Multidimensional Vectors in C++
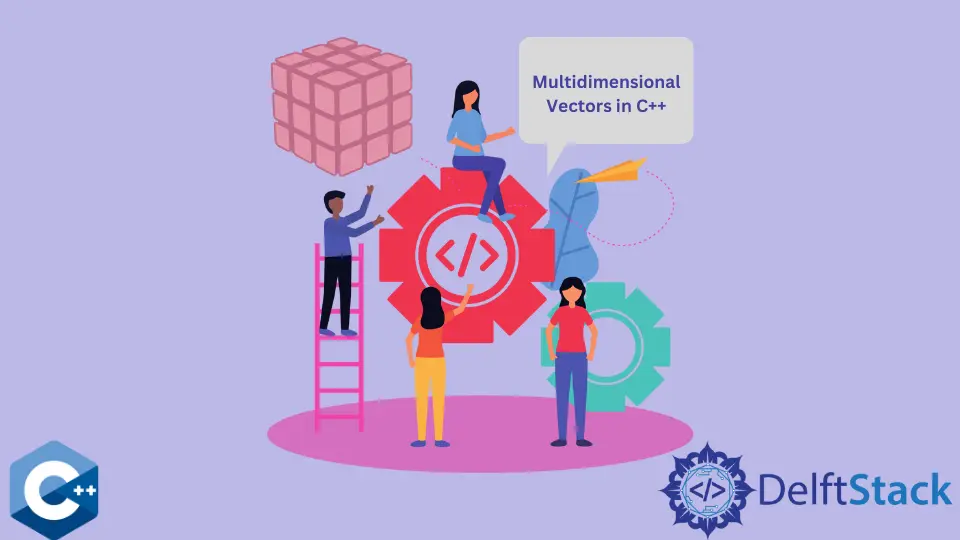
This brief programming tutorial is about the introduction to multidimensional vectors in C++. Vectors are containers that can store data like dynamic arrays in C++ with an automatic resizing feature.
Multidimensional Vectors in C++
An abstract data structure called a C++ multidimensional vector may be created using several C++ building components, but this tutorial will mainly look at the Standard Template Library std::vector
container implementation. We will use some methods in the std::vector
class to simplify operations on multidimensional vectors.
Declare and Initialize 2D Vectors in C++
By placing a vector specifier within the template parameter of another vector, you may specify a 2-dimensional vector.
Remember that only the inner vector is set for the element data type. It is possible to express matrices and carry out associated operations using 2-dimensional vectors.
The following code sample shows the definition and list initialization of 2-dimensional vectors.
We decided to define a 2-dimensional vector with integer members at random. The following sample code has three vectors with the labels mat1
, mat2
, and mat3
.
Not everyone has the same number of elements in each row, as you can see. Finally, we print the data in these vectors using a helper function called PrintMatrix
.
#include <iomanip>
#include <iostream>
#include <vector>
using namespace std;
void PrintMatrix(vector<vector<int>> vec) {
for (auto &item : vec) {
for (auto &elem : item) cout << elem << " ";
cout << endl;
}
cout << endl;
}
int main() {
vector<vector<int>> mat1 = {{0, 1, 2}, {3, 4, 5}, {6, 7}};
vector<vector<int>> mat2 = {{10, 9}, {8, 7, 6, 5}, {4, 3, 2}};
vector<vector<int>> mat3 = {{11, 12}, {13}, {14, 15, 16, 17, 18, 19}};
PrintMatrix(mat1);
PrintMatrix(mat2);
PrintMatrix(mat3);
return 0;
}
You can see in the above code snippet that the three vectors have different sizes for each row. These sizes are automatically adjusted according to the data initialized in them.
The code will give the following output:
Declare and Initialize 3D Vectors in C++
Like a 2D vector, the 3D vector is a vector of the vector of a vector
. It is like a 3-dimensional matrix.
The syntax will follow the same pattern as in a 2D vector, as shown in the example below:
vector<vector<vector<int>>> threeDVector = {{{11, 12}, {12, 13}, {13, 14, 15}},
{{11, 12}, {12, 13}, {13, 14}},
{{11, 12}, {12, 13}, {13, 14}}};
for (int a = 0; a < threeDVector.size(); a++) {
for (int b = 0; b < threeDVector[a].size(); b++) {
for (int c = 0; c < threeDVector[a][b].size(); c++) {
cout << threeDVector[a][b][c] << " ";
}
cout << endl;
}
cout << endl;
}
This will give the following output:
Thus, we can say that vectors act like arrays but are more efficient to operate and use in terms of memory and time.