NULL Undeclared Error in C++
- Solution 1: Include Necessary Headers
- Solution 2: Use nullptr Instead of NULL
- Solution 3: Check Project Configuration
- Conclusion
- FAQ
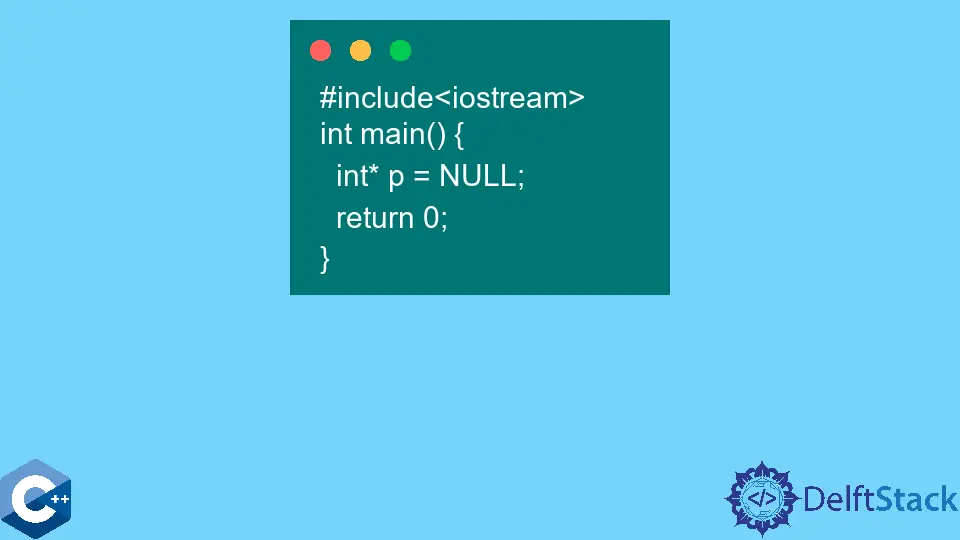
When working with C++, encountering a NULL undeclared error can be quite frustrating. This error typically occurs when the compiler cannot recognize the NULL identifier, often due to missing headers or improper configurations. Understanding how to troubleshoot and resolve this issue is essential for any C++ developer, whether you’re a beginner or an experienced programmer.
In this article, we will explore the causes of the NULL undeclared error and provide effective solutions to help you get back on track with your coding projects. Let’s dive in!
There are cases when you get the error when using the NULL
keyword like this:
int main() {
int* p = NULL;
return 0;
}
Output:
Now, let’s discuss how to solve this error.
The NULL undeclared error in C++ indicates that the compiler does not recognize the NULL keyword. This can happen for several reasons, including missing standard library headers or misconfigured project settings. In C++, NULL is often used as a null pointer constant, and its absence can lead to significant issues in your code.
To avoid this error, it’s crucial to ensure that your code is correctly set up and that you are using the appropriate libraries. In many cases, simply including the right headers can resolve the problem.
Solution 1: Include Necessary Headers
One of the simplest and most effective ways to resolve the NULL undeclared error is to ensure that you have included the necessary headers in your C++ code. The NULL identifier is defined in the header file <cstddef>
, which is part of the C++ standard library. By including this header, you can ensure that the NULL identifier is recognized by the compiler.
Here’s how you can include the necessary header:
#include <cstddef>
int main() {
int* ptr = NULL;
return 0;
}
Including the <cstddef>
header allows the compiler to recognize the NULL macro, preventing any undeclared errors. This is a straightforward solution that can often resolve the issue without requiring extensive code changes. Remember that good coding practices include always checking which headers are necessary for your project.
Solution 2: Use nullptr Instead of NULL
Another modern solution to the NULL undeclared error is to use nullptr
, which was introduced in C++11. The nullptr
keyword serves as a type-safe null pointer constant, making it a preferable alternative to NULL. By using nullptr
, you can avoid potential issues related to type ambiguity that can arise with NULL.
Here’s how to implement this change:
int main() {
int* ptr = nullptr;
return 0;
}
By switching to nullptr
, you not only eliminate the undeclared error but also make your code more robust and easier to understand. This change is particularly beneficial in large codebases where clarity and type safety are paramount. As C++ continues to evolve, adopting newer features like nullptr
can lead to cleaner and more efficient code.
Solution 3: Check Project Configuration
Sometimes, the NULL undeclared error can stem from issues in your project configuration. If your development environment is not set up correctly, it may not recognize standard library headers, leading to compilation errors. To resolve this issue, you should check your project settings and ensure that the correct paths to the C++ standard library are included.
Here’s a general approach to checking your project configuration:
- Open your project settings in your IDE.
- Navigate to the C++ compiler settings.
- Verify that the include paths for the standard library are correctly set.
- If necessary, add the path to the standard library headers.
By ensuring that your project is configured correctly, you can prevent the NULL undeclared error from occurring in the first place. This solution may require a bit more effort, but it is essential for maintaining a stable and functional development environment.
Conclusion
Encountering a NULL undeclared error in C++ can be a common hurdle for developers. However, by following the solutions outlined in this article—such as including necessary headers, using nullptr
, and checking your project configuration—you can effectively resolve this issue. Understanding these concepts not only helps in troubleshooting but also enhances your overall coding skills. With practice and attention to detail, you can prevent such errors from disrupting your workflow.
FAQ
-
What causes the NULL undeclared error in C++?
The NULL undeclared error usually occurs when the compiler cannot recognize the NULL identifier due to missing headers or misconfigured project settings. -
How can I include necessary headers in my C++ code?
You can include necessary headers by using the#include
directive at the beginning of your code, such as#include <cstddef>
. -
Why should I use nullptr instead of NULL?
Usingnullptr
provides type safety and helps avoid ambiguity, making your code clearer and more robust. -
How do I check my project configuration in an IDE?
You can check your project configuration by accessing the project settings in your IDE and verifying the include paths for the C++ standard library.
- Can the NULL undeclared error affect my entire project?
Yes, if not resolved, the NULL undeclared error can lead to compilation failures, affecting your entire project.