The or Operator in C++
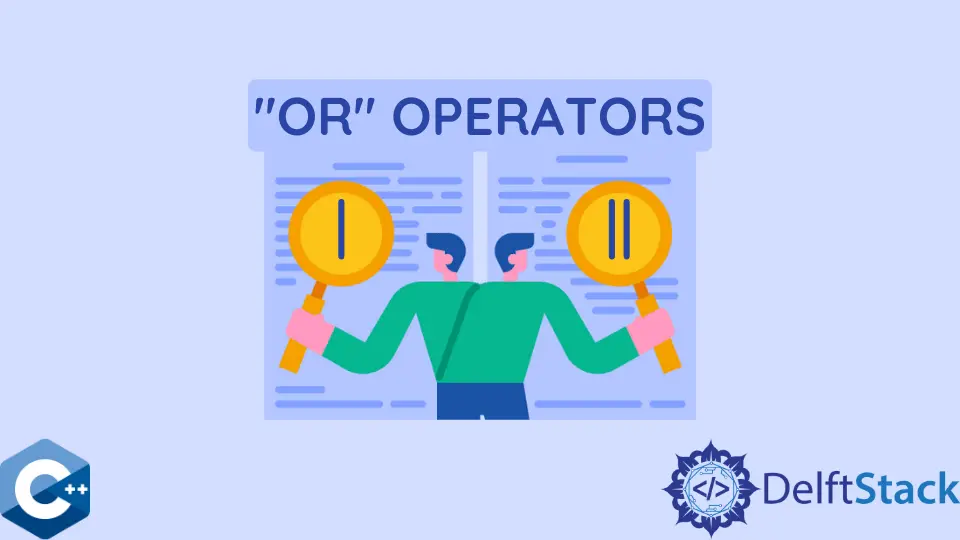
This article explains OR
operators in C++ and their use cases. Like any other programming language, C++ has logical OR
and bitwise OR
; let’s look at them one by one.
the Logical OR
Operator in C++
We use a logical operator to compare two or more operands/expressions and return true
or false
values. These are useful in decision-making scenarios.
Logical OR
is represented using ||
.
Syntax:
expression1 || expression2
The logical OR
operator ||
returns:
true
- if at least one operand or expression is true.false
- if and only if all the operands are false.
The truth table of logical OR
||
.
A
and B
are operands.
A | B | A||B |
---|---|---|
F | F | F |
F | T | T |
T | F | T |
T | T | T |
We can see only when both the operands are false
; we get the output as false
. In C or C++, false
is zero and true
is any non-zero value; generally, it is represented using integer 1
.
Example:
Let’s see how logical OR
is used in decision-making. Consider a situation where a user inputs a lowercase character and wants to know whether it’s a vowel
or a consonant
.
#include <iostream>
using namespace std;
int main() {
char ch;
cin >> ch;
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') {
cout << "It's is a VOWEL.";
} else
cout << "It's a consonant";
}
If the input is z
, all the OR
conditions inside the if
statement are false
, it is evaluated as false
, and the if
condition fails. Then the else
block is executed.
Output:
z
It's a consonant
One interesting property to remember about the logical OR
is that if the left-hand side part is true
, the right-hand side part is not evaluated because the whole expression is true now anyway.
Meaning in expression1||expression2
if expression1
is true
, expression2
is not evaluated.
Example code:
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = (a = a > 5 ? 25 : 0) || 0;
cout << "Value of a " << a << endl;
cout << "Value of b " << b << endl;
// part two
int x = 9;
int c = (x = 0) || (x = 6789);
cout << "Value of x " << x << endl;
cout << "Value of c " << c << endl;
}
Output:
Value of a 25
Value of b 1
Value of x 6789
Value of c 1
Explanation: (a=a>5?25:0)
is evaluated as true
because a
is greater than 5 and 25 is assigned to a
, and in C++ other than 0(false)
, all values are considered as true
.
Since the left-hand side part of logical OR
is true
right-hand side is not evaluated; the overall expression value is true
that is 1
and is assigned to b
.
Now part two of the program c = (x=0) || (x=6789)
, LHS x=0
means it’s false
, so RHS is evaluated x=6789
since any value other than zero is true
, the whole expression becomes true now, and 1
is assigned to c
.
the Bitwise OR
Operator in C++
A single vertical line |
represents the bitwise OR
. The main difference between logical and bitwise operators is that the result of logical operators (&&
, ||
, and !
) is either 0(false)
or 1(true)
, but the result of a bitwise operator is an integer value.
The |
(bitwise OR
) in C or C++ takes two numbers as operands and does OR
on every bit of two numbers and returns the result.
Example code:
#include <iostream>
using namespace std;
int main() {
int a = 2;
int b = 4;
int c = a || b; // logical OR
int d = a | b; // bitwise OR
cout << "Value of c " << c << endl;
cout << "value of d " << d << endl;
}
Output:
Value of c 1
value of d 6
Explanation: For a||b, a=2
, which is a non-zero value, is evaluated as true
, so the value of c
becomes true
.
For a|b
, we have to first convert a
and b
in binary format and then apply OR
on each bit, a = 010
and b = 100
in binary, and if we apply OR
on each bit, we get output as 6, which is assigned to the variable d
.
0 1 0 this is a=2
1 0 0 this is b=4
-------
1 1 0 //this is 6 in decimal
-------
Conclusion
We learned about logical and bitwise OR
operators in this article.
We understood that logical OR
is used for decision making, and any non-zero operands/expressions are considered 1(true)
, whereas in bitwise OR
, the operation is applied to each bit, and an integer value is returned as output.