How to Overload Input and Output Stream Insertion Operators in C++
- Uses of Input and Output Stream Insertion Operators
- Steps to Overload Input and Output Stream Insertion Operators in C++
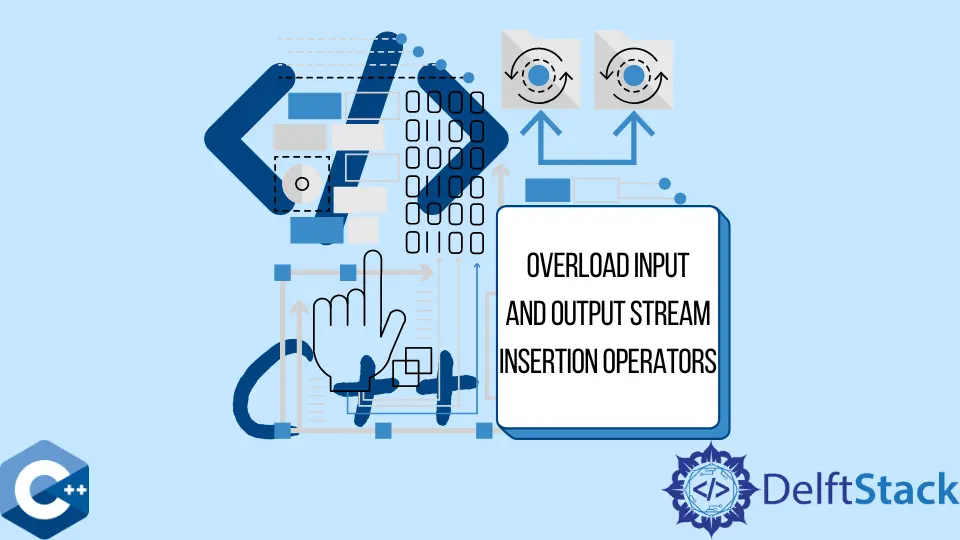
You can perform operator overloading in C++. This article is about the input and output stream insertion operators.
Operators are a way to create expressions in C++. You can overload them, which means you can define new meanings for the operators.
The input and output stream insertion operators read or write information from a file. In C++, the stream insertion operator <<
is used for output, while >>
is used for input.
Before we begin overloading these operators, we must first understand these points. cin
and cout
are objects of the istream
and ostream
classes.
As a global function, these operators must be overloaded. And we need to add them as friends if we want to give them access to the class members’ private information.
It is important to note that in the case of operator overloading, the object on the operator’s left side must contain the operator if it is overloaded as a member.
Uses of Input and Output Stream Insertion Operators
The following are the uses of inputs and outputs stream insertion operators:
- To insert input data in a program.
- To insert output data in a program.
- To create a pipe that can be used to pass data from one process to another.
Steps to Overload Input and Output Stream Insertion Operators in C++
The following steps can be followed to overload inputs and outputs stream insertion operators in C++:
-
Create a class with two public data members: Input and Output stream.
-
Create two public functions, namely, output
operator<<()
and inputoperator>>()
. -
In both functions, create a loop that iterates through each character of the input string using
getline()
and insert them into the output string usingputchar()
. -
In both functions, use
return 0;
to terminate the program.
Let’s discuss an example.
#include <iostream>
using namespace std;
class Demo {
private:
int x;
int y;
public:
Demo() {
x = 0;
y = 0;
}
Demo(int x1, int y1) {
x = x1;
y = y1;
}
friend ostream &operator<<(ostream &output, const Demo &S) {
output << "x1 : " << S.x << " y1 : " << S.y;
return output;
}
};
int main() {
Demo S1(6, 2), S2(3, 19);
cout << "1st Value : " << S1 << endl;
cout << "2nd Value : " << S2 << endl;
return 0;
}
Output:
1st Value : x1 : 6 y1 : 2
2nd Value : x1 : 3 y1 : 19
Click here to check the working of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook