How to Remove Spaces From String in C++
-
Use
erase-remove
Idiom to Remove Spaces From String in C++ - Use Custom Function to Remove Spaces From String in C++
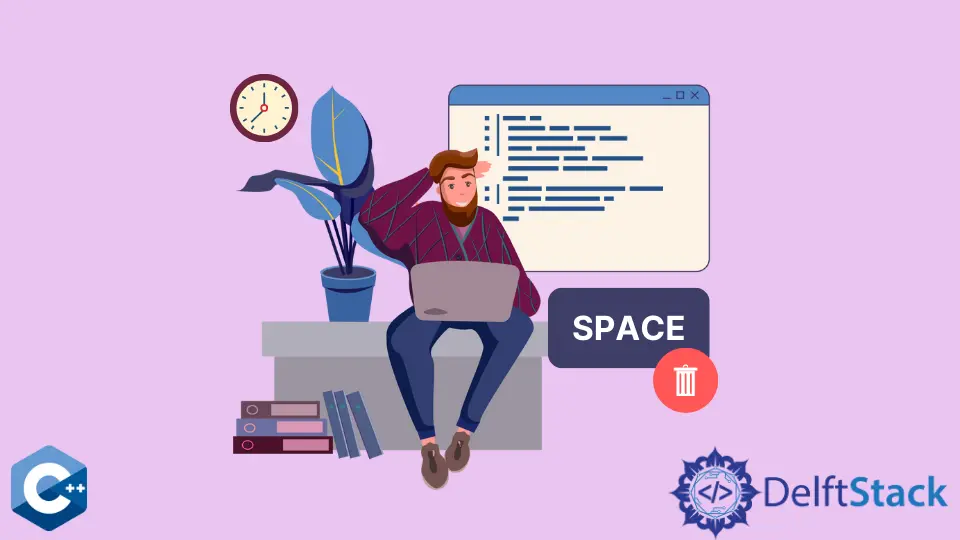
This article will demonstrate multiple methods about how to remove spaces from a string in C++.
Use erase-remove
Idiom to Remove Spaces From String in C++
One of the most useful methods for range manipulation in C++ is the erase-remove idiom which consists of two functions - std::erase
(built-in function for the most STL containers) and std::remove
(the part of the STL algorithms library). Note that both of them are chained to conduct the removal operation on the given object. std::remove
function takes two iterators to specify the range, and the third argument to denote the element’s value to be removed. In this case, we directly specify a space character, but any character can be specified to remove all of its occurrences in the string.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
str.erase(std::remove(str.begin(), str.end(), ' '), str.end());
cout << str << endl;
return EXIT_SUCCESS;
}
Output:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
On the other hand, the user also can pass unary predicate as the third argument to the std::remove
algorithm. The predicate should evaluate to bool
value for every element, and when the result is true
, the corresponding values are removed from the range. Thus, we can utilize a predefined isspace
function that checks for multiple whitespace characters like space - " "
, newline - \n
, horizontal tab - \t
, and several others.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
str.erase(std::remove_if(str.begin(), str.end(), isspace), str.end());
cout << str << endl;
return EXIT_SUCCESS;
}
Output:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
Use Custom Function to Remove Spaces From String in C++
Notice that all previous solutions modified the original string object, but sometimes, one may need to create a new string with all spaces removed. We can implement a custom function using the same erase-remove
idiom, that takes the string reference and returns a parsed value to be stored in a separate string object. This method could also be modified to support another function parameter that will specify the character that needs to be removed.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
string removeSpaces(const string& s) {
string tmp(s);
tmp.erase(std::remove(tmp.begin(), tmp.end(), ' '), tmp.end());
return tmp;
}
int main() {
string str = " Arbitrary str ing with lots of spaces to be removed .";
cout << str << endl;
string newstr = removeSpaces(str);
cout << newstr << endl;
return EXIT_SUCCESS;
}
Output:
Arbitrary str ing with lots of spaces to be removed .
Arbitrarystringwithlotsofspacestoberemoved.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++