How to Overload the Bracket Operator in C++
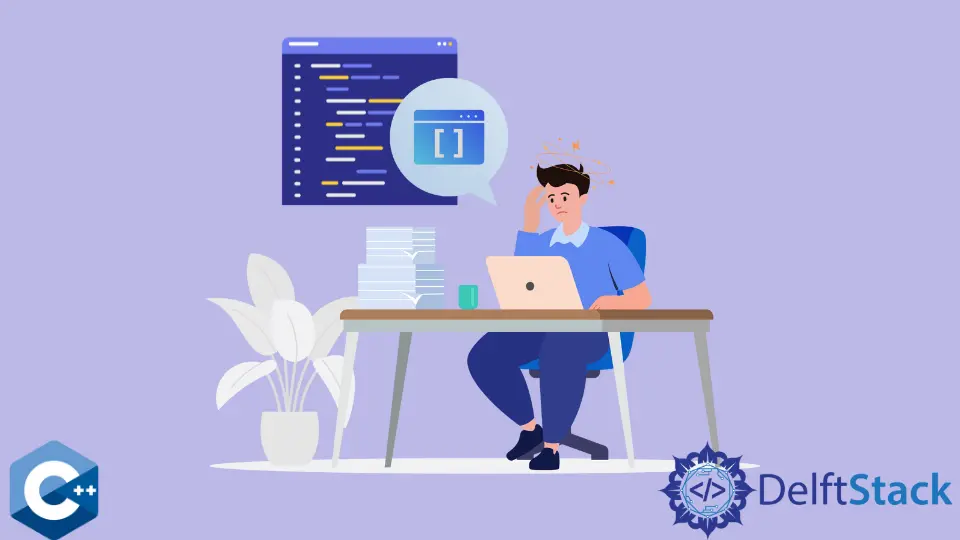
This trivial programming guide overloads the bracket ([]
) operator to be a getter and setter for the class objects.
First, let’s have a brief introduction to operator overloading.
Operator Overloading in C++
The idea of modifying how operators behave while working with user-defined datatypes like classes and structs is known as operator overloading. These are similar to member functions in a class called when that operator is used.
For instance, assume we want to determine which employee in a class of Employees
has a higher salary than the others. The >
operator may be overloaded to compare the salary
data member of the two objects and return the outcome.
The syntax for the operator overloading is as follows:
class ClassIdentifier {
[AccessSpecifier] : ReturnType operator OperatorSymbol([list of arguments]) {
// definition
}
};
The optional access specifier should preferably be public
. If you make it private
, then the overloading function will only be able to get accessed from within the class.
This is usually undesirable in most cases.
Note that operator
is a keyword used for operator overloading; after that, we specify the symbol of the operator that needs to be overloaded. Remember that we cannot overload operators for fundamental data types like int, char, float, etc.
Overload the Bracket []
Operator in C++
We can also overload the []
bracket operator so that it can be used to get and set the value in a class object.
For example, we have the MyClass
class with an array as a data member. To access the elements of that array using the object’s name, we can overload the []
bracket operator like this:
class MyClass {
private:
int arr[5];
public:
int operator[](int i) const { return arr[i]; } // for getting value
int& operator[](int i) { return arr[i]; } // for setting value
};
Note that we have returned the reference to the given index for setting the value so the user can change it. You can use it in the driver program like this:
int main() {
MyClass obj;
obj[0] = 100; // setting values
obj[1] = 200; // setting values
cout << obj[0] << endl; // getting values
cout << obj[1] << endl; // getting values
return 0;
}
In the above main()
function, when the obj[0] = 100;
is executed, it calls the second overloaded function with 0
as an argument to i
. The overloaded setter method returns an integer reference to the memory space of the 0th index of arr
.
The value at the right side of the assignment operator (=
) is now assigned at the returned location. Therefore, the 0th index of the data member of obj
will be given a value of 100
.
Let’s combine the above code pieces into one complete executable program.
#include <iostream>
using namespace std;
class MyClass {
private:
int arr[5];
public:
int operator[](int i) const { return arr[i]; } // for getting value
int& operator[](int i) { return arr[i]; } // for setting value
};
int main() {
MyClass obj;
obj[0] = 100; // setting values
obj[1] = 200; // setting values
cout << obj[0] << endl; // getting values
cout << obj[1] << endl; // getting values
return 0;
}
Output:
100
200