How to Parse Int From String in C++
-
Use the
std::stoi
Function to Parse Int From String -
Use the
std::from_chars
Function to Parse Int From String
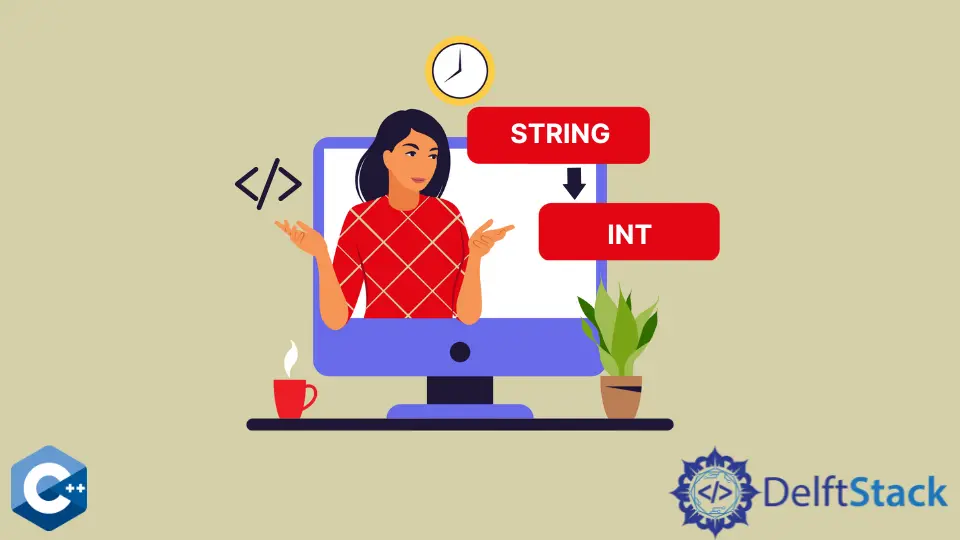
This article will explain several methods of how to parse an int
from a string in C++.
Use the std::stoi
Function to Parse Int From String
The stoi
function is part of the string library defined in the string
header, and it can be utilized to convert string values to different numeric types. std::stoi
, std::stol
and std::stoll
are used for signed integer conversion. The stoi
function takes a single string
object as a mandatory argument, but the programmer may also specify the address to store the integer and the number base in which the input string has to be processed.
The following example demonstrates multiple use cases of the stoi
function. Note that stoi
can handle leading white spaces in the string, but any other character will cause a std::invalid_argument
exception.
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
int main() {
string s1 = "333";
string s2 = "-333";
string s3 = "333xio";
string s4 = "01011101";
string s5 = " 333";
string s6 = "0x33";
int num1, num2, num3, num4, num5, num6;
num1 = stoi(s1);
num2 = stoi(s2);
num3 = stoi(s3);
num4 = stoi(s4, nullptr, 2);
num5 = stoi(s5);
num6 = stoi(s6, nullptr, 16);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
return EXIT_SUCCESS;
}
Output:
num1: 333 | num2: -333
num3: 333 | num4: 93
num5: 333 | num6: 51
Use the std::from_chars
Function to Parse Int From String
As an alternative, the from_chars
function from the utilities library can parse int
values. It’s been part of the standard library since the C++17 version and is defined in the <charconv>
header file.
Much different from the stoi
, from_chars
operates on the ranges of characters, not being aware of the object length and borders. Thus, the programmer should specify the start and end of the range as the first two arguments. The third argument is the int
variable to which the converted value will be assigned.
Mind though, from_chars
can only handle the leading -
sign in the input string; hence it prints garbage values of num5
and num6
in the following example.
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::from_chars;
using std::stoi;
using std::string;
int main() {
string s1 = "333";
string s2 = "-333";
string s3 = "333xio";
string s4 = "01011101";
string s5 = " 333";
string s6 = "0x33";
int num1, num2, num3, num4, num5, num6;
from_chars(s1.c_str(), s1.c_str() + s1.length(), num1);
from_chars(s2.c_str(), s2.c_str() + s2.length(), num2);
from_chars(s3.c_str(), s3.c_str() + s3.length(), num3);
from_chars(s4.c_str(), s4.c_str() + s4.length(), num4, 2);
from_chars(s5.c_str(), s5.c_str() + s5.length(), num5);
from_chars(s6.c_str(), s6.c_str() + s6.length(), num6);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
return EXIT_SUCCESS;
}
Output:
num1: 333 | num2: -333
num3: 333 | num4: 93
num5: -1858679306 | num6: 0
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook