Concept of Returning Value, Reference to Value, and Const Reference in C++
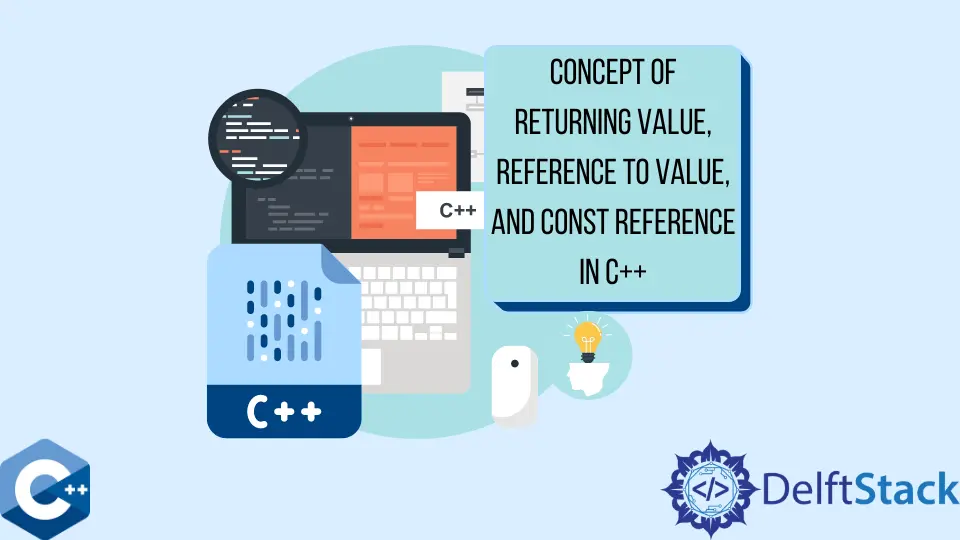
This article discusses returning a value, reference to value, and const
reference in C++.
Returning Value in C++
When you return something by value, you are returning a copy of the object. This imposes constraints on the class.
Accordingly, it may be costly to return an object by value for some types of objects. This also implies that the new object is independent of other objects and has value.
Many binary operators, such as +
, -
, and *
, should most likely return this value.
Reference to Value in C++
In C++, a reference is an object that holds the address of another object. This reference to value happens when a variable is declared with the type “reference to type”.
A reference-to-value can be converted to a value by using the &
operator.
Const
Reference to Value in C++
A const
variable is initialized with a value. This means that it can’t be changed later on.
A const
variable can be initialized with any type, including another const
variable or a constant expression. The initialization is usually done in the declaration, but it can also be done in the body of the function, as long as it’s before the first use of the variable.
In short, you cannot change an aliased object. When the object to be returned is expensive to copy, and you can guarantee its existence after returning from a function, you may use this technique instead of returning by value.
This is what operator=
typically returns to support multiple assignments in a way standard types do not.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook