C++ Invalid Conversion of Int* to Int
- Understanding the Invalid Conversion Error
- Dereferencing the Pointer
- Using Static Cast for Conversion
- Conclusion
- FAQ
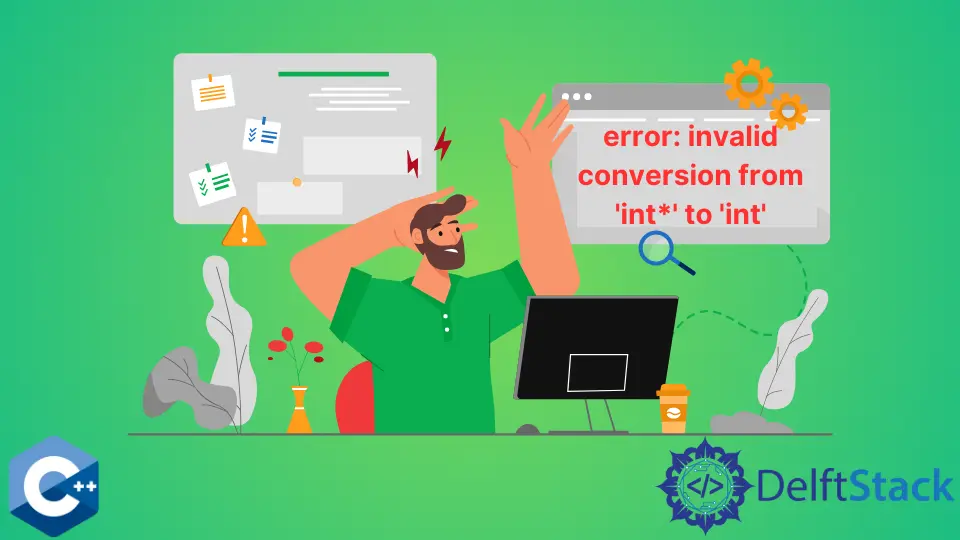
C++ is a powerful programming language that offers extensive control over system resources, but it comes with its own set of challenges. One frequently encountered issue is the “invalid conversion of int* to int” error. This error often arises when programmers mistakenly attempt to convert a pointer type into a non-pointer type, leading to confusion and frustration. If you’ve ever found yourself stuck on this error, you’re not alone. In this guide, we’ll explore what causes this error and how to troubleshoot it effectively. With clear explanations and practical examples, you’ll be well on your way to mastering this aspect of C++ programming.
Understanding the Invalid Conversion Error
The “invalid conversion of int* to int” error typically occurs when you try to assign an integer pointer to an integer variable. In C++, pointers are variables that store memory addresses, whereas integers store numerical values. Attempting to directly assign a pointer to an integer is not only incorrect but also leads to undefined behavior. Understanding the distinction between these two types is crucial for effective C++ programming.
For example, consider the following code snippet:
int* ptr = new int(5);
int num = ptr; // This line will cause the invalid conversion error
When you run this code, the compiler will throw an error indicating that it cannot convert from int*
to int
. The variable ptr
is a pointer that holds the address of an integer, while num
is just an integer.
Output:
invalid conversion from 'int*' to 'int'
To resolve this error, you need to dereference the pointer to access the value it points to. This process involves using the dereference operator *
.
Dereferencing the Pointer
One of the simplest ways to fix the invalid conversion error is by dereferencing the pointer. Dereferencing allows you to access the value stored at the memory address the pointer is pointing to. Here’s how you can do it:
int* ptr = new int(5);
int num = *ptr; // Correctly dereferencing the pointer
In this corrected code, the *ptr
expression dereferences the pointer, retrieving the integer value stored at that memory location. Now, num
correctly holds the integer value 5
.
Output:
num = 5
By dereferencing the pointer, you eliminate the type mismatch, allowing the assignment to proceed without errors. This method is fundamental in C++ programming and is essential for working with pointers effectively. It’s important to remember to free the allocated memory later in your code using delete ptr;
to prevent memory leaks.
Using Static Cast for Conversion
Another approach to resolve the invalid conversion error is by using static_cast
. This method is particularly useful when you want to convert between pointer types safely. Here’s how you can apply static_cast
to fix the error:
int* ptr = new int(5);
int num = static_cast<int>(*ptr); // Using static_cast for conversion
In this example, static_cast<int>(*ptr)
explicitly converts the dereferenced pointer back to an integer. This method is safer than a C-style cast because it provides compile-time type checking.
Output:
num = 5
Using static_cast
can make your code clearer and safer, especially when dealing with complex pointer conversions. It ensures that you are explicitly indicating your intention to convert types, which can help prevent errors in larger codebases.
Conclusion
The “invalid conversion of int* to int” error in C++ can be a stumbling block for many developers, but understanding pointers and how to work with them is key to overcoming this challenge. By employing techniques like dereferencing pointers or using static_cast
, you can resolve this common issue and write more robust C++ code. Remember, pointers are powerful tools, but they require careful handling to avoid pitfalls. With practice, you’ll become more comfortable navigating these challenges, allowing you to harness the full potential of C++ programming.
FAQ
-
what causes the invalid conversion of int* to int?
This error occurs when you try to assign a pointer type to a non-pointer type without dereferencing it. -
how can I fix the invalid conversion error?
You can fix this error by dereferencing the pointer or usingstatic_cast
to convert the pointer value to an integer. -
is it safe to use pointers in C++?
Yes, but they require careful management to avoid memory leaks and undefined behavior. -
what is the difference between a pointer and an integer in C++?
A pointer stores a memory address, while an integer stores a numerical value. -
what is the dereference operator in C++?
The dereference operator*
is used to access the value stored at the address a pointer is pointing to.