How to Return 2D Array From Function in C++
- Use Pointer Notation to Return 2D Array From Function in C++
- Use Pointer to Pointer Notation to Return 2D Array From Function in C++
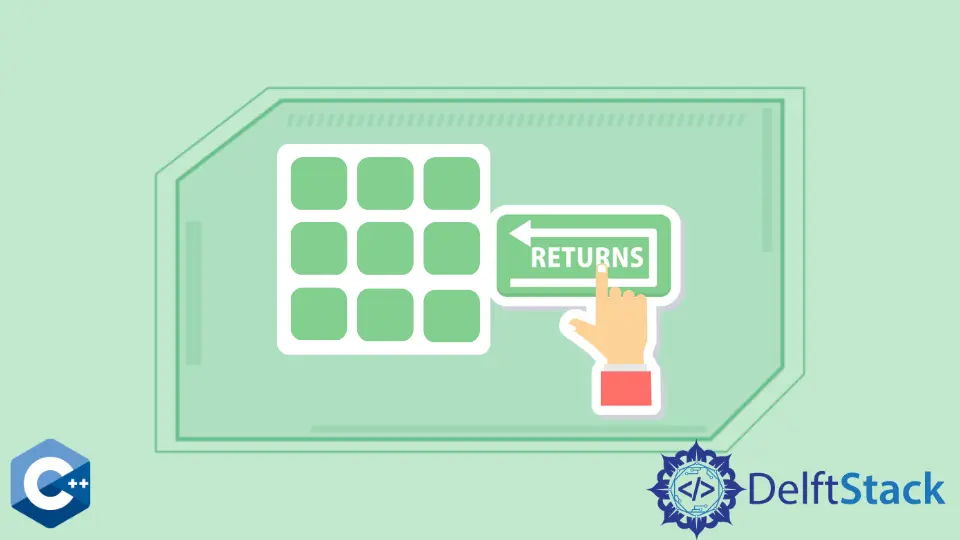
This article will introduce how to return a 2D array from a function in C++.
Use Pointer Notation to Return 2D Array From Function in C++
Return by the pointer is the preferred method for larger objects rather than returning them by value. Since the 2D array can get quite big, it’s best to pass the pointer
to the first element of the matrix, as demonstrated in the following code example. Note that the parameter for 2D array in ModifyArr
is defined with arr[][SIZE]
notation to access its elements with brackets in the function scope.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int SIZE = 4;
int *ModifyArr(int arr[][SIZE], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
return reinterpret_cast<int *>(arr);
}
int main() {
int c_array[SIZE][SIZE] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (auto &i : c_array) {
cout << " [ ";
for (int j : i) {
cout << setw(2) << j << ", ";
}
cout << "]" << endl;
}
cout << endl;
int *ptr = ModifyArr(c_array, SIZE);
cout << "modified array\n";
for (int i = 0; i < SIZE; ++i) {
cout << " [ ";
for (int j = 0; j < SIZE; ++j) {
cout << setw(2) << *(ptr + (i * SIZE) + j) << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
modified array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Use Pointer to Pointer Notation to Return 2D Array From Function in C++
As an alternative, we can use a pointer to pointer notation to return the array from the function. This method has an advantage over others if the objects to be returned are allocated dynamically. Usually, one should modify the element access expression once the pointer is returned in the caller scope. Notice that we cast the array address to int*
and then dereference to get the values.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int SIZE = 4;
int **ModifyArr2(int *arr, int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) *(arr + (i * len) + j) *= 2;
}
return reinterpret_cast<int **>(arr);
}
int main() {
int c_array[SIZE][SIZE] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (auto &i : c_array) {
cout << " [ ";
for (int j : i) {
cout << setw(2) << j << ", ";
}
cout << "]" << endl;
}
cout << endl;
int **ptr2 = ModifyArr2(c_array[0], SIZE);
cout << "modified array\n";
for (int i = 0; i < SIZE; ++i) {
cout << " [ ";
for (int j = 0; j < SIZE; ++j) {
cout << setw(2) << *((int *)ptr2 + (i * SIZE) + j) << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
modified array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook