Maze Solver in C++
- Understanding the Maze Structure
- Depth-First Search (DFS) Method
- Breadth-First Search (BFS) Method
- Conclusion
- FAQ
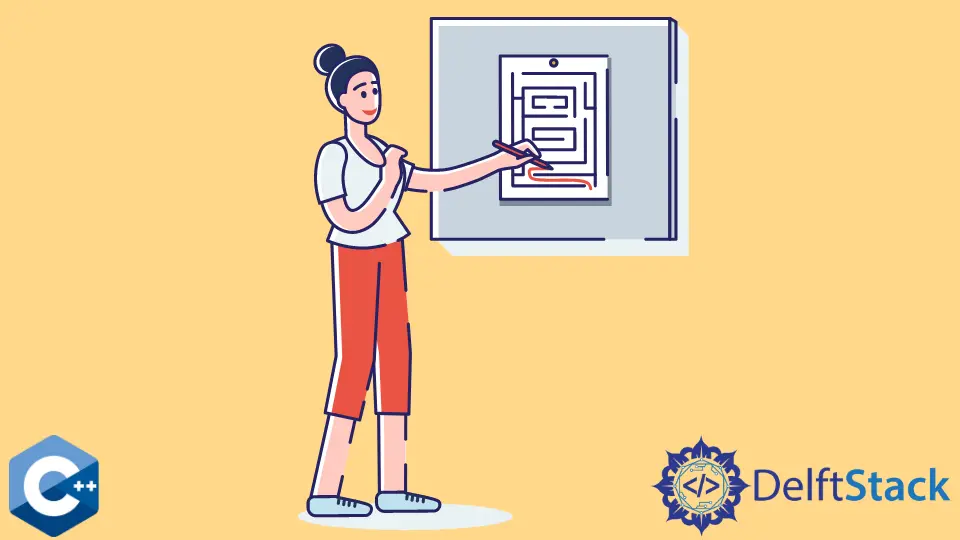
Navigating through a maze can be a daunting task, whether it’s a physical maze or a virtual one in programming.
In this article, we’ll explore how to implement a maze solver in C++ that efficiently finds the shortest path from the start to the finish using a maze-solving algorithm. By moving only in the four compass directions—up, down, left, and right—this approach ensures that we find the most optimal route. Whether you’re a beginner or an experienced coder, understanding this algorithm can enhance your problem-solving skills and deepen your knowledge of data structures. Let’s dive into the world of maze-solving algorithms and see how we can implement them in C++.
Understanding the Maze Structure
Before we jump into the code, it’s essential to understand how a maze is structured. Typically, a maze is represented as a 2D array, where:
- ‘0’ represents a path that can be traversed
- ‘1’ represents a wall or obstacle
For example, a simple maze might look like this:
0 1 0 0
0 1 0 1
0 0 0 1
1 1 0 0
In this representation, the goal is to find a way from the top-left corner (0,0) to the bottom-right corner (3,3). The maze solver will utilize a depth-first search (DFS) or breadth-first search (BFS) algorithm to explore possible paths.
Depth-First Search (DFS) Method
The DFS algorithm is a popular choice for maze-solving because it explores as far as possible along each branch before backtracking. Here’s how you can implement it in C++:
#include <iostream>
#include <vector>
using namespace std;
bool isSafe(int x, int y, vector<vector<int>>& maze, vector<vector<bool>>& visited) {
return (x >= 0 && x < maze.size() && y >= 0 && y < maze[0].size() && maze[x][y] == 0 && !visited[x][y]);
}
bool dfs(vector<vector<int>>& maze, int x, int y, vector<vector<bool>>& visited, vector<pair<int, int>>& path) {
if (x == maze.size() - 1 && y == maze[0].size() - 1) {
path.push_back({x, y});
return true;
}
visited[x][y] = true;
path.push_back({x, y});
int row[] = { -1, 1, 0, 0 };
int col[] = { 0, 0, -1, 1 };
for (int i = 0; i < 4; i++) {
int newX = x + row[i];
int newY = y + col[i];
if (isSafe(newX, newY, maze, visited) && dfs(maze, newX, newY, visited, path)) {
return true;
}
}
path.pop_back();
return false;
}
vector<pair<int, int>> solveMaze(vector<vector<int>>& maze) {
vector<vector<bool>> visited(maze.size(), vector<bool>(maze[0].size(), false));
vector<pair<int, int>> path;
if (dfs(maze, 0, 0, visited, path)) {
return path;
}
return {};
}
int main() {
vector<vector<int>> maze = {
{0, 1, 0, 0},
{0, 1, 0, 1},
{0, 0, 0, 1},
{1, 1, 0, 0}
};
vector<pair<int, int>> solution = solveMaze(maze);
for (auto& p : solution) {
cout << "(" << p.first << ", " << p.second << ") ";
}
return 0;
}
Output:
(0, 0) (1, 0) (2, 0) (2, 1) (2, 2) (3, 2) (3, 3)
The DFS method starts at the top-left corner of the maze and recursively explores each possible direction. The isSafe
function checks whether the next move is valid. If it reaches the goal (bottom-right corner), it returns the path taken. If a dead end is encountered, the function backtracks, removing the last position from the path. This process continues until the maze is completely explored or the path is found.
Breadth-First Search (BFS) Method
Another effective way to solve mazes is through the BFS algorithm, which explores all neighbors at the present depth prior to moving on to nodes at the next depth level. This approach is particularly useful for finding the shortest path in an unweighted maze.
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
struct Node {
int x, y;
vector<pair<int, int>> path;
};
vector<pair<int, int>> bfsMazeSolver(vector<vector<int>>& maze) {
vector<vector<bool>> visited(maze.size(), vector<bool>(maze[0].size(), false));
queue<Node> q;
q.push({0, 0, {{0, 0}}});
visited[0][0] = true;
int row[] = { -1, 1, 0, 0 };
int col[] = { 0, 0, -1, 1 };
while (!q.empty()) {
Node current = q.front();
q.pop();
if (current.x == maze.size() - 1 && current.y == maze[0].size() - 1) {
return current.path;
}
for (int i = 0; i < 4; i++) {
int newX = current.x + row[i];
int newY = current.y + col[i];
if (newX >= 0 && newX < maze.size() && newY >= 0 && newY < maze[0].size() &&
maze[newX][newY] == 0 && !visited[newX][newY]) {
visited[newX][newY] = true;
vector<pair<int, int>> newPath = current.path;
newPath.push_back({newX, newY});
q.push({newX, newY, newPath});
}
}
}
return {};
}
int main() {
vector<vector<int>> maze = {
{0, 1, 0, 0},
{0, 1, 0, 1},
{0, 0, 0, 1},
{1, 1, 0, 0}
};
vector<pair<int, int>> solution = bfsMazeSolver(maze);
for (auto& p : solution) {
cout << "(" << p.first << ", " << p.second << ") ";
}
return 0;
}
Output:
(0, 0) (1, 0) (2, 0) (2, 1) (2, 2) (3, 2) (3, 3)
In the BFS method, we utilize a queue to explore the maze level by level. Starting from the initial position, we check all four possible directions. If a valid move is found, we mark it as visited and push it onto the queue. Each node in the queue retains its path, allowing us to return the shortest path once we reach the destination. This method guarantees that the first time we reach the end of the maze, we have found the shortest path.
Conclusion
Implementing a maze solver in C++ is a rewarding exercise that highlights the power of algorithms like DFS and BFS. By understanding how these algorithms work, you can tackle more complex problems in the future. Whether you’re solving puzzles, creating games, or working on robotics, mastering maze-solving techniques can significantly enhance your programming skills. As you continue to explore different algorithms, remember that practice is key. So, roll up your sleeves and start coding your own maze solver today!
FAQ
-
What is a maze-solving algorithm?
A maze-solving algorithm is a method used to find the shortest path from a starting point to an endpoint in a maze. -
Why use DFS or BFS for maze solving?
DFS is useful for exploring deep paths, while BFS guarantees the shortest path in an unweighted maze. -
Can I use other programming languages for maze solving?
Yes, maze-solving algorithms can be implemented in various programming languages, including Python, Java, and C#. -
How can I visualize the maze-solving process?
You can visualize the process by printing the maze at each step or using graphical libraries to create a visual representation. -
Are there more complex maze-solving algorithms?
Yes, there are advanced algorithms like A* and Dijkstra’s algorithm, which can be used for weighted mazes or more complex scenarios.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook