How to Use the Modulo Operator in C++
-
Use the
%
Operator to Calculate Remainder in Division -
Use the
%
Operator to Generate Random Integers Less Than a Given Maximum Number in C++ -
Use Library-Defined Function Objects to Substitute the
%
Operator in C++
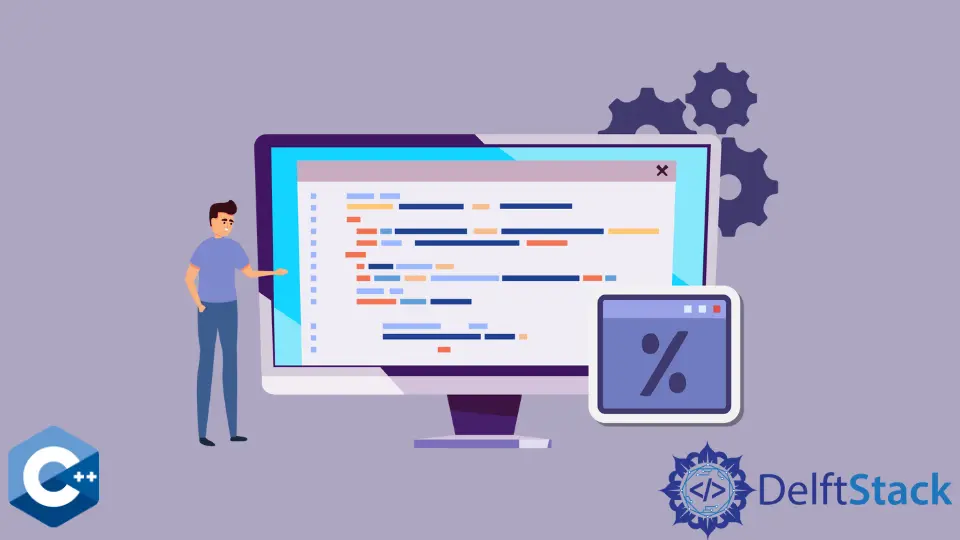
This article will introduce how to use the modulo operator in C++.
Use the %
Operator to Calculate Remainder in Division
The modulo (%
) operator’s standard feature is to compute the remainder of a division. The return value of the statement - x % y
represents the remainder left after x
is divided by y
. Modulo operator is defined so that both operands must be integers and a divisor is non-zero. The following example demonstrates the modulo operator used with different signed integer pairs. Note that, to print the character %
to the console using the printf
function, we should use %%
.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> ivec1{24, 24, -24, -24, 24};
vector<int> ivec2{7, 8, -8, -7, -6};
for (size_t i = 0; i < ivec1.size(); ++i) {
printf("% -d %% % -d = % -d\n", ivec1[i], ivec2[i], ivec1[i] % ivec2[i]);
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
24 % 7 = 3
24 % 8 = 0
-24 % -8 = 0
-24 % -7 = -3
24 % -6 = 0
Use the %
Operator to Generate Random Integers Less Than a Given Maximum Number in C++
Alternative usage of modulo operator is to control the random number generator to provide only numbers less than a specific integer. A programmer is responsible for choosing the random number generation toolkit depending on the need for quality of randomness, but both methods can be combined with %
to specify the upper limit of generated numbers. In this case, we use the rand
function, the return value of which is paired with the desired maximum value using the modulo operator.
#include <ctime>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
constexpr int MAX = 1000;
constexpr int NUMS_TO_GENERATE = 10;
int main() {
vector<int> ivec1{24, 24, -24, -24, 24};
vector<int> ivec2{7, 8, -8, -7, -6};
std::srand(std::time(nullptr));
for (int i = 0; i < NUMS_TO_GENERATE; i++) {
cout << rand() % MAX << "; ";
}
cout << endl;
cout << endl;
return EXIT_SUCCESS;
}
Output:
98; 194; 398; 190; 782; 550; 404; 557; 509; 945;
Use Library-Defined Function Objects to Substitute the %
Operator in C++
The C++ standard library defines multiple classes that represent traditional arithmetic, relational and logical operators. These are called function objects and have names as std::plus<Type>
, std::modulus<Type>
and etc. Type
specifies the parameter type of the call operator. The following code sample shows the std::modulus
function object utilized on vectors of integers.
#include <ctime>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> ivec1{24, 24, -24, -24, 24};
vector<int> ivec2{7, 8, -8, -7, -6};
std::modulus<> intMod;
int mod_of_nums = intMod(ivec1[1], ivec2[1]);
printf("%d %% %d = %d\n", ivec1[1], ivec2[1], mod_of_nums);
return EXIT_SUCCESS;
}
Output:
24 % 8 = 0
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook