How to Use the STL Priority Queue in C++
- Understanding the STL Priority Queue
- Basic Operations with STL Priority Queue
- Custom Comparison in STL Priority Queue
- Priority Queue with Objects
- Conclusion
- FAQ
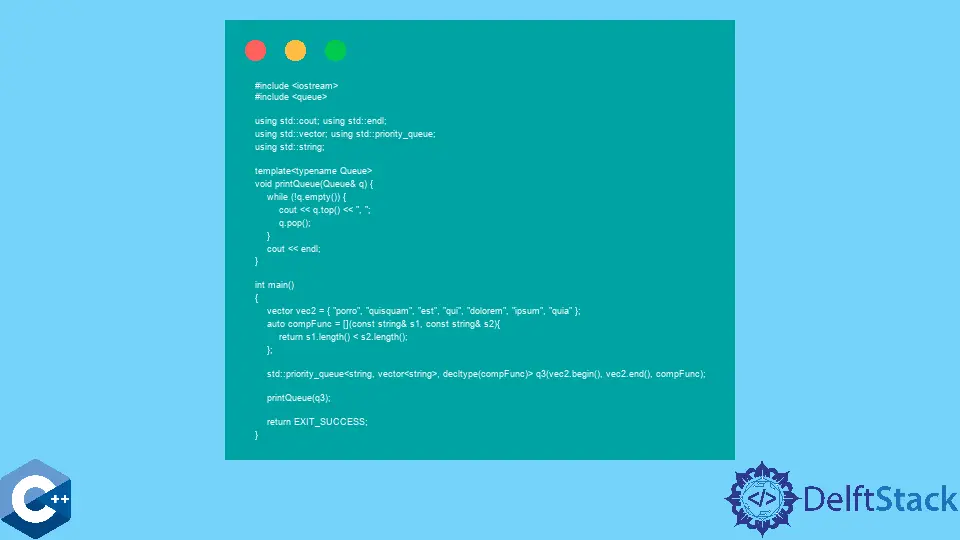
When it comes to managing data efficiently, the Standard Template Library (STL) in C++ offers a robust collection of data structures. One of the most powerful among these is the priority queue. A priority queue allows you to store elements in a way that the highest (or lowest) priority elements are always accessible first. This makes it incredibly useful for algorithms like Dijkstra’s shortest path, Huffman coding, and many more.
In this article, we will explore how to effectively use the STL priority queue in C++, including its features, methods, and practical code examples. Whether you are a beginner or looking to refine your skills, this guide will help you understand the ins and outs of priority queues in C++.
Understanding the STL Priority Queue
Before diving into code, it’s essential to understand what a priority queue is and how it operates in C++. The STL priority queue is a container adapter that provides constant time access to the largest element. It is implemented as a max-heap by default, meaning that the highest value is always at the top. You can also configure it as a min-heap if you prefer to prioritize lower values.
The priority queue supports several key operations:
Push
: Insert an element into the queue.Pop
: Remove the highest priority element.Top
: Access the highest priority element without removing it.Empty
: Check if the queue is empty.Size
: Get the number of elements in the queue.
With this foundational understanding, let’s look at how to implement and manipulate priority queues in C++.
Basic Operations with STL Priority Queue
Let’s start with a simple example of how to create a priority queue, add elements to it, and retrieve the highest priority element.
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> pq;
pq.push(10);
pq.push(20);
pq.push(15);
std::cout << "The highest priority element is: " << pq.top() << std::endl;
pq.pop();
std::cout << "After popping, the highest priority element is: " << pq.top() << std::endl;
return 0;
}
Output:
The highest priority element is: 20
After popping, the highest priority element is: 15
In this example, we first include the necessary header file for the priority queue. We then create a priority queue of integers. Using the push
method, we add three integers: 10, 20, and 15. The top
method retrieves the highest priority element, which is 20, without removing it from the queue. After calling pop
, we remove the top element, and the next highest priority element, 15, becomes accessible.
Custom Comparison in STL Priority Queue
One of the powerful features of the STL priority queue is the ability to define custom comparison functions. This allows you to change the way elements are prioritized. For instance, if you want a min-heap instead of a max-heap, you can use a custom comparator.
#include <iostream>
#include <queue>
#include <vector>
struct Compare {
bool operator()(int a, int b) {
return a > b; // For min-heap
}
};
int main() {
std::priority_queue<int, std::vector<int>, Compare> minHeap;
minHeap.push(10);
minHeap.push(20);
minHeap.push(15);
std::cout << "The lowest priority element is: " << minHeap.top() << std::endl;
minHeap.pop();
std::cout << "After popping, the lowest priority element is: " << minHeap.top() << std::endl;
return 0;
}
Output:
The lowest priority element is: 10
After popping, the lowest priority element is: 15
In this code, we define a custom comparator called Compare
. This struct overrides the operator()
to establish a min-heap by returning true
when the first element is greater than the second. When we create our priority queue, we specify this comparator as a template argument. As a result, the lowest priority element, 10, is retrieved first.
Priority Queue with Objects
Priority queues can also be used to manage more complex data types, such as objects. For example, let’s consider a scenario where we want to prioritize tasks based on their urgency.
#include <iostream>
#include <queue>
#include <string>
struct Task {
std::string name;
int priority;
bool operator<(const Task& other) const {
return priority < other.priority; // Max-heap based on priority
}
};
int main() {
std::priority_queue<Task> taskQueue;
taskQueue.push({"Task 1", 2});
taskQueue.push({"Task 2", 1});
taskQueue.push({"Task 3", 3});
while (!taskQueue.empty()) {
std::cout << "Processing: " << taskQueue.top().name << std::endl;
taskQueue.pop();
}
return 0;
}
Output:
Processing: Task 3
Processing: Task 1
Processing: Task 2
In this example, we define a Task
struct containing a name and a priority. By overloading the <
operator, we establish how tasks are compared based on their priority. When we create the priority queue, tasks are processed in order of their priority, with Task 3 being the highest priority.
Conclusion
The STL priority queue in C++ is a versatile and powerful data structure that can help you manage data efficiently. By understanding its basic operations, customizing comparisons, and even working with complex data types, you can leverage this tool to solve various programming challenges. Whether you’re optimizing algorithms or managing tasks, the priority queue is an essential part of your C++ toolkit. With practice, you’ll find it an invaluable resource in your programming endeavors.
FAQ
-
What is a priority queue in C++?
A priority queue is a data structure that allows you to store elements such that the highest (or lowest) priority element is always accessible first. -
How do I create a priority queue in C++?
You can create a priority queue by including the<queue>
header and using thestd::priority_queue
class. -
Can I use custom types in a priority queue?
Yes, you can store custom types in a priority queue by defining how they should be compared, typically by overloading the<
operator. -
What is the default behavior of the STL priority queue?
The default behavior of the STL priority queue is to create a max-heap, meaning the largest element is prioritized. -
How do I convert a max-heap to a min-heap?
You can convert a max-heap to a min-heap by using a custom comparator that defines the desired ordering.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook