How to Overload the Addition Operator in C++
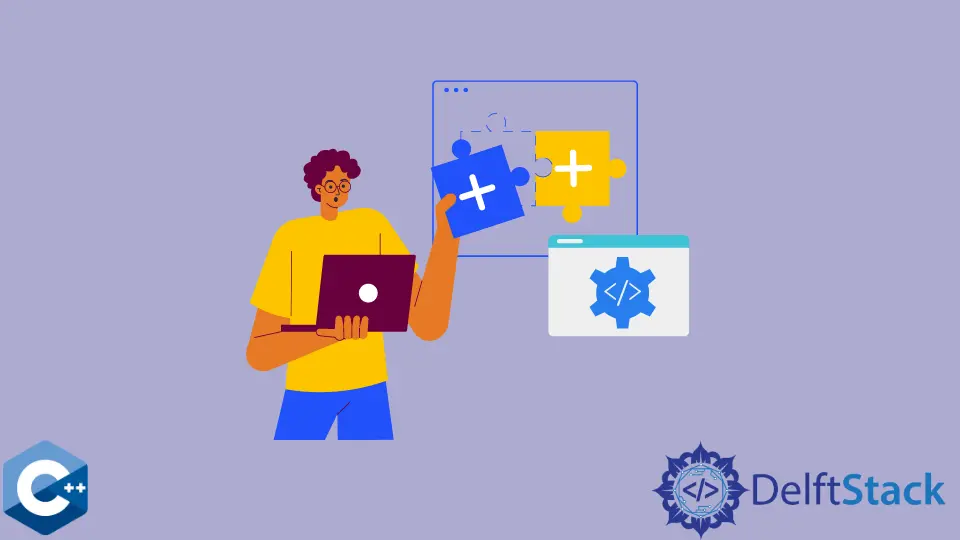
Operator overloading is a technique in which a programmer can redefine the meaning of an operator. It is a mechanism that allows programmers to extend the syntax of their programming language by adding new meanings to symbols and keywords.
Operators can be classified as unary, binary, or ternary. Unary operators take one operand, binary operators take two operands, and ternary operators take three operands.
You can overload operators using a friend
function, a member function, or a normal function. This article will only focus on overloading the addition operator using the friend
function in C++.
The friend
keyword is used in the C++ language to specify which classes can access private data members of a class. It is important to note that a friend
function is not a class member but is given permission to access some or all of that class’s private data and functions.
Friend classes are often used to provide helper functions for other classes. The friend
functions are sometimes called non-member functions since they are not members of the class.
In C++, if you want to overload an operator using the friend
function, you need to declare it as a friend
. The friend
declaration tells the compiler that this function will be used with other functions and objects of the same class.
Overload the Addition Operator Using the friend
Function in C++
In C++, the overloaded addition operator is a binary operator that takes two operands of the same type and performs addition on them.
The following steps are used to overload the addition operator in C++ using the friend
function:
-
Define a template class, which can call
operator+()
in the derived class. -
Define a
friend
function that overloads the addition operator in the derived class. -
Define the overloaded operators for addition in the template class.
-
Define an overloaded operator for the addition operation in the derived class called by the
friend
function defined in step 2.
Example code:
#include <iostream>
class Demo {
private:
int e_demo{};
public:
Demo(int demo) : e_demo{demo} {}
friend Demo operator+(const Demo& x1, const Demo& x2);
int getDemo() const { return e_demo; }
};
Demo operator+(const Demo& x1, const Demo& x2) {
return Demo{x1.e_demo + x2.e_demo};
}
int main() {
Demo demo1{5};
Demo demo2{5};
Demo demoSum{demo1 + demo2};
std::cout << "Total Count is " << demoSum.getDemo();
return 0;
}
Output:
Total Count is 10
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook