How to Access Member Functions From Pointer to a Vector in C++
- Understanding Vectors and Pointers in C++
- Accessing Member Functions Using Pointer to a Vector
- Using Iterators with Pointers to Vectors
- Accessing Member Functions in a Loop
- Conclusion
- FAQ
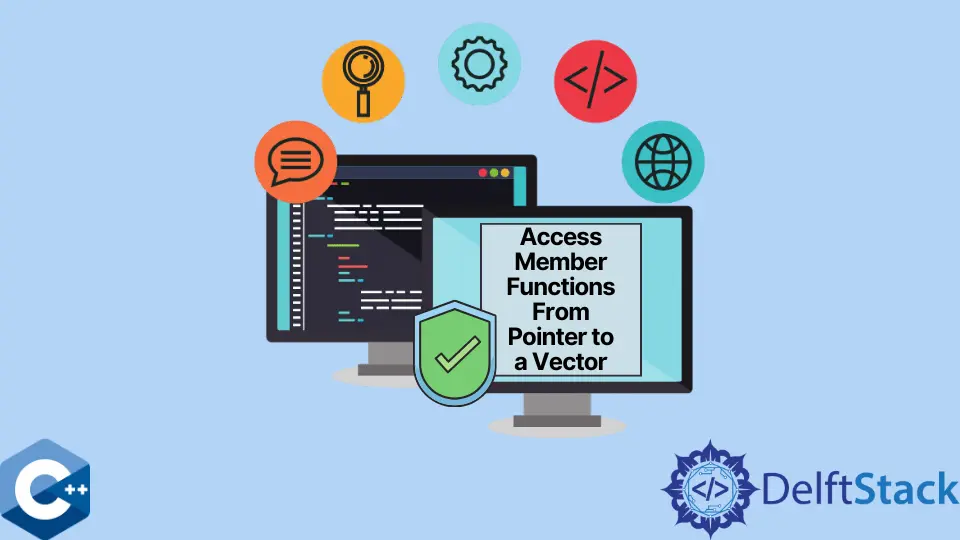
Navigating the intricacies of C++ can be both rewarding and challenging, especially when it comes to understanding pointers and vectors. One common task developers encounter is accessing member functions through pointers to vectors.
This article will guide you through the process of accessing member functions from a pointer to a vector in C++. We’ll explore various methods and provide clear code examples to solidify your understanding. Whether you’re a beginner looking to enhance your skills or an experienced programmer brushing up on your knowledge, this guide will equip you with the know-how to tackle this topic confidently.
Understanding Vectors and Pointers in C++
Before we dive into the methods, it’s essential to understand what vectors and pointers are in C++. A vector is a dynamic array that can grow in size, making it a versatile choice for storing collections of data. Pointers, on the other hand, are variables that store memory addresses, allowing you to manipulate data stored in different locations. When you have a pointer to a vector, you can access its elements and member functions, which is crucial for effective programming in C++.
Accessing Member Functions Using Pointer to a Vector
To access member functions from a pointer to a vector, you need to understand the syntax involved. The typical way to do this is by dereferencing the pointer and then calling the member function. Here’s a simple example to illustrate this concept.
#include <iostream>
#include <vector>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
std::vector<MyClass> myVector(1);
MyClass* ptr = &myVector[0];
ptr->display();
return 0;
}
Output:
Hello from MyClass!
In this example, we define a class MyClass
with a member function display
. We create a vector of MyClass
objects and obtain a pointer to the first element of the vector. By dereferencing the pointer with ptr->display()
, we can access the member function and execute it. This straightforward approach is effective for accessing member functions in C++.
Using Iterators with Pointers to Vectors
Another method to access member functions involves using iterators. Iterators are objects that allow you to traverse through the elements of a container like a vector. They provide a more flexible way to access elements, especially when you want to iterate through a vector using a pointer. Here’s how you can implement this.
#include <iostream>
#include <vector>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
std::vector<MyClass> myVector(1);
std::vector<MyClass>::iterator it = myVector.begin();
MyClass* ptr = &(*it);
ptr->display();
return 0;
}
Output:
Hello from MyClass!
In this example, we utilize an iterator to point to the first element of the vector. By dereferencing the iterator with *it
, we obtain a pointer to the MyClass
object. This method is particularly useful in scenarios where you need to navigate through the vector dynamically, allowing for more complex operations.
Accessing Member Functions in a Loop
When dealing with larger vectors, you may want to access member functions in a loop. This method is efficient for performing operations on multiple elements within your vector. Here’s a practical example of how to implement this.
#include <iostream>
#include <vector>
class MyClass {
public:
void display(int id) {
std::cout << "Hello from MyClass instance " << id << "!" << std::endl;
}
};
int main() {
std::vector<MyClass> myVector(5);
for (size_t i = 0; i < myVector.size(); ++i) {
MyClass* ptr = &myVector[i];
ptr->display(i);
}
return 0;
}
Output:
Hello from MyClass instance 0!
Hello from MyClass instance 1!
Hello from MyClass instance 2!
Hello from MyClass instance 3!
Hello from MyClass instance 4!
In this example, we create a vector of five MyClass
objects. We then iterate through the vector using a loop. In each iteration, we obtain a pointer to the current MyClass
object and call its display
method, passing the index as an argument. This approach allows you to access and manipulate multiple objects within the vector seamlessly.
Conclusion
Accessing member functions from a pointer to a vector in C++ is a fundamental skill that enhances your programming toolkit. Whether you use direct pointers, iterators, or loops, understanding these methods will empower you to write more efficient and effective code. By mastering these techniques, you can confidently handle complex data structures and improve your overall programming proficiency. Keep practicing, and you’ll find that working with pointers and vectors becomes second nature.
FAQ
-
What is a vector in C++?
A vector is a dynamic array that can change size during runtime, allowing for flexible data storage. -
How do I create a pointer to a vector?
You can create a pointer to a vector by using the&
operator on a vector object, likestd::vector<Type>* ptr = &myVector;
. -
Can I access private member functions using pointers?
Yes, you can access private member functions using pointers, provided you have the appropriate access rights in the context of your code. -
What are iterators in C++?
Iterators are objects that allow you to traverse through the elements of a container, such as a vector, in a consistent manner. -
How do I use pointers with vectors efficiently?
You can use pointers with vectors efficiently by utilizing iterators and loops, which allow for dynamic access and manipulation of vector elements.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Function
- C++ Redefinition of Formal Parameter
- The ShellExecute() Function in C++
- The const Keyword in Function Declaration of Classes in C++
- How to Handle Arguments Using getopt in C++
- Time(NULL) Function in C++
- Cotangent Function in C++
Related Article - C++ Pointer
- Dangling Pointers in C++
- Declaration and Uses of unique_ptr in C++
- Function Pointer to Member Function in C++
- How to Swap Two Numbers Using Pointers in C++
- Void Pointer in C++
- Functionality and Difference Between *& and **& in C++