C++ - Conclusion
- FAQ
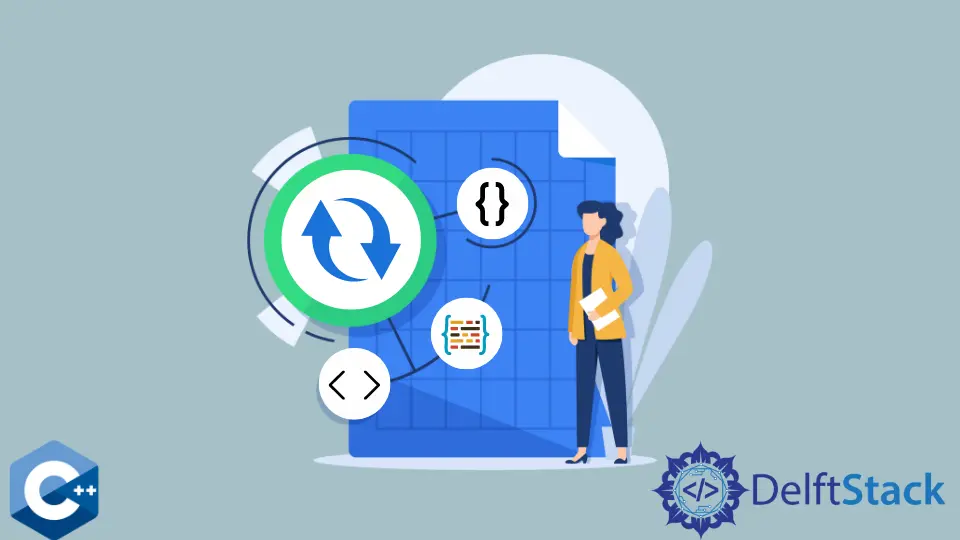
Reversing an array is a fundamental task in programming that can come in handy in various scenarios, from algorithm challenges to real-world applications. In C++, understanding how to reverse an array can enhance your coding skills and improve your problem-solving abilities.
This article will guide you through different methods to reverse an array in C++, complete with clear examples and explanations. Whether you are a beginner or an experienced programmer looking to brush up on your skills, this guide will provide you with the knowledge you need to effectively reverse arrays in C++. Let’s dive in and explore the various techniques!
Method 1: Using a Simple Loop
One of the most straightforward methods to reverse an array in C++ is by using a simple loop. This approach involves swapping elements from the start and end of the array until you reach the midpoint. It’s efficient and easy to understand, making it a great choice for beginners.
Here’s how you can implement this method:
#include <iostream>
using namespace std;
void reverseArray(int arr[], int n) {
for (int i = 0; i < n / 2; i++) {
swap(arr[i], arr[n - i - 1]);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output:
5 4 3 2 1
In this code, we define a function reverseArray
that takes an array and its size as parameters. The loop runs from the start of the array to its midpoint. During each iteration, we swap the element at the current index with the corresponding element from the end of the array. This continues until all elements have been swapped. The result is a reversed array, which we then print in the main
function. This method is efficient with a time complexity of O(n) and a space complexity of O(1).
Method 2: Using the Standard Library
C++ provides a powerful Standard Template Library (STL) that includes a function specifically designed to reverse arrays. Utilizing the std::reverse
function from the <algorithm>
header can simplify your code significantly. This method is not only concise but also takes advantage of the efficiency of the STL.
Here’s how to use std::reverse
:
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverse(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output:
5 4 3 2 1
In this example, we include the <algorithm>
header to access the std::reverse
function. We call reverse
with two parameters: the beginning and the end of the array. This function handles the swapping internally, making the code cleaner and easier to read. The result is the same as the previous method, but with less code to manage. This approach is particularly useful when you want to reverse arrays or vectors quickly without writing the logic from scratch.
Method 3: Using Recursion
If you’re looking for a more advanced technique, reversing an array using recursion can be a fascinating approach. This method involves calling the function recursively, swapping elements until the base case is reached. While not as efficient as the previous methods, it’s an excellent way to understand recursion better.
Here’s how to implement this recursive approach:
#include <iostream>
using namespace std;
void reverseArray(int arr[], int start, int end) {
if (start >= end) return;
swap(arr[start], arr[end]);
reverseArray(arr, start + 1, end - 1);
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
reverseArray(arr, 0, n - 1);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Output:
5 4 3 2 1
In this code snippet, we define a recursive function reverseArray
that takes the array and two indices: start
and end
. The base case checks if the start
index is greater than or equal to the end
index. If so, we return from the function. Otherwise, we swap the elements at the start
and end
indices and call the function recursively with the updated indices. This method is elegant and demonstrates how recursion can be used to solve problems, though it may not be the most efficient for large arrays due to the overhead of recursive calls.
Use rbegin
/rend
Iterators to Reverse Array in C++
In contrast with the first example of this topic, there are use cases when the reordered contents of the vector
need not be stored in the program flow, rather just outputted to console or display. The following example demonstrates how to print the array elements to console in reverse order and without modifying underlying variable contents.
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::ostream_iterator;
using std::vector;
void PrintVector(vector<int> &arr) {
copy(arr.begin(), arr.end(), ostream_iterator<int>(cout, "; "));
cout << endl;
}
int main() {
vector<int> arr1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
copy(arr1.rbegin(), arr1.rend(), ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Output:
10; 9; 8; 7; 6; 5; 4; 3; 2; 1;
This code demonstrates how to print a vector’s elements in reverse order without modifying the original vector. It uses the std::copy
algorithm along with reverse iterators and an ostream_iterator
. The copy
function is called with arr1.rbegin()
and arr1.rend()
as its first two arguments, which provide reverse iterators for the vector. The third argument is an ostream_iterator
that writes each element to cout
, followed by a semicolon and space.
This approach efficiently outputs the vector’s contents in reverse order without creating a new container or modifying the original data, showcasing a clean and memory-efficient way to display reversed data in C++.
Conclusion
Reversing an array in C++ can be achieved through various methods, each with its own advantages. Whether you choose to use a simple loop, the STL’s std::reverse
function, or a recursive approach, understanding these techniques will enhance your programming skills. As you practice these methods, you’ll find that reversing arrays can serve as a stepping stone to more complex data manipulation tasks. So, pick a method that suits your needs and start reversing arrays like a pro!
FAQ
-
What is the time complexity of reversing an array using a loop?
The time complexity is O(n), where n is the number of elements in the array. -
Can I reverse an array of characters using these methods?
Yes, all the methods discussed can be used to reverse arrays of any data type, including characters. -
Is the
std::reverse
function part of the C++ standard library?
Yes,std::reverse
is part of the C++ Standard Template Library (STL) and is included in the<algorithm>
header. -
What is the space complexity of the recursive method to reverse an array?
The space complexity is O(n) due to the call stack used for recursion. -
Which method is the most efficient for reversing large arrays?
The loop andstd::reverse
methods are generally more efficient for large arrays compared to the recursive method.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook