How to Resize 2D Vector C++
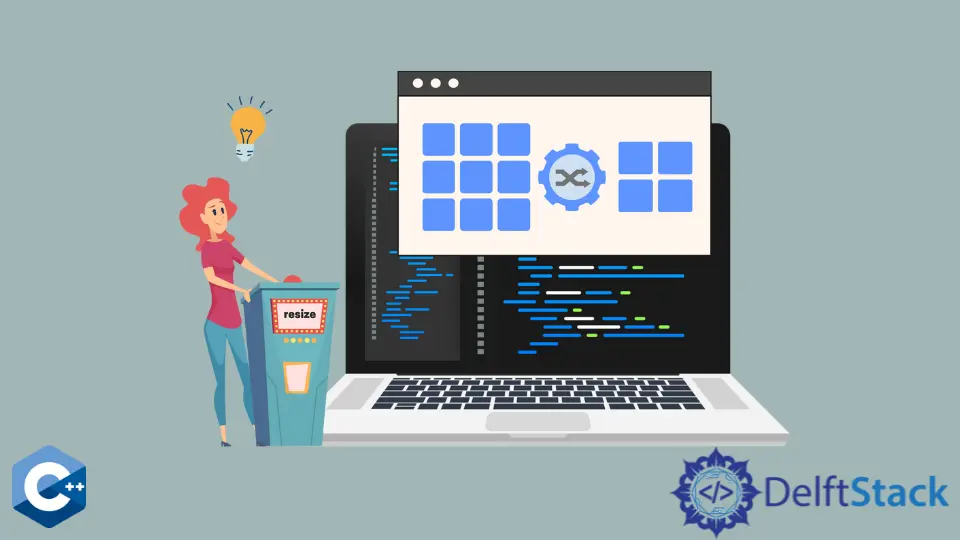
In C++, we can create a 2D vector using two one-dimensional vectors. The first one-dimensional vector specifies a point on a two-dimensional plane, while the second one-dimensional vector specifies an angle or rotation from this point.
In this short article, we’ll focus on how to resize the 2d vector in C++.
Resize 2D Vector in C++
To resize the 2d vector in C++, we need to use a function called resize()
, which is part of the STL library.
Syntax:
vector::resize()
The resize()
method takes two parameters, both of which are integers. The first integer specifies the new length of the vector, and the second integer specifies its new width.
To resize our vector, we need to call this function with a value for both parameters greater than or equal to 0. For example, if we want our 2d vector to be 10 pixels long and 5 pixels wide, we would call this function with a value of 10 for its length and 5 for its width.
1st Example Code:
#include <iostream>
#include <vector>
using namespace std;
#define X 1
#define Y 7
int main() {
int demo = 4;
vector<vector<int>> sam;
cout << "Vector contains:" << sam.size() << endl;
sam.resize(X, vector<int>(Y, demo));
cout << "Vector contains after resize:" << sam.size() << endl;
return 0;
}
Output:
Vector contains:0
Vector contains after resize:1
2nd Example Code:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> demovec;
// set vector content
for (int x = 2; x < 20; x++) demovec.push_back(x);
demovec.resize(2);
demovec.resize(9);
cout << "Vector contains:" << endl;
for (int x = 0; x < demovec.size(); x++) cout << ' ' << demovec[x];
return 0;
}
Output:
Vector contains:
2 3 0 0 0 0 0 0 0
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook