POD Type in C++
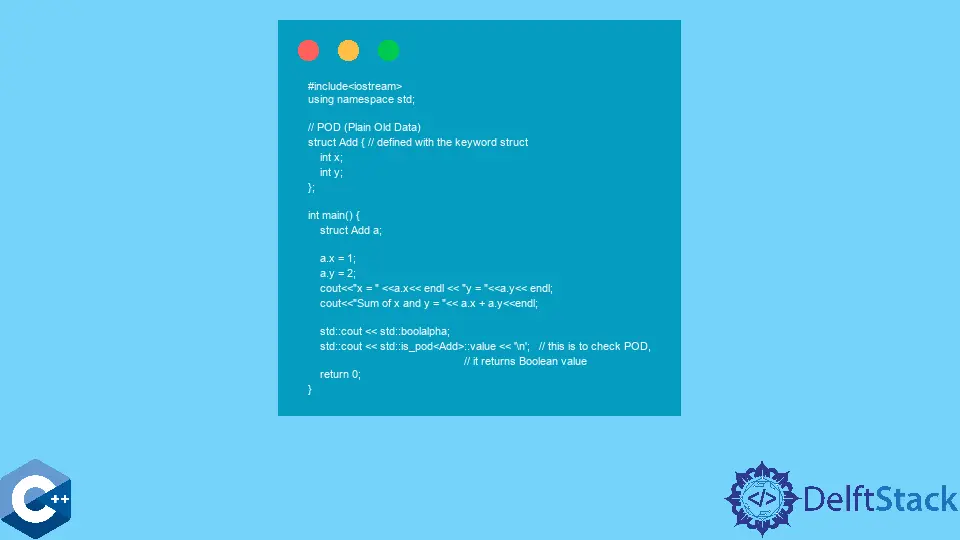
PODs in C++ stands for Plain Old Data. It is a class defined with the keywords struct
or class
and only has data members such as int, char, double, bool, signed/unsigned, long/short, float, etc.
POD Type in C++
As we know, PODs are generally built-in data types like classes and structures that you can define with the keyword class
or struct
, but it is not like other classes or structures. PODs in C++ do not support constructors, destructors, virtual functions, etc.
PODs (Plain Old Data) in C++ is an aggregate class or structure that contains only PODs as data members. It does not define user-defined copy assignment operators or other non-static members of the pointer-to-member type.
Example code:
#include <iostream>
using namespace std;
// POD (Plain Old Data)
struct Add { // defined with the keyword struct
int x;
int y;
};
int main() {
struct Add a;
a.x = 1;
a.y = 2;
cout << "x = " << a.x << endl << "y = " << a.y << endl;
cout << "Sum of x and y = " << a.x + a.y << endl;
std::cout << std::boolalpha;
std::cout << std::is_pod<Add>::value << '\n'; // this is to check POD,
// it returns Boolean value
return 0;
}
Output:
x = 1
y = 2
Sum of x and y = 3
true
This code has a class ADD
defined with the keyword struct
and has two data members - x
and y
. Values are passed to the ADD
class members through the class object in the main function.
These values are further added and displayed as output in the cout
statement. Now, the class is ready, and values are passed.
It is time to check whether it is a POD or not; the function is_pod
is here to help us. It returns True
if the class is a POD and returns False
otherwise.
Furthermore, pointers, including pointer-to-member and pointer-to-function, are PODs. Enums
, const
, and volatile
are also PODs.
A struct
, class
, or union
of PODs is also a POD, provided that all non-static data members are specified as public
and have no virtual functions, constructors, or destructors, and base class.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn